Utilice KeyListener en Java
-
Clase
KeyEvent
-
Implementación de la interfaz
KeyListener
- Una aplicación simple de KeyListener
- Una aplicación de juego simple usando KeyListener
- Resumen
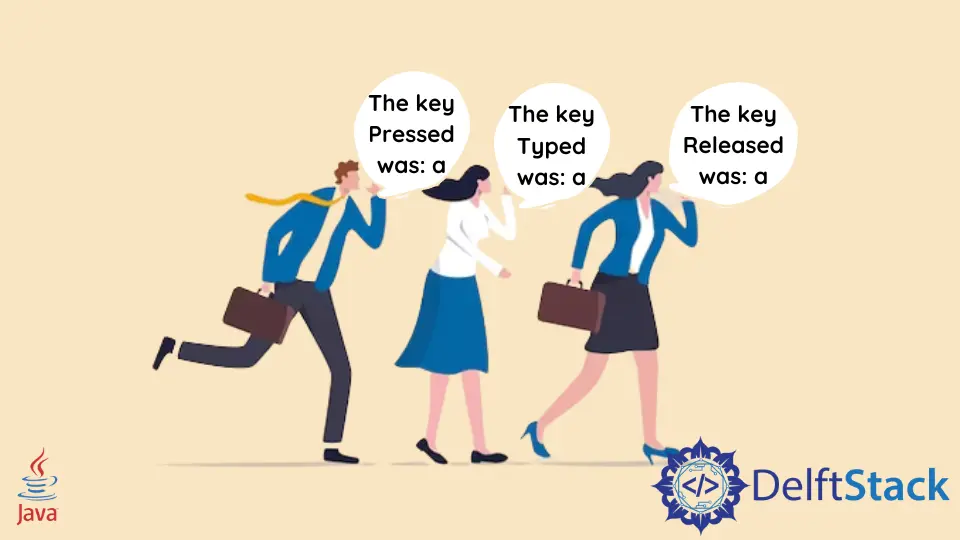
Este tutorial presenta cómo usar KeyListener en Java y enumera algunos códigos de ejemplo para comprender el tema.
KeyListener
es una interfaz que se ocupa de los cambios en el estado de las teclas de nuestro teclado. Como sugiere el nombre de la interfaz, escucha las claves y actúa en consecuencia.
En este tutorial, aprenderemos cómo implementar esta interfaz y trabajar con eventos clave.
Clase KeyEvent
Siempre que se presiona una tecla del teclado, un objeto de la clase KeyEvent
notifica al KeyListener
. La clase KeyEvent
tiene algunos métodos que se utilizan para obtener más información sobre el evento clave. A continuación se resumen tres de los métodos más importantes de esta clase.
- El método
getKeyChar()
obtiene el carácter clave asociado con el evento. Este método es muy beneficioso si queremos agregar alguna funcionalidad a una clave en particular. Por ejemplo, si se presionae
, la aplicación debería salir. - El método
getKeyCode()
es muy similar al métodogetKeyChar()
. Devuelve un código de tecla entero asociado con la tecla presionada. - El método
isActionKey()
puede indicar si se presiona una tecla de acción (como Bloq Mayús). Devuelve un booleano verdadero o falso.
Implementación de la interfaz KeyListener
La interfaz KeyListener escucha eventos clave y realiza alguna acción. La declaración de esta interfaz se muestra a continuación.
public interface KeyListener extends EventListener
Necesitamos anular los siguientes tres métodos de esta interfaz en nuestra clase.
- El método
keyPressed(KeyEvent e)
se invocará cuando se presione una tecla. - El método
keyReleased(KeyEvent e)
se invocará cuando se suelte la tecla. - El método
keyTyped(KeyEvent e)
se invocará cuando una tecla escriba un carácter.
También usaremos el método addKeyListener()
. Necesitamos pasar un objeto de la clase que implementa la interfaz KeyListener
a este método. Es una forma de registrar el objeto para escuchar y reaccionar ante eventos clave.
Una aplicación simple de KeyListener
Creemos una aplicación simple que escuche eventos clave e imprima algo en la consola. Crearemos un marco y le agregaremos una etiqueta. Nuestro programa debería imprimir el carácter clave y la acción clave en la consola. Si la tecla presionada es una tecla de acción, entonces el programa debería terminar.
