Cómo comprobar si un valor existe en la lista de Python de forma rápida
-
Método
in
para comprobar la existencia de valores en la lista de Python - Convertir la lista en set y luego hacer el chequeo de membresía en Python
- Comparación de rendimiento entre la lista y el conjunto de comprobación de pertenencia
- Conclusión de la comparación del rendimiento
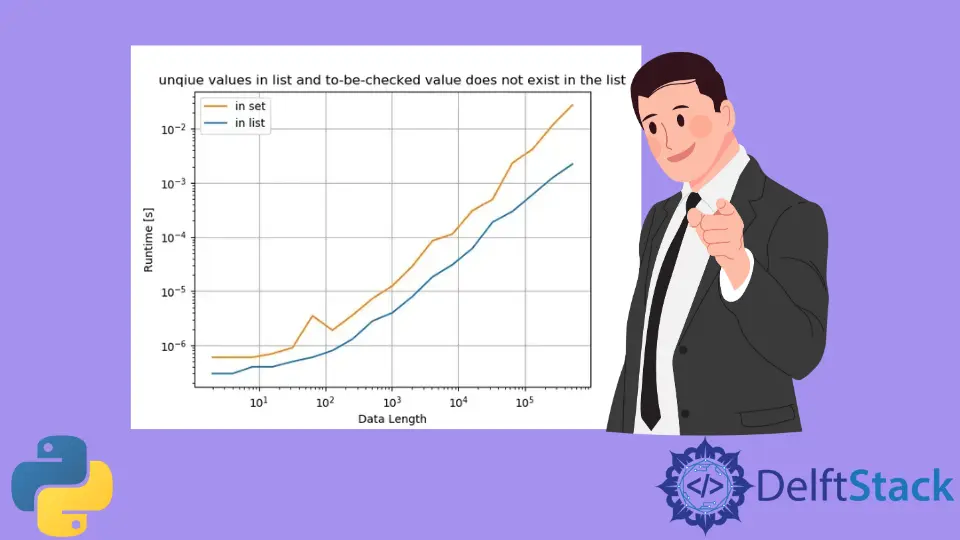
Introduciremos diferentes métodos para comprobar si existe un valor en la lista de Python y comparar su rendimiento.
Los métodos incluyen,
- Método de comprobación de pertenencia -
in
Method para comprobar si el valor existe - Convierta la lista a
set
y luego use el método de comprobación de pertenenciain
Método in
para comprobar la existencia de valores en la lista de Python
in
es la manera apropiada de hacer el chequeo de membresía en la lista de Python, set, diccionario u otros objetos iterables de Python.
>>> testList = [1, 2, 3, 4]
>>> 2 in testList
True
>>> 6 in testList
False
Convertir la lista en set y luego hacer el chequeo de membresía en Python
La lista de verificación de miembros podría ser ineficiente si el tamaño de la lista aumenta, especialmente si existen elementos duplicados en la lista.
El conjunto de Python es un tipo de datos mejor en este escenario para realizar la comprobación de pertenencia porque sólo contiene valores únicos.
Comparación de rendimiento entre la lista y el conjunto de comprobación de pertenencia
Compararemos las diferencias de rendimiento en cuatro situaciones,
- La lista original tiene valores únicos y el valor verificado existe en la lista
- La lista original tiene valores únicos y el valor verificado no existe en la lista
- La lista original tiene valores duplicados y el valor verificado existe en la lista
- La lista original sólo tiene valores duplicados, y el valor comprobado no existe en la lista
La lista original sólo tiene valores unívocos y el valor verificado existe en la lista
from itertools import chain
import perfplot
import numpy as np
def setupTest(n):
a = np.arange(n)
np.random.shuffle(a)
randomlist = a[: n // 2].tolist()
randomvalue = randomlist[len(randomlist) // 2]
return [randomlist, randomvalue]
def inListMethod(L):
x, y = L
return y in x
def inSetMethod(L):
x, y = L
x = set(x)
return y in x
perfplot.show(
setup=setupTest,
kernels=[inListMethod, inSetMethod],
labels=["in list", "in set"],
n_range=[2 ** k for k in range(1, 20)],
xlabel="Data Length",
title="unique values in list and to-be-checked value exists in the list",
logx=True,
logy=True,
)
La lista original sólo tiene valores únicos y el valor verificado no existe en la lista
from itertools import chain
import perfplot
import numpy as np
def setupTest(n):
a = np.arange(n)
np.random.shuffle(a)
randomlist = a[: n // 2].tolist()
randomvalue = n + 1
return [randomlist, randomvalue]
def inListMethod(L):
x, y = L
return y in x
def inSetMethod(L):
x, y = L
x = set(x)
return y in x
perfplot.show(
setup=setupTest,
kernels=[inListMethod, inSetMethod],
labels=["in list", "in set"],
n_range=[2 ** k for k in range(1, 20)],
xlabel="Data Length",
title="unique values in list and to-be-checked value does not exist in the list",
logx=True,
logy=True,
)
La lista original tiene valores duplicados y el valor verificado existe en la lista
from itertools import chain
import perfplot
import numpy as np
def setupTest(n):
a = np.arange(n)
np.random.shuffle(a)
randomlist = np.random.choice(n, n // 2).tolist()
randomvalue = randomlist[len(randomlist) // 2]
return [randomlist, randomvalue]
def inListMethod(L):
x, y = L
return y in x
def inSetMethod(L):
x, y = L
x = set(x)
return y in x
perfplot.show(
setup=setupTest,
