Comprobar valores faltantes mediante un operador booleano en R
- Error al verificar valores existentes y valores faltantes en R
-
Use la función
is.na()
para buscar valores faltantes en R - Conclusión
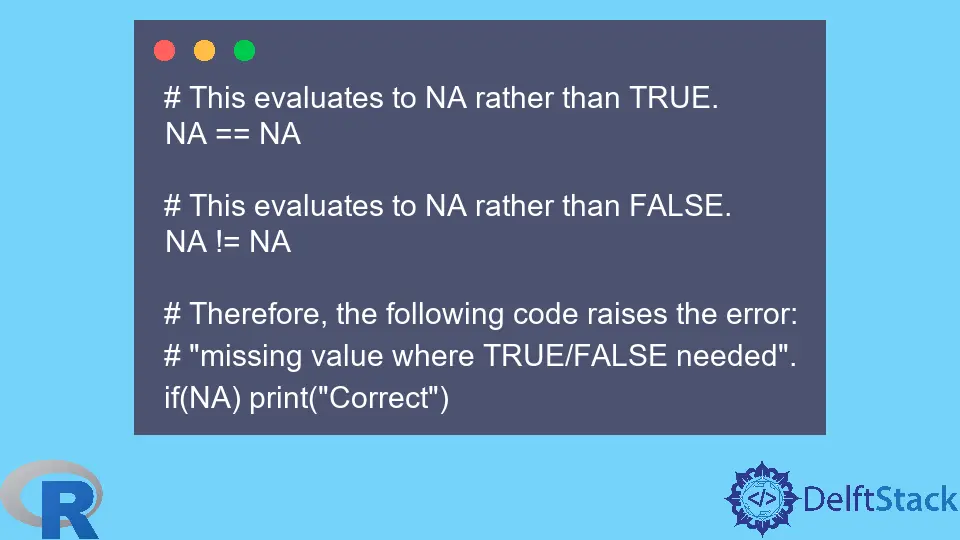
Al analizar datos, podemos importar datos de una fuente externa, como un archivo CSV. Los datos pueden contener valores faltantes marcados como NA
.
Si necesitamos verificar diferentes valores en nuestros datos, o si necesitamos verificar NA
, primero debemos abordar los valores que faltan para evitar errores. Veremos cómo hacerlo en este artículo.
Crearemos un vector simple para demostrar el problema y la solución.
Código de muestra:
myVec = c(50, 60, NA, 40, 80)
Error al verificar valores existentes y valores faltantes en R
Primero, comprobemos el valor 60
, que sabemos que existe en el vector.
Después de eso, buscaremos un valor faltante. Ambos dan el mismo error.
Código de muestra:
# Value 60 exists.
for(i in 1:length(myVec))
if(myVec[i] == 60) {print("Present")}
# A missing value, NA, exists.
for(i in 1:length(myVec))
if(myVec[i] == NA) {print("Missing")}
Producción :
> # Value 60 exists.
> for(i in 1:length(myVec))
+ if(myVec[i] == 60) {print("Present")}
[1] "Present"
Error in if (myVec[i] == 60) { : missing value where TRUE/FALSE needed
> # A missing value, NA, exists.
> for(i in 1:length(myVec))
+ if(myVec[i] == NA) {print("Missing")}
Error in if (myVec[i] == NA) { : missing value where TRUE/FALSE needed
Obtuvimos ese error porque la condición booleana que ingresamos en la declaración if
compara un valor con NA
o NA
con NA
. Estas condiciones booleanas evalúan NA
en lugar de VERDADERO
o FALSO
.
Código de muestra:
# This evaluates to NA rather than TRUE.
NA == NA
# This evaluates to NA rather than FALSE.
NA != NA
# Therefore, the following code raises the error:
# "missing value where TRUE/FALSE needed".
if(NA) print("Correct")
Producción :
> # This evaluates to NA rather than TRUE.
> NA == NA
[1] NA
>
> # This evaluates to NA rather than FALSE.
> NA != NA
[1] NA
>
> # Therefore, the following code raises the error:
> # "missing value where TRUE/FALSE needed".
> if(NA) print("Correct")
Error in if (NA) print("Correct") : missing value where TRUE/FALSE needed
Use la función is.na()
para buscar valores faltantes en R
Para sortear el problema causado por los valores faltantes, necesitamos identificar los valores faltantes usando la función is.na()
. Se pueden manejar utilizando una secuencia de condiciones if
y else
o condiciones anidadas if
y else
.
El requisito básico se encuentra a continuación.
- Los valores
NA
deben coincidir por separado de todos los demás valores. - Al verificar otros valores, debemos excluir los valores
NA
explícitamente.
Código de muestra:
# Using a sequence of if and else conditions.
for(i in 1:length(myVec)){
if(!is.na(myVec[i]) & myVec[i] == 60){
print("Match found")} else
if(!is.na(myVec[i]) & myVec[i] != 60){
print("Match not found")} else
if(is.na(myVec[i])) {
print("Found NA")}
}
# Using a nested if.
for(i in 1:length(myVec)){
if(!is.na(myVec[i])){
if(myVec[i]==60){
print("Match Found")} else {
print("Match not found")}
} else {
print("Found NA")}
}
Producción :
> # Using a sequence of if and else conditions.
> for(i in 1:length(myVec)){
+ if(!is.na(myVec[i]) & myVec[i] == 60){
+ print("Match found")} else
+ if(!is.na(myVec[i]) & myVec[i] != 60){
+ print("Match not found")} else
+ if(is.na(myVec[i])) {
+ print("Found NA")}
+ }
[1] "Match not found"
[1] "Match found"
[1] "Found NA"
[1] "Match not found"
[1] "Match not found"
>
> # Using a nested if.
> for(i in 1:length(myVec)){
+ if(!is.na(myVec[i])){
+ if(myVec[i]==60){
+ print("Match Found")} else {
+ print("Match not found")}
+ } else {
+ print("Found NA")}
+ }
[1] "Match not found"
[1] "Match Found"
[1] "Found NA"
[1] "Match not found"
[1] "Match not found"
Conclusión
Siempre que exista la posibilidad de que nuestros datos tengan valores faltantes, debemos escribir un código que separe los valores faltantes de otros valores.