C# で円を描く
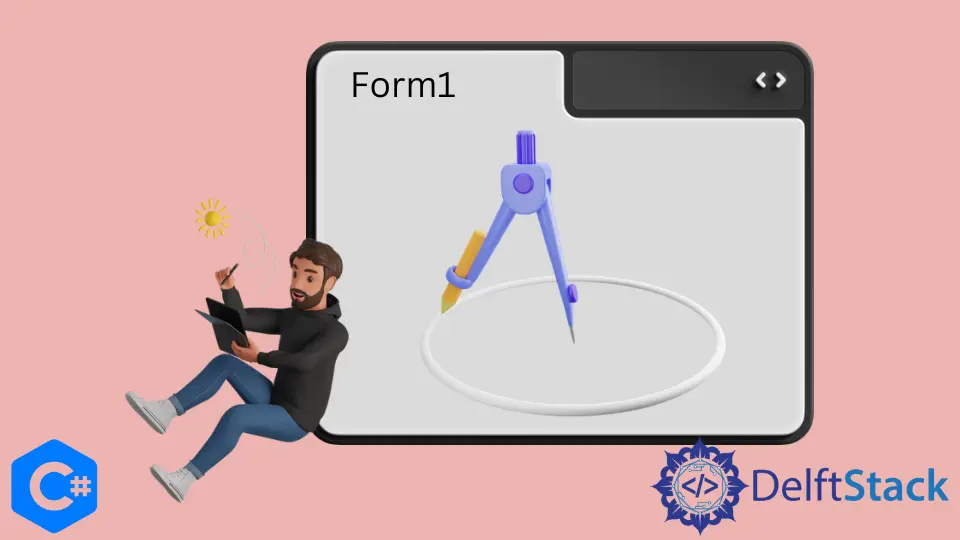
この記事では、C# で円を描く方法を見ていきます。
C#
で Drawing.Ellipse()
メソッドを使用して円を描く
System.Drawing
には明示的な円の描画がありません。 同じ機能を提供する Drawing.Ellipse()
メソッドを使用するか、Windows.FORMS(.NET FRAMEWORK)
で新しい FORM
を作成してインターフェイスを試すことができます。
.NET Framework を使用して C# で円を描く
FORM
を起動したときに Paint
メソッドが呼び出されることを確認してください。 フォームをダブルクリックすると、プロパティが開きます。
EVENTS
セクションに切り替えます。
EVENTS
セクション内で、PAINT
が見つかるまで下にスクロールし、それをダブルクリックして PAINT
関数を生成します。
ここで、System.Drawing
を使用して ELLIPSE
を作成し、楕円オプションを選択します。
e.Graphics.DrawEllipse(new Pen(System.Drawing.Color.Red), new Rectangle(10, 10, 50, 50));
赤のパラメータで新しい PEN
を選択しました。 次のパラメーターは、円をカプセル化する RECTANGLE
を描画する傾向があります。
最初の 2つのパラメーターは原点 ( x and y )
を表し、最後の 2つのパラメーターは x 軸と y 軸のサイズを表します。
出力:
C#
で FillEllipse()
メソッドを使用して円を塗りつぶす
上記の出力の円を塗りつぶすには、FILLELLIPSE()
関数を使用します。
e.Graphics.FillEllipse(Brushes.Red, 10, 10, 50, 50);
Drawing.Ellipse()
で行ったように、FillEllipse()
関数で同じポイントを定義して、正しい領域が塗りつぶされるようにします。 最初のパラメータとして Brushes.Red
ブラシを選択しました。
出力:
完全なコード スニペット:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WindowsFormsApp1 {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e) {}
private void Form1_Paint(object sender, PaintEventArgs e) {
e.Graphics.DrawEllipse(new Pen(System.Drawing.Color.Red), new Rectangle(10, 10, 50, 50));
e.Graphics.FillEllipse(Brushes.Red, 10, 10, 50, 50);
}
private void Form1_MouseHover(object sender, EventArgs e) {}
}
}
これが C# で円を描く方法です。 これをよく学び、必要に応じて変更できることを願っています。
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHub