C# で DataTable を Excel にエクスポートする
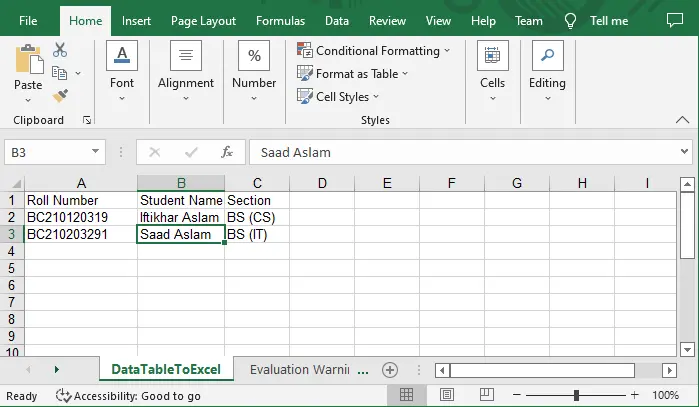
このチュートリアルでは、C# プログラミング言語でデータテーブルを Excel ファイルにエクスポートする方法を説明します。 このプロジェクトに進む前に、Excel ファイルを作成してエクスポートするための参照を追加する必要があります。
Visual Studio で参照パッケージを追加する
ExcelMapper
パッケージは、さまざまな Excel 機能を実行するために含まれます。 これを行うには、以下に概説する手順に従ってください。
Visual Studio,
を開き、Console Application,
を作成して名前を付けます。ソリューション エクスプローラー
パネルを右クリックし、NuGet パッケージの管理
を選択します。Browse
オプションをクリックし、ExcelMapper を検索してインストールします。
コード例でそれを理解しましょう。
C#
を使用して DataTable
を Excel にエクスポート
まず、次のライブラリをインポートします。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Data;
using Spire.Xls;
ここで、Workbook
オブジェクトの新しいインスタンスを生成し、Workbook
という名前で保存します。
Workbook workBook = new Workbook();
workBook.Worksheets.Clear();
Worksheet
クラスの新しいインスタンスを含めて、Worksheet
と呼びます。
Worksheet workSheet = workBook.Worksheets.Add("DataTableToExcel");
DataTable
という変数名で、タイプ DataTable
の新しいオブジェクトを作成します。
DataTable dataTable = new DataTable();
Roll Number, Student Name,
および Section.
というラベルの付いた新しい列を作成します。
dataTable.Columns.Add("Roll Number", typeof(String));
dataTable.Columns.Add("Student Name", typeof(String));
dataTable.Columns.Add("Section", typeof(String));
DataRow
クラスの新しいオブジェクトをインスタンス化し、dtr.
という名前を付ける必要があります。
DataRow dtr = dataTable.NewRow();
次に、DataRow.
という名前の列にデータを入力します。
dtr[0] = "BC210120319";
dtr[1] = "Iftikhar Aslam";
dtr[2] = "BS (CS)";
dataTable.Rows.Add(dtr);
dtr = dataTable.NewRow();
dtr[0] = "BC210203291";
dtr[1] = "Saad Aslam";
dtr[2] = "BS (IT)";
dataTable.Rows.Add(dtr);
dtr = dataTable.NewRow();
作成したばかりのスプレッドシートに、DataTable.
というラベルの付いた列を挿入する必要があります。
workSheet.InsertDataTable(dataTable, true, 1, 1, true);
この時点で、Excel ファイルとして保存する前にドキュメントに名前を付ける必要があります。
workBook.SaveToFile(@"E:\DataTableToExcel.xlsx", ExcelVersion.Version2016);
完全なソース コード:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Data;
using Spire.Xls;
namespace ExportDataTableToExcelBySaad {
class Program {
static void Main(string[] args) {
Workbook workBook = new Workbook();
workBook.Worksheets.Clear();
Worksheet workSheet = workBook.Worksheets.Add("DataTableToExcel");
DataTable dataTable = new DataTable();
dataTable.Columns.Add("Roll Number", typeof(String));
dataTable.Columns.Add("Student Name", typeof(String));
dataTable.Columns.Add("Section", typeof(String));
DataRow dtr = dataTable.NewRow();
dtr[0] = "BC210120319";
dtr[1] = "Iftikhar Aslam";
dtr[2] = "BS (CS)";
dataTable.Rows.Add(dtr);
dtr = dataTable.NewRow();
dtr[0] = "BC210203291";
dtr[1] = "Saad Aslam";
dtr[2] = "BS (IT)";
dataTable.Rows.Add(dtr);
dtr = dataTable.NewRow();
workSheet.InsertDataTable(dataTable, true, 1, 1, true);
workBook.SaveToFile(@"E:\DataTableToExcel.xlsx", ExcelVersion.Version2016);
}
}
}
出力:
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn