Obtener el tamaño de una matriz pasada como argumento
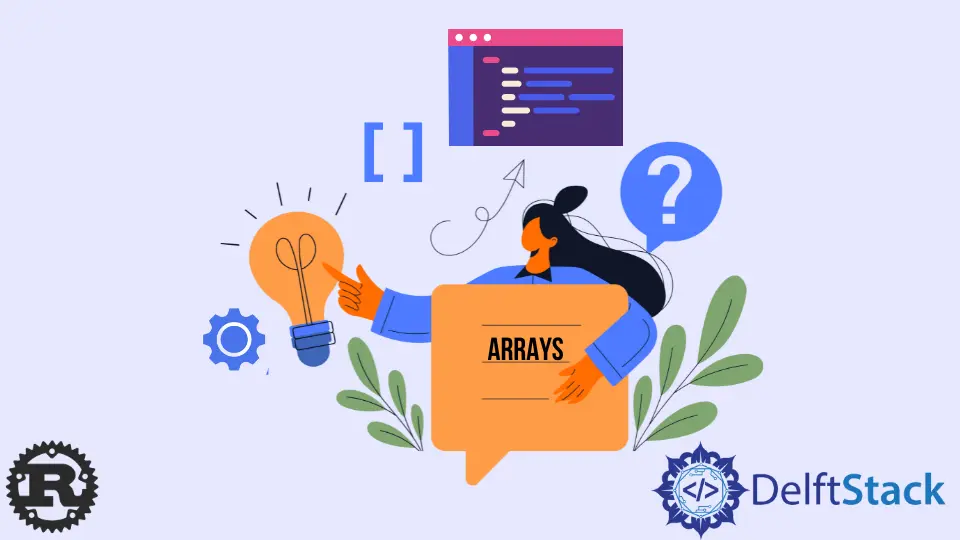
Este artículo trata sobre arreglos y cómo obtener el tamaño de un arreglo pasado como argumento en Rust.
Matriz en Rust
Una matriz se conoce como la colección homogénea de diferentes valores. También podemos decir que una matriz es la colección de valores que tienen el mismo tipo de datos.
Los bloques de memoria secuencial se asignan una vez que se declara la matriz. No se pueden cambiar de tamaño una vez inicializados, ya que son estáticos.
Cada bloque de memoria representa un elemento de matriz. Los valores de un elemento de matriz se pueden actualizar o modificar, pero no se pueden eliminar.
Un ejemplo de una matriz simple se escribe a continuación.
fn main(){
let arr:[i32;5] = [11,12,13,14,15];
println!("Array is {:?}",arr);
println!("The size of the array is: {}",arr.len());
}
Producción :
Array is [11, 12, 13, 14, 15]
The size of the array is: 5
El siguiente código de ejemplo crea la matriz y todos sus elementos se inicializan con el valor predeterminado de -1.
fn main() {
let arr:[i32;4] = [-1;4];
println!("The array is {:?}",arr);
println!("The size of the array is: {}",arr.len());
}
Producción :
The array is [-1, -1, -1, -1]
The size of the array is: 4
Use la función iter()
para obtener valores de elementos de matriz en Rust
La función iter()
se utiliza para obtener los valores de todos los elementos disponibles en una matriz.
Código de ejemplo:
fn main(){
let num:[i32;4] = [50,60,70,80];
println!("The array is {:?}",num);
println!("The size of the array is: {}",num.len());
for val in num.iter(){
println!("The value of the array is: {}",val);
}
}
Producción :
The array is [50, 60, 70, 80]
The size of the array is: 4
The value of the array is: 50
The value of the array is: 60
The value of the array is: 70
The value of the array is: 80