import java.awt.FlowLayout;
import java.awt.Frame;
import java.awt.Label;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
public class KeyListenerExample implements KeyListener {
@Override
public void keyTyped(KeyEvent e) {
System.out.println("The key Typed was: " + e.getKeyChar());
}
@Override
public void keyPressed(KeyEvent e) {
if (e.isActionKey())
System.exit(0);
System.out.println("The key Pressed was: " + e.getKeyChar());
}
@Override
public void keyReleased(KeyEvent e) {
System.out.println("The key Released was: " + e.getKeyChar());
}
public static void main(String[] args) {
// Setting the Frame and Labels
Frame f = new Frame("Demo");
f.setLayout(new FlowLayout());
f.setSize(500, 500);
Label l = new Label();
l.setText("This is a demonstration");
f.add(l);
f.setVisible(true);
// Creating and adding the key listener
KeyListenerExample k = new KeyListenerExample();
f.addKeyListener(k);
}
}
Producción :
The key Pressed was: a
The key Typed was: a
The key Released was: a
The key Pressed was: b
The key Typed was: b
The key Released was: b
Una aplicación de juego simple usando KeyListener
Creemos otro programa que tomará las teclas de flecha como entrada. Este programa moverá el número 0 a diferentes ubicaciones en el array de acuerdo con la tecla presionada. La salida se imprime en la consola.
import java.awt.FlowLayout;
import java.awt.Frame;
import java.awt.Label;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.Arrays;
public class KeyListenerExample implements KeyListener {
// Matrix and x, y coordinates of 0
int[][] matrix;
int x;
int y;
KeyListenerExample() {
// Initializing the Matrix
matrix = new int[3][3];
matrix[0] = new int[] {1, 1, 1};
matrix[1] = new int[] {1, 0, 1};
matrix[2] = new int[] {1, 1, 1};
x = 1;
y = 1;
// Printing the Matrix
for (int i = 0; i < 3; i++) System.out.println(Arrays.toString(matrix[i]));
System.out.println();
}
// keyPressed() method takes care of moving the zero according to the key pressed
@Override
public void keyPressed(KeyEvent e) {
if (e.getKeyCode() == KeyEvent.VK_RIGHT) {
if (x != 2) {
x += 1;
System.out.println("Moving Right");
} else
System.out.println("Cannot Move Right");
}
if (e.getKeyCode() == KeyEvent.VK_LEFT) {
if (x != 0) {
x -= 1;
System.out.println("Moving Left");
} else
System.out.println("Cannot Move Left");
}
if (e.getKeyCode() == KeyEvent.VK_DOWN) {
if (y != 2) {
y += 1;
System.out.println("Moving Down");
} else
System.out.println("Cannot Move Down");
}
if (e.getKeyCode() == KeyEvent.VK_UP) {
if (y != 0) {
y -= 1;
System.out.println("Moving Up");
} else
System.out.println("Cannot Move Up");
}
matrix[0] = new int[] {1, 1, 1};
matrix[1] = new int[] {1, 1, 1};
matrix[2] = new int[] {1, 1, 1};
matrix[y][x] = 0;
for (int i = 0; i < 3; i++) System.out.println(Arrays.toString(matrix[i]));
System.out.println();
}
// We don't need the other two methods
@Override
public void keyReleased(KeyEvent e) {}
@Override
public void keyTyped(KeyEvent e) {}
public static void main(String[] args) {
// Setting the frame and labels
Frame f = new Frame("Demo");
f.setLayout(new FlowLayout());
f.setSize(200, 200);
Label l = new Label();
l.setText("This is a Game");
f.add(l);
f.setVisible(true);
// Creating and adding the key listener
KeyListenerExample k = new KeyListenerExample();
f.addKeyListener(k);
}
}
Producción :
[1, 1, 1]
[1, 0, 1]
[1, 1, 1]
Moving Right
[1, 1, 1]
[1, 1, 0]
[1, 1, 1]
Cannot Move Right
[1, 1, 1]
[1, 1, 0]
[1, 1, 1]
Moving Left
[1, 1, 1]
[1, 0, 1]
[1, 1, 1]
Moving Left
[1, 1, 1]
[0, 1, 1]
[1, 1, 1]
Cannot Move Left
[1, 1, 1]
[0, 1, 1]
[1, 1, 1]
Moving Up
[0, 1, 1]
[1, 1, 1]
[1, 1, 1]
Cannot Move Up
[0, 1, 1]
[1, 1, 1]
[1, 1, 1]
Moving Down
[1, 1, 1]
[0, 1, 1]
[1, 1, 1]
Moving Down
[1, 1, 1]
[1, 1, 1]
[0, 1, 1]
Cannot Move Down
[1, 1, 1]
[1, 1, 1]
[0, 1, 1]
Resumen
Las clases implementan la interfaz KeyListener
para escuchar y reaccionar ante eventos clave. En este tutorial, aprendimos algunos métodos importantes de la clase KeyEvent
. También aprendimos cómo implementar la interfaz KeyListener y cómo anular los métodos keyPressed()
, keyReleased()
y keyTyped()
. También vimos algunos ejemplos que demostraron el uso de esta interfaz.