console.log() in React
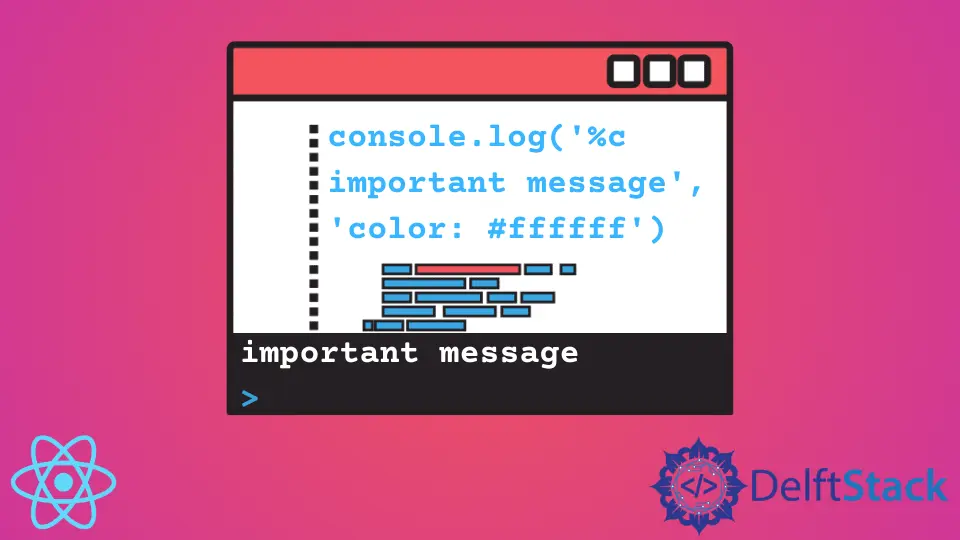
console.log()
is developers’ go-to method for debugging their code. It is used to log JavaScript values on the console. React does not restrict the use of the console.log()
method, but because of its special JSX syntax, there are rules that need to be followed.
React console
Object
console
object comes with an interface that contains dozens of debugging methods. You can find the exact list of methods on the official MDN page. This article will focus on the most popular method - console.log()
and look into how to make the best use of it.
Logging in React
There are numerous ways to console.log()
a value in React. If you want to continue logging a value every time the component re-renders, you should put the console.log()
method under render()
call. Here’s what this implementation would look like.
class App extends Component {
constructor(props){
super(props)
this.state = {
input: ""
}
}
render() {
console.log('I log every time the state is updated');
return <input type="text" onChange={(e) => this.setState({input: e.target.value})}></input>;
}
}
Every time we enter a new value into the text <input>
element, the state will change, which will trigger the component to re-render. Every time it does, it will log the specified text to the console.
If you want to console.log()
a value only once, you can use lifecycle methods of React class components. Here’s what the actual code would look like:
class App extends Component {
constructor(props){
super(props)
this.state = {
input: ""
}
}
componentDidMount(){
console.log('I log only once');
}
render() {
return <input type="text" onChange={(e) => this.setState({input: e.target.value})}></input>;
}
}
Once you debug your app, make sure to delete all instances of console.log()
before exiting the development mode. console.log()
statements add nothing to the production mode, so they should be removed.
Customization
React developers can customize the style of the regular console.log()
method. For instance, if you want to create a special message for tasks that require attention, you can create a custom console.attention
method based on the core functionality of console.log()
.
If you add this definition anywhere in your app and call the console.important()
method with the message
argument, you’ll see that your console will have a colored message.
console.important = function(text) {
console.log('%c important message', 'color: #913831')
}
Here’s an example of colored console message:
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn