C++ で範囲外例外をスローする
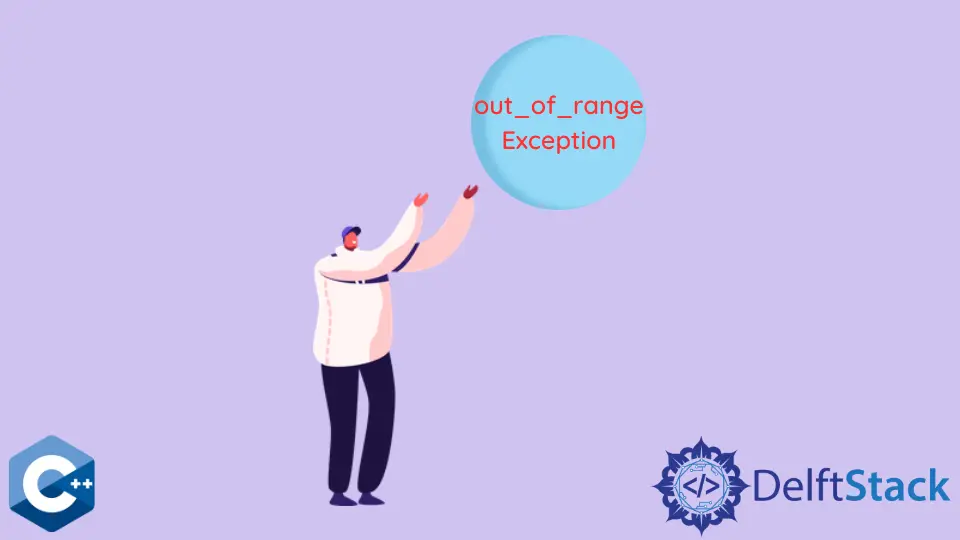
C++ では、try-catch
ブロックを使用して例外を処理できます。 throw
ステートメントを使用して明示的に例外をスローすることもできます。
この記事では、C++ で範囲外の例外をスローする方法について説明します。
C++ で範囲外例外をスローする
C++ で範囲外の例外をスローするには、例外オブジェクトを作成できます。 これには、out_of_range()
コンストラクターを使用できます。
out_of_range()
コンストラクターは、標準 C++ ライブラリで定義されています。 入力引数として文字列オブジェクトを取り、範囲外の例外を返します。
次の例に示すように、throw
ステートメントを使用して例外をスローできます。
#include <iostream>
int main() {
int number = 100;
if (number > 50) {
throw std::out_of_range("I am an exception.");
}
return 0;
}
出力:
terminate called after throwing an instance of 'std::out_of_range'
what(): I am an exception.
Aborted
ここでは、最初に number
という名前の変数を作成しました。 次に、number
の値が 50
より大きいかどうかを確認しました。
はいの場合、標準 C++ ライブラリで定義されている範囲外の例外がスローされています。
try-catch
ブロックを使用して、範囲外の例外を処理することもできます。 try
ブロックで例外をスローします。
範囲外の例外をキャッチし、catch
ブロックで例外を処理したことを出力します。 これは、次の例で確認できます。
#include <iostream>
int main() {
int number = 100;
try {
if (number > 50) {
throw std::out_of_range("I am an exception.");
}
} catch (std::out_of_range) {
std::cout << "I am here. Exception caught and handled.";
}
return 0;
}
出力:
I am here. Exception caught and handled.
上記の出力は、範囲外の例外を処理した後、プログラムが正常に終了したことを示しています。 一方、例外を処理しなかったため、前のプログラムは中止されました。
C++ で範囲外例外をスローする際のエラー
以下に示すように、C++ で範囲外の例外をスローするプログラムを作成できる場合があります。
#include <iostream>
int main() {
int number = 100;
try {
if (number > 50) {
throw std::out_of_range;
}
} catch (std::out_of_range) {
std::cout << "I am here. Exception caught and handled.";
}
return 0;
}
上記のコードを実行すると、プログラムは次の実行トレースでエラーになります。
/tmp/EG06BKsTcd.cpp: In function 'int main()':
/tmp/EG06BKsTcd.cpp:8:36: error: expected primary-expression before ';' token
8 | throw std::out_of_range;
| ^
範囲外の例外オブジェクトの代わりに std::out_of_range
をスローしたため、エラーが発生します。 std::out_of_range
コンストラクターは、範囲外の例外を作成するために使用される型定義です。
したがって、throw
ステートメントを使用してスローすることはできません。 C++ で範囲外の例外をスローするには、入力引数として文字列を std::out_of_range()
コンストラクターに渡して、範囲外の例外を作成する必要があります。 そうして初めて、C++ で範囲外の例外を正常にスローできるようになります。
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub