PyQt5 Tutorial - CheckBox
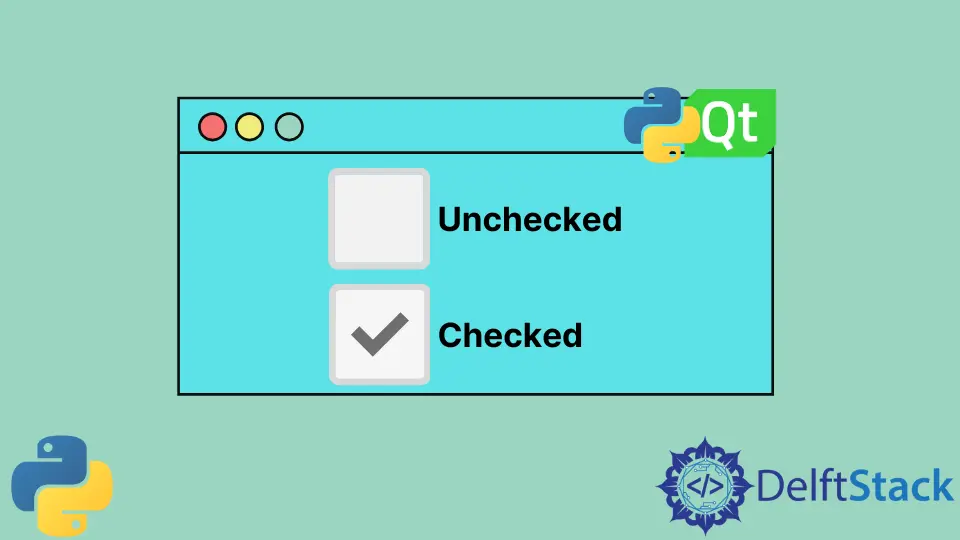
In questo tutorial impareremo QCheckBox
in PyQt5. Un QCheckBox
è un pulsante di opzione che può essere selezionato o deselezionato. L’utente può selezionare più opzioni dal gruppo di checkbox.
Esempio di CheckBox
import sys
from PyQt5.QtWidgets import QWidget, QLabel, QHBoxLayout, QCheckBox, QApplication
class basicWindow(QWidget):
def __init__(self):
super().__init__()
layout = QHBoxLayout()
self.setLayout(layout)
self.checkBoxA = QCheckBox("Select This.")
self.labelA = QLabel("Not slected.")
layout.addWidget(self.checkBoxA)
layout.addWidget(self.labelA)
self.setGeometry(200, 200, 300, 200)
self.setWindowTitle("CheckBox Example")
if __name__ == "__main__":
app = QApplication(sys.argv)
windowExample = basicWindow()
windowExample.show()
sys.exit(app.exec_())
Dove,
self.checkBoxA = QCheckBox("Select This.")
self.checkBoxA
è un’istanza del widget QCheckBox
in PyQt5. Il testo dato - Select This.
verrà visualizzato accanto al quadrato cavo CheckBox
.
Evento CheckBox
Fondamentalmente un utente dovrebbe selezionare o deselezionare la casella di controllo, poi l’azione dovrebbe essere eseguita in base al segnale di cambio di stato.
import sys
from PyQt5.QtWidgets import QWidget, QLabel, QHBoxLayout, QCheckBox, QApplication
from PyQt5 import QtCore
class basicWindow(QWidget):
def __init__(self):
super().__init__()
layout = QHBoxLayout()
self.setLayout(layout)
self.checkBoxA = QCheckBox("Select This.")
self.labelA = QLabel("Not slected.")
self.checkBoxA.stateChanged.connect(self.checkBoxChangedAction)
layout.addWidget(self.checkBoxA)
layout.addWidget(self.labelA)
self.setGeometry(200, 200, 300, 200)
self.setWindowTitle("CheckBox Example")
def checkBoxChangedAction(self, state):
if QtCore.Qt.Checked == state:
self.labelA.setText("Selected.")
else:
self.labelA.setText("Not Selected.")
if __name__ == "__main__":
app = QApplication(sys.argv)
windowExample = basicWindow()
windowExample.show()
sys.exit(app.exec_())
self.checkBoxA.stateChanged.connect(self.checkBoxChangedAction)
Colleghiamo il metodo dello slot checkBoxChangeAction()
al segnale CheckBox stateChanged
. Ogni volta che l’utente spunta o deseleziona la casella di controllo, chiamerà checkBoxChangeAction()
.
def checkBoxChangedAction(self, state):
if QtCore.Qt.Checked == state:
self.labelA.setText("Selected.")
else:
self.labelA.setText("Not Selected.")
L’argomento state
è lo stato del CheckBox
passato e il testo labelA
cambierà in Selected.
se il CheckBox
è spuntato, o in Not Selected.
se è deselezionato.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook