C# でストップウォッチを使用する
Muhammad Maisam Abbas
2024年2月16日
Csharp
Csharp Time
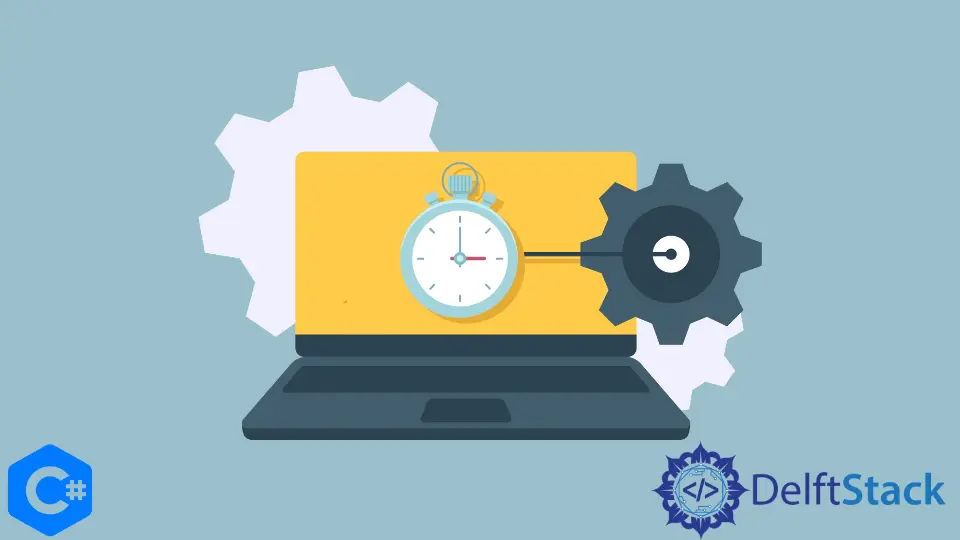
このチュートリアルでは、C# で経過時間を計算する方法について説明します。
C# の Stopwatch
クラスで経過時間を計算する
Stopwatch
クラスは、C# での経過時間を正確に測定します。Stopwatch.Start()
メソッドはストップウォッチを開始し、Stopwatch.Stop()
メソッドはストップウォッチを停止して経過時間を計算します。
次のコード例は、C# の Stopwatch
クラスで実行するコードの一部にかかる時間を計算する方法を示しています。
using System;
using System.Diagnostics;
namespace stopwatch {
class Program {
static void StopwatchUsingMethod() {
var timer = new Stopwatch();
timer.Start();
for (int i = 0; i < 1000000000; i++) {
int x = i * i + 1;
}
timer.Stop();
TimeSpan timeTaken = timer.Elapsed;
string foo = "Time taken: " + timeTaken.ToString(@"m\:ss\.fff");
Console.WriteLine(foo);
}
static void Main(string[] args) {
StopwatchUsingMethod();
}
}
}
出力:
Time taken: 0:03.226
上記のコードでは、Stopwatch
クラス timer
のインスタンスを作成し、for
ループの実行にかかる時間を計算しました。timer.Start()
メソッドを使用してストップウォッチを開始し、timer.Stop()
メソッドを使用してストップウォッチを停止しました。経過時間を timer.Elapsed
プロパティを使用して TimeSpan
クラスのインスタンスに格納し、C# の ToString()
関数を使用して文字列変数に変換しました。
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn