Méthode de mise en veille des threads dans Scala
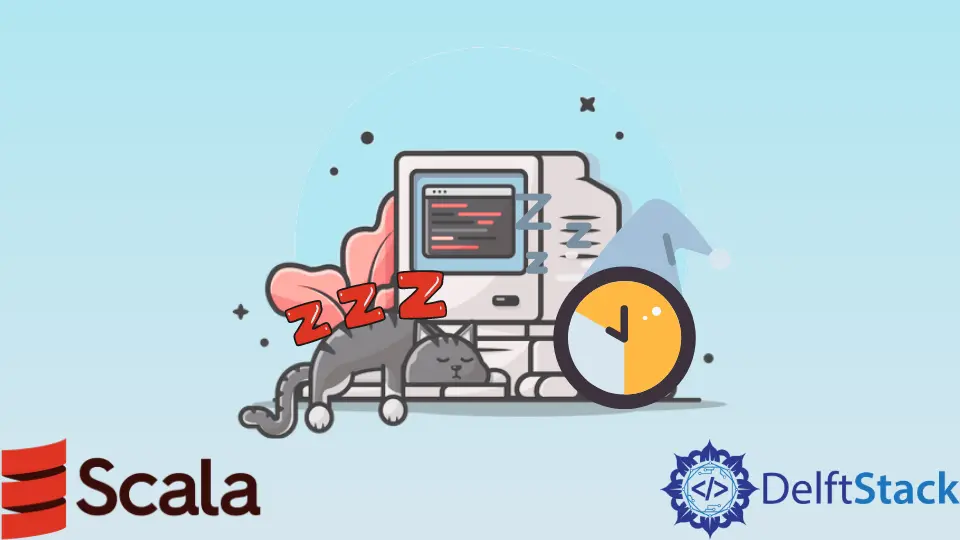
Tout d’abord, cet article apprendra comment les threads sont implémentés dans Scala. Ensuite, nous verrons comment fonctionne la méthode Sleep dans Scala.
Threads en Scala
Les threads sont des sous-processus légers qui prennent moins d’espace et s’exécutent plus rapidement. Dans un environnement multithread, de nombreux threads s’exécutent simultanément, chacun exécutant une tâche différente. Cela conduit à une meilleure utilisation du processeur et des ressources, et à son tour, nous obtenons un meilleur débit.
Dans Scala, les threads sont créés de deux manières : la première consiste à étendre l’interface Runnable
, et la seconde à étendre la classe Thread
.
Méthode 1 : Extension de l’interface exécutable
Nous créons une classe qui étend l’interface Runnable et remplace la méthode run().
Exemple de code :
class test extends Runnable
{
override def run()
{
println("Thread "+Thread.currentThread().getName()+" is running.")
}
}
object MyClass {
def main(args: Array[String]) {
for(i<- 1 to 5)
{
var obj = new Thread(new test()) //creating the object
obj.setName(i.toString())
obj.start() //starts the thread
}
}
}
Production :
Thread 1 is running.
Thread 2 is running.
Thread 3 is running.
Thread 4 is running.
Thread 5 is running.
Méthode 2 : Extension de la classe Thread
Nous créons une classe qui étend la classe Thread et redéfinit la méthode run().
Exemple de code :
class test extends Thread
{
override def run()
{
println("Thread "+Thread.currentThread().getName()+" is running.")
}
}
object MyClass {
def main(args: Array[String]) {
for(i<- 1 to 5)
{
var obj = new Thread(new test()) //creating the object
obj.setName(i.toString())
obj.start() //starts the thread
}
}
}
Production :
Thread 1 is running.
Thread 2 is running.
Thread 3 is running.
Thread 4 is running.
Thread 5 is running.
Thread.sleep()
en Scala
Thread.sleep(time)
est utilisé pour mettre en veille un thread pendant une durée spécifique. Le temps en millisecondes lui est passé en argument.
Syntaxe:
Thread.sleep(1000)
Here the thread would sleep for 1000 milliseconds
Exemple de code :
class test extends Thread
{
override def run()
{
var i = 0
println("Thread "+Thread.currentThread().getName()+" is running.")
for(i<-1 to 5)
{
Thread.sleep(1000) //will sleep the thread
}
}
}
object MyClass {
def main(args: Array[String]) {
for(i<- 1 to 5)
{
var obj = new Thread(new test()) //creating the object
var thread1 = new Thread(obj);
var thread2 = new Thread(obj);
thread1.setName("1")
thread2.setName("2")
thread1.start() //starts the thread
thread2.start()
}
}
}
Production :
Thread 1 is running.
Thread 2 is running.
Thread 1 is running.
Thread 2 is running.
Thread 1 is running.
Thread 2 is running.
Thread 1 is running.
Thread 2 is running.
Thread 1 is running.
Thread 2 is running.