Método de suspensión de subprocesos en Scala
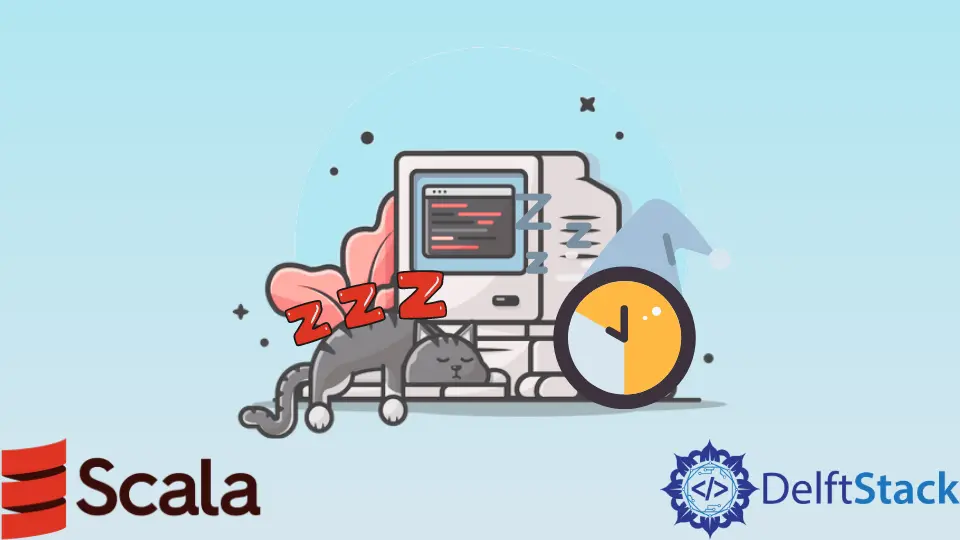
Primero, este artículo aprenderá cómo se implementan los subprocesos en Scala. Luego, veremos cómo funciona el método Sleep en Scala.
Hilos en Scala
Los subprocesos son subprocesos livianos que ocupan menos espacio y se ejecutan más rápido. En un entorno de subprocesos múltiples, muchos subprocesos se ejecutan simultáneamente y cada uno realiza una tarea diferente. Esto conduce a una mejor utilización de la CPU y los recursos y, a su vez, obtenemos un mejor rendimiento.
En Scala, los hilos se crean de dos maneras: primero, se extiende la interfaz Runnable
, y el segundo se extiende la clase Thread
.
Método 1: Ampliación de la interfaz ejecutable
Creamos una clase que amplía la interfaz Runnable y anula el método run().
Código de ejemplo:
class test extends Runnable
{
override def run()
{
println("Thread "+Thread.currentThread().getName()+" is running.")
}
}
object MyClass {
def main(args: Array[String]) {
for(i<- 1 to 5)
{
var obj = new Thread(new test()) //creating the object
obj.setName(i.toString())
obj.start() //starts the thread
}
}
}
Producción :
Thread 1 is running.
Thread 2 is running.
Thread 3 is running.
Thread 4 is running.
Thread 5 is running.
Método 2: Ampliación de la clase de subprocesos
Creamos una clase que extiende la clase Thread y anula el método run()
.
Código de ejemplo:
class test extends Thread
{
override def run()
{
println("Thread "+Thread.currentThread().getName()+" is running.")
}
}
object MyClass {
def main(args: Array[String]) {
for(i<- 1 to 5)
{
var obj = new Thread(new test()) //creating the object
obj.setName(i.toString())
obj.start() //starts the thread
}
}
}
Producción :
Thread 1 is running.
Thread 2 is running.
Thread 3 is running.
Thread 4 is running.
Thread 5 is running.
Thread.sleep()
en Scala
Thread.sleep(time)
se utiliza para dormir un hilo durante un período de tiempo específico. El tiempo en milisegundos se le pasa como argumento.
Sintaxis:
Thread.sleep(1000)
Here the thread would sleep for 1000 milliseconds
Código de ejemplo:
class test extends Thread
{
override def run()
{
var i = 0
println("Thread "+Thread.currentThread().getName()+" is running.")
for(i<-1 to 5)
{
Thread.sleep(1000) //will sleep the thread
}
}
}
object MyClass {
def main(args: Array[String]) {
for(i<- 1 to 5)
{
var obj = new Thread(new test()) //creating the object
var thread1 = new Thread(obj);
var thread2 = new Thread(obj);
thread1.setName("1")
thread2.setName("2")
thread1.start() //starts the thread
thread2.start()
}
}
}
Producción :
Thread 1 is running.
Thread 2 is running.
Thread 1 is running.
Thread 2 is running.
Thread 1 is running.
Thread 2 is running.
Thread 1 is running.
Thread 2 is running.
Thread 1 is running.
Thread 2 is running.