React 中的 console.log()
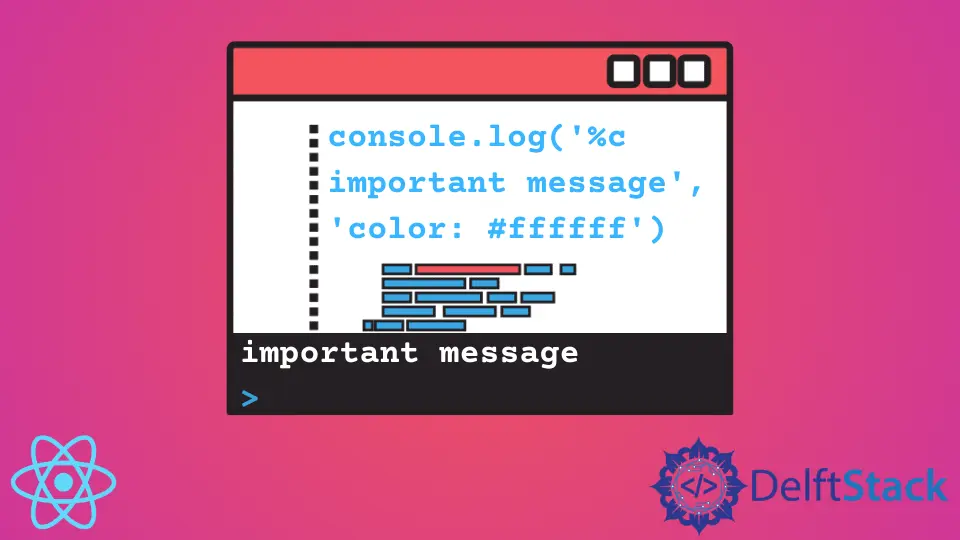
console.log()
是开发人员调试代码的首选方法。它用于在控制台上记录 JavaScript 值。React 不限制使用 console.log()
方法,但由于其特殊的 JSX 语法,有一些规则需要遵守。
控制台
对象
console
对象带有一个包含数十种调试方法的接口。你可以在 official MDN 页