Pandas Copia DataFrame
-
Sintassi del metodo
pandas.DataFrame.copy()
-
Copia Pandas DataFrame usando il metodo
pandas.DataFrame.copy()
- Copia Pandas DataFrame assegnando il DataFrame a una variabile
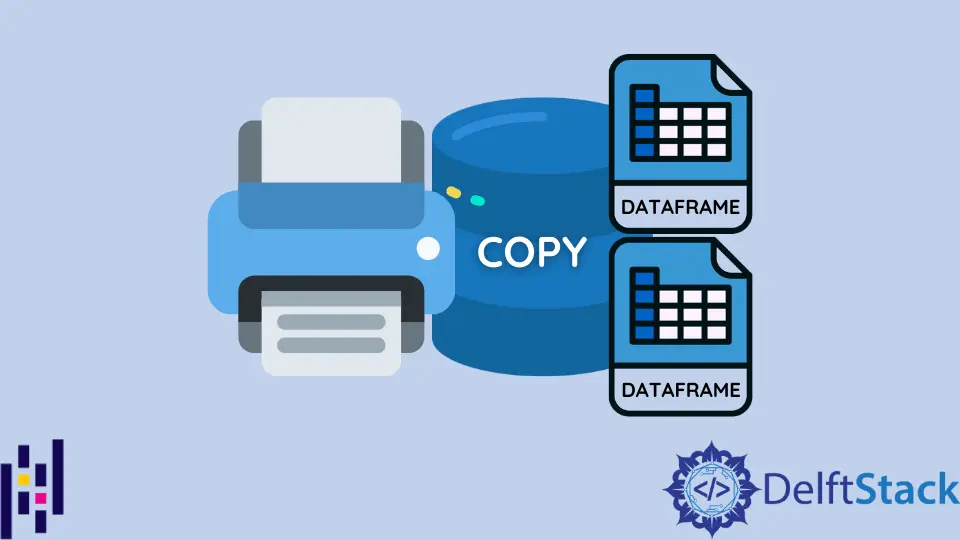
Questo tutorial introdurrà come copiare un oggetto DataFrame usando il metodo DataFrame.copy()
.
import pandas as pd
items_df = pd.DataFrame(
{
"Id": [302, 504, 708],
"Cost": ["300", "400", "350"],
}
)
print(items_df)
Produzione:
Id Cost
0 302 300
1 504 400
2 708 350
Useremo l’esempio sopra per dimostrare l’uso del metodo DataFrame.copy()
in Pandas.
Sintassi del metodo pandas.DataFrame.copy()
DataFrame.copy(deep=True)
Restituisce una copia del DataFrame
. deep
è per impostazione predefinita True
, il che significa che qualsiasi modifica apportata alla copia non si rifletterà nel DataFrame originale. Tuttavia, se impostiamo deep=False
, tutte le modifiche apportate alla copia si rifletteranno anche nel DataFrame originale.
Copia Pandas DataFrame usando il metodo pandas.DataFrame.copy()
import pandas as pd
import numpy as np
items_df = pd.DataFrame(
{
"Id": [302, 504, 708],
"Cost": ["300", "400", "350"],
}
)
deep_copy = items_df.copy()
print("Original DataFrame before changing value in copy DataFrame:")
print(items_df, "\n")
print("Copy DataFrame before changing value in copy DataFrame:")
print(deep_copy, "\n")
deep_copy.loc[0, "Cost"] = np.nan
print("Original DataFrame after changing value in copy DataFrame:")
print(items_df, "\n")
print("Copy DataFrame after changing value in copy DataFrame:")
print(deep_copy, "\n")
Produzione:
Original DataFrame before changing value in copy DataFrame:
Id Cost
0 302 300
1 504 400
2 708 350
Copy DataFrame before changing value in copy DataFrame:
Id Cost
0 302 300
1 504 400
2 708 350
Original DataFrame after changing value in copy DataFrame:
Id Cost
0 302 300
1 504 400
2 708 350
Copy DataFrame after changing value in copy DataFrame:
Id Cost
0 302 NaN
1 504 400
2 708 350
Crea una copia del DataFrame items_df
come deep_copy
. Se modifichiamo qualsiasi valore della copia deep_copy
, non ci sarà alcun cambiamento nel DataFrame originale items_df
. Abbiamo impostato il valore della colonna Cost
della prima riga su NaN
in deep_copy
ma items_df
non viene modificato.
Copia Pandas DataFrame assegnando il DataFrame a una variabile
import pandas as pd
import numpy as np
items_df = pd.DataFrame(
{
"Id": [302, 504, 708],
"Cost": ["300", "400", "350"],
}
)
copy_cost = items_df["Cost"]
print("Cost column of Original DataFrame before changing value in copy DataFrame:")
print(items_df, "\n")
print("Cost column of Copied DataFrame before changing value in copy DataFrame:")
print(copy_cost, "\n")
copy_cost[0] = np.nan
print("Cost column of Original DataFrame after changing value in copy DataFrame:")
print(copy_cost, "\n")
print("Cost column of Copied DataFrame after changing value in copy DataFrame:")
print(copy_cost, "\n")
Produzione:
Cost column of Original DataFrame before changing value in copy DataFrame:
Id Cost
0 302 300
1 504 400
2 708 350
Cost column of Copied DataFrame before changing value in copy DataFrame:
0 300
1 400
2 350
Name: Cost, dtype: object
Cost column of Original DataFrame after changing value in copy DataFrame:
0 NaN
1 400
2 350
Name: Cost, dtype: object
Cost column of Copied DataFrame after changing value in copy DataFrame:
0 NaN
1 400
2 350
Name: Cost, dtype: object
Crea una copia della colonna Cost
del DataFrame items_df
come copy_cost
.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedInArticolo correlato - Pandas DataFrame
- Come ottenere le intestazioni delle colonne DataFrame Pandas come lista
- Come cancellare la colonna DataFrame Pandas DataFrame
- Come convertire la colonna DataFrame in data e ora in pandas
- Converti un Float in un Integer in Pandas DataFrame
- Ordina Pandas DataFrame in base ai valori di una colonna
- Ottieni l'aggregato di Pandas Group-By e Sum