Erstellen Sie einen Pandas-Datenrahmen aus einer Wörterbuchliste
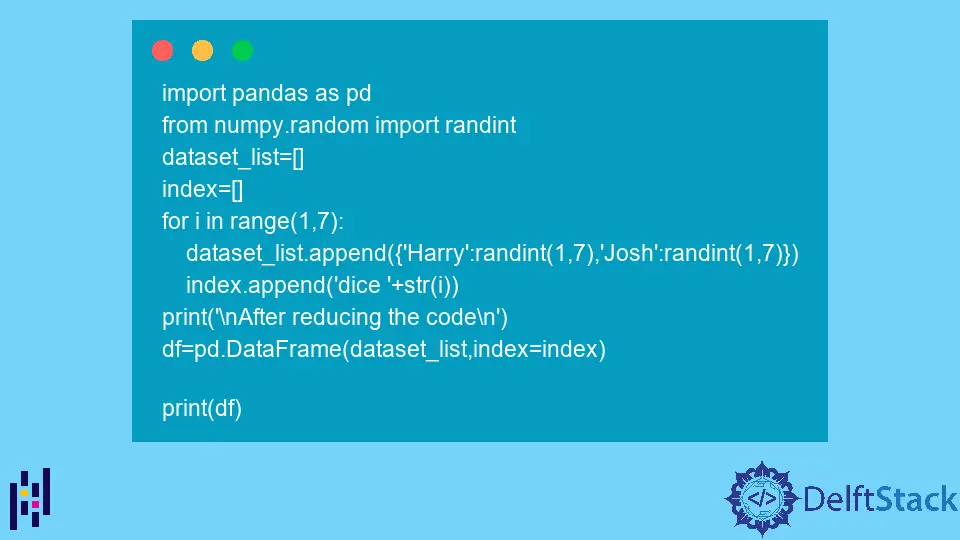
Das Dictionary ist ein kompakter und flexibler Python-Container, der separate Key-Value-Maps speichert. Wörterbücher werden in geschweiften Klammern ({}
) geschrieben, die durch Kommas getrennte Schlüsselwortpaare enthalten, (,)
und :
trennen jeden Schlüssel von seinem Wert.
Nachfolgend sind drei Wörterbücher aufgeführt, die ein Beispiel für ein Würfelspiel enthalten.
Nehmen wir ein Beispiel aus einem Würfelspiel. In diesem Fall würfeln zwei Spieler mit ihren sechs Würfeln und speichern Würfel mit entsprechenden Spielern.
import pandas as pd
from numpy.random import randint
# create datset from multiple dictionaries
dataset_list = [
{"Harry": 1, "Josh": 3, "dices": "first dice"},
{"Harry": 5, "Josh": 1, "dices": "second dice"},
{"Harry": 6, "Josh": 2, "dices": "third dice"},
{"Harry": 2, "Josh": 3, "dices": "fourth dice"},
{"Harry": 6, "Josh": 6, "dices": "fifth dice"},
{"Harry": 4, "Josh": 3, "dices": "sixth dice"},
]
df = pd.DataFrame(dataset_list)
print(df)
print()
harry = []
josh = []
for i in range(6):
harry.append(randint(1, 7))
josh.append(randint(1, 7))
Wir haben einen Datensatz aus einer Liste von Elementen erstellt, die ein Dictionary enthalten, da wir wissen, dass der Datenrahmen
ein Schlüssel-Wert-Paar verwendet. Deshalb ist dies mit einem Dictionary angebracht.
Ausgabe:
Harry Josh dices
0 1 3 first dice
1 5 1 second dice
2 6 2 third dice
3 2 3 fourth dice
4 6 6 fifth dice
5 4 3 sixth dice
Im letzten Beispiel setzen wir die Würfel manuell; jetzt verwenden wir eine randint
-Methode, die in der numpy
-Bibliothek definiert ist. Wir haben zwei leere Listen mit den Namen harry
und josh
in der folgenden Zeile erstellt. Als nächstes haben wir eine for
-Schleife erstellt, dieser Bereich ist definiert von 0-6, wobei Zufallszahlen in zwei definierten Listen als Element mit der append()
-Methode angehängt werden, siehe unten.
