Convertir Iterable a Stream en Java
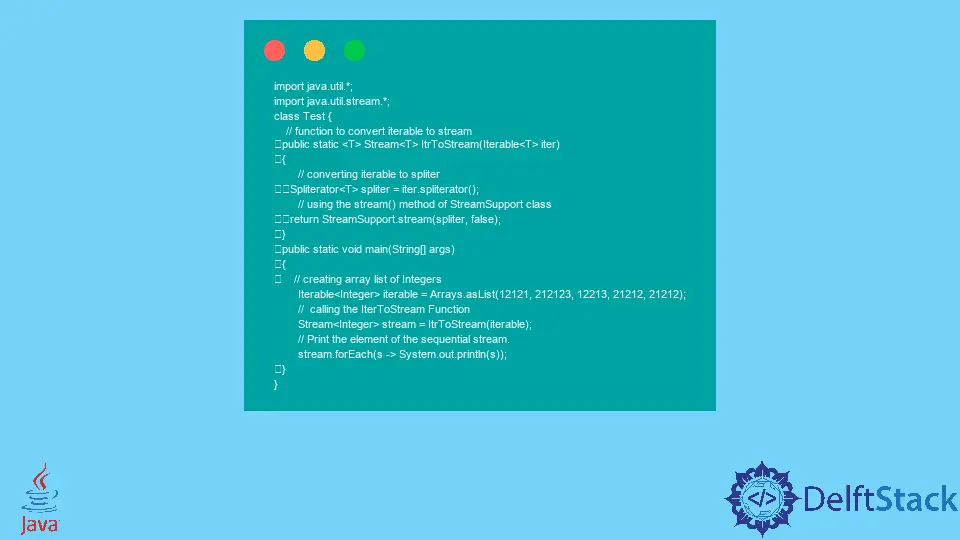
En Java, podemos usar el Iterador
para iterar a través de cada elemento de la colección. La secuencia es una canalización de objetos de colecciones.
Puede haber dos tipos de flujos. Uno es paralelo y otro es un flujo secuencial.
En este artículo, aprenderemos un método para convertir el Iterable
en Stream
.
Utilice el método StreamSupport.stream()
para convertir Iterable
a Stream
en Java
Ningún método en la interfaz iterable
puede convertir directamente iterable
en stream
. Entonces, debemos usar el método stream()
de la clase StreamSupport
.
Los usuarios pueden seguir la siguiente sintaxis para usar el método stream()
.
Spliterator<T> spliter = iter.spliterator();
return StreamSupport.stream(spliter, isParallel);
Parámetros:
spliter |
Es un divisor del iterador, que hemos convertido mediante el método Iterable.spliterator() . |
isParallel |
Un valor booleano define si la transmisión es paralela o secuencial. Los usuarios deben pasar el valor booleano true para obtener el flujo paralelo. |
En el siguiente ejemplo, hemos creado el iterable de enteros. Llamamos a la función IterToStream()
y pasamos el iterable como un argumento que devuelve el flujo de un iterable en particular.
En la función ItrToStream()
, estamos convirtiendo el iterable en spliterator
. Después de eso, usamos el método StreamSupport.stream()
para convertir el divisor
en flujo.
Por fin, estamos imprimiendo la transmisión.
import java.util.*;
import java.util.stream.*;
class Test {
// function to convert iterable to stream
public static <T> Stream<T> ItrToStream(Iterable<T> iter) {
// converting iterable to spliter
Spliterator<T> spliter = iter.spliterator();
// using the stream() method of StreamSupport class
return StreamSupport.stream(spliter, false);
}
public static void main(String[] args) {
// creating array list of Integers
Iterable<Integer> iterable = Arrays.asList(12121, 212123, 12213, 21212, 21212);
// calling the IterToStream Function
Stream<Integer> stream = ItrToStream(iterable);
// Print the element of the sequential stream.
stream.forEach(s -> System.out.println(s));
}
}
Producción :
12121
212123
12213
21212
21212
Hemos aprendido con éxito a convertir iterable
a stream
en Java usando el método StreamSupport.stream()
.