kernels=[inListMethod, inSetMethod],
labels=["in list", "in set"],
n_range=[2 ** k for k in range(2, 20)],
xlabel="Data Length",
title="duplicate values in list and to-be-checked value exists in the list",
logx=True,
logy=True,
)
La lista original sólo tiene valores duplicados, y el valor verificado no existe en la lista
from itertools import chain
import perfplot
import numpy as np
def setupTest(n):
a = np.arange(n)
np.random.shuffle(a)
randomlist = np.random.choice(n, n // 2).tolist()
randomvalue = n + 1
return [randomlist, randomvalue]
def inListMethod(L):
x, y = L
return y in x
def inSetMethod(L):
x, y = L
x = set(x)
return y in x
perfplot.show(
setup=setupTest,
kernels=[inListMethod, inSetMethod],
labels=["in list", "in set"],
n_range=[2 ** k for k in range(2, 20)],
xlabel="Data Length",
title="duplicate values in list and to-be-checked value does not exist in the list",
logx=True,
logy=True,
)
Conclusión de la comparación del rendimiento
Aunque la comprobación de la membresía en el set
de Python es más rápida que la de la lista de Python, la conversión de la lista o del set
consume tiempo. Por lo tanto, si los datos dados son de la lista de Python, no tiene ningún beneficio de rendimiento si primero conviertes la lista a set
y luego haces el chequeo de membresía en set
.
from itertools import chain
import perfplot
import numpy as np
def setupTest(n):
a = np.arange(n)
np.random.shuffle(a)
unique_randomlist = a[: n // 2].tolist()
duplicate_randomlist = np.random.choice(n, n // 2).tolist()
existing_randomvalue = unique_randomlist[len(unique_randomlist) // 2]
nonexisting_randomvalue = n + 1
return [
unique_randomlist,
duplicate_randomlist,
existing_randomvalue,
nonexisting_randomvalue,
]
def inListMethod_UniqueValue_ValueExisting(L):
u, d, ex, ne = L
return ex in u
def inListMethod_DuplicateValue_ValueExisting(L):
u, d, ex, ne = L
return ex in d
def inListMethod_UniqueValue_ValueNotExisting(L):
u, d, ex, ne = L
return ne in u
def inListMethod_DuplicateValue_ValueNotExisting(L):
u, d, ex, ne = L
return ne in d
def inSetMethod_UniqueValue_ValueExisting(L):
u, d, ex, ne = L
u = set(u)
return ex in u
def inSetMethod_DuplicateValue_ValueExisting(L):
u, d, ex, ne = L
d = set(d)
return ex in d
def inSetMethod_UniqueValue_ValueNotExisting(L):
u, d, ex, ne = L
u = set(u)
return ne in u
def inSetMethod_DuplicateValue_ValueNotExisting(L):
u, d, ex, ne = L
d = set(d)
return ne in d
perfplot.show(
setup=setupTest,
equality_check=None,
kernels=[
inListMethod_UniqueValue_ValueExisting,
inListMethod_DuplicateValue_ValueExisting,
inListMethod_UniqueValue_ValueNotExisting,
inListMethod_DuplicateValue_ValueNotExisting,
inSetMethod_UniqueValue_ValueExisting,
inSetMethod_DuplicateValue_ValueExisting,
inSetMethod_UniqueValue_ValueNotExisting,
inSetMethod_DuplicateValue_ValueNotExisting,
],
labels=[
"inListMethod_UniqueValue_ValueExisting",
"inListMethod_DuplicateValue_ValueExisting",
"inListMethod_UniqueValue_ValueNotExisting",
"inListMethod_DuplicateValue_ValueNotExisting",
"inSetMethod_UniqueValue_ValueExisting",
"inSetMethod_DuplicateValue_ValueExisting",
"inSetMethod_UniqueValue_ValueNotExisting",
"inSetMethod_DuplicateValue_ValueNotExisting",
],
n_range=[2 ** k for k in range(2, 20)],
xlabel="Data Length",
logx=True,
logy=True,
)
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookArtículo relacionado - Python List
- Convertir un diccionario en una lista en Python
- Eliminar todas las apariciones de un elemento de una lista en Python
- Eliminar duplicados de una lista en Python
- Cómo obtener el promedio de una lista en Python
- ¿Cuál es la diferencia entre los métodos de lista que añaden y amplían
- Cómo convertir una lista en cadena en Python