import pandas as pd
from numpy.random import randint
print()
harry = []
josh = []
for i in range(6):
harry.append(randint(1, 7))
josh.append(randint(1, 7))
# create datset from multiple dictionaries
dataset_list = [
{"Harry": harry[0], "Josh": josh[0], "dices": "first dice"},
{"Harry": harry[1], "Josh": josh[1], "dices": "second dice"},
{"Harry": harry[2], "Josh": josh[2], "dices": "third dice"},
{"Harry": harry[3], "Josh": josh[3], "dices": "fourth dice"},
{"Harry": harry[4], "Josh": josh[4], "dices": "fifth dice"},
{"Harry": harry[5], "Josh": josh[5], "dices": "sixth dice"},
]
df = pd.DataFrame(dataset_list)
print(df)
Denken Sie daran, dass randint()
den Bereich von gegeben eins bis n-1
oder standardmäßig null bis n-1
nimmt, deshalb haben wir den Bereich von 1-7
definiert.
Ausgang
Harry Josh dices
0 4 1 first dice
1 4 2 second dice
2 3 4 third dice
3 1 1 fourth dice
4 4 5 fifth dice
5 4 4 sixth dice
Nun haben wir mit Hilfe der for
-Schleife Codezeilen reduziert und das ganze Dictionary in einer Liste angehängt, weitere Indizes in der Liste namens index
anhängen, entsprechen den Spielerzügen und als Index in DataFrame
gesetzt.
import pandas as pd
from numpy.random import randint
dataset_list = []
index = []
for i in range(1, 7):
dataset_list.append({"Harry": randint(1, 7), "Josh": randint(1, 7)})
index.append("dice " + str(i))
print("\nAfter reducing the code\n")
df = pd.DataFrame(dataset_list, index=index)
print(df)
Ausgang:
Harry Josh
dice 1 2 4
dice 2 2 3
dice 3 6 5
dice 4 5 2
dice 5 4 2
dice 6 1 1
Alle Beispiele:
import pandas as pd
from numpy.random import randint
# create datset from multiple dictionaries
dataset_list = [
{"Harry": 1, "Josh": 3, "dices": "first dice"},
{"Harry": 5, "Josh": 1, "dices": "second dice"},
{"Harry": 6, "Josh": 2, "dices": "third dice"},
{"Harry": 2, "Josh": 3, "dices": "fourth dice"},
{"Harry": 6, "Josh": 6, "dices": "fifth dice"},
{"Harry": 4, "Josh": 3, "dices": "sixth dice"},
]
df = pd.DataFrame(dataset_list)
print(df)
print()
harry = []
josh = []
for i in range(6):
harry.append(randint(1, 7))
josh.append(randint(1, 7))
# create datset from multiple dictionaries
dataset_list = [
{"Harry": harry[0], "Josh": josh[0], "dices": "first dice"},
{"Harry": harry[1], "Josh": josh[1], "dices": "second dice"},
{"Harry": harry[2], "Josh": josh[2], "dices": "third dice"},
{"Harry": harry[3], "Josh": josh[3], "dices": "fourth dice"},
{"Harry": harry[4], "Josh": josh[4], "dices": "fifth dice"},
{"Harry": harry[5], "Josh": josh[5], "dices": "sixth dice"},
]
df = pd.DataFrame(dataset_list)
print(df)
dataset_list = []
index = []
for i in range(1, 7):
dataset_list.append({"Harry": randint(1, 7), "Josh": randint(1, 7)})
index.append("dice " + str(i))
print("\nAfter reducing the code\n")
df = pd.DataFrame(dataset_list, index=index)
print(df)
Ausgang:
Harry Josh dices
0 1 3 first dice
1 5 1 second dice
2 6 2 third dice
3 2 3 fourth dice
4 6 6 fifth dice
5 4 3 sixth dice
Harry Josh dices
0 4 1 first dice
1 4 2 second dice
2 3 4 third dice
3 1 1 fourth dice
4 4 5 fifth dice
5 4 4 sixth dice
After reducing the code
Harry Josh
dice 1 2 4
dice 2 2 3
dice 3 6 5
dice 4 5 2
dice 5 4 2
dice 6 1 1