Crea array di strutture in C++
- Utilizzare la dichiarazione di matrice in stile C per creare un array di strutture a lunghezza fissa
-
Usa
std::vector
e il costruttore dell’lista di inizializzatori per creare array di strutture a lunghezza variabile
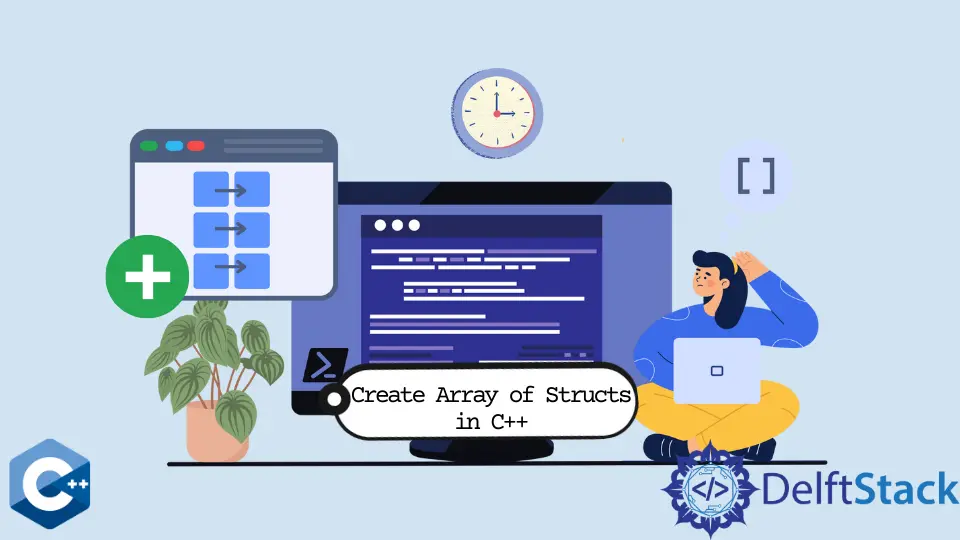
Questo articolo illustrerà più metodi su come creare un array di strutture in C++.
Utilizzare la dichiarazione di matrice in stile C per creare un array di strutture a lunghezza fissa
Gli array di strutture a lunghezza fissa possono essere dichiarati usando la notazione di array in stile []
. In questo caso, abbiamo definito una struct
arbitraria denominata Company
con più membri di dati e un array di 2 elementi inizializzato. L’unico svantaggio di questo metodo è che l’array dichiarato è un oggetto grezzo senza alcuna funzione incorporata. Tra i lati positivi, può essere una struttura dati più efficiente e veloce rispetto ai contenitori di librerie C++.
#include <iostream>
#include <string>
#include <vector>
using std::cout;
using std::endl;
using std::string;
using std::vector;
struct Company {
string name;
string ceo;
float income;
int employess;
};
int main() {
Company comp_arr[2] = {{"Intel", "Bob Swan", 91213.11, 110823},
{"Apple", "Tim Cook", 131231.11, 137031}};
for (const auto &arr : comp_arr) {
cout << "Name: " << arr.name << endl
<< "CEO: " << arr.ceo << endl
<< "Income: " << arr.income << endl
<< "Employees: " << arr.employess << endl
<< endl;
}
return EXIT_SUCCESS;
}
Produzione:
Name: Intel
CEO: Bob Swan
Income: 91213.1
Employees: 110823
Name: Apple
CEO: Tim Cook
Income: 131231
Employees: 137031
Usa std::vector
e il costruttore dell’lista di inizializzatori per creare array di strutture a lunghezza variabile
In alternativa, possiamo utilizzare un contenitore std::vector
per dichiarare un array di variabili che fornisce più metodi incorporati per la manipolazione dei dati. L’oggetto std::vector
può essere inizializzato con la stessa notazione dell’esempio precedente. Nuovi elementi possono essere aggiunti all’array usando il tradizionale metodo push_back
e l’ultimo elemento rimosso con pop_back
. In questo esempio, gli elementi vengono stampati sulla console uno per uno.
Si noti che i membri dell’lista di inizializzatori devono includere parentesi graffe esterne per l’assegnazione e la formattazione corrette.
#include <iostream>
#include <string>
#include <vector>
using std::cout;
using std::endl;
using std::string;
using std::vector;
struct Company {
string name;
string ceo;
float income;
int employess;
};
int main() {
vector<Company> comp_arr = {{"Intel", "Bob Swan", 91213.11, 110823},
{"Apple", "Tim Cook", 131231.11, 137031}};
for (const auto &arr : comp_arr) {
cout << "Name: " << arr.name << endl
<< "CEO: " << arr.ceo << endl
<< "Income: " << arr.income << endl
<< "Employees: " << arr.employess << endl
<< endl;
}
return EXIT_SUCCESS;
}
Produzione:
Name: Intel
CEO: Bob Swan
Income: 91213.1
Employees: 110823
Name: Apple
CEO: Tim Cook
Income: 131231
Employees: 137031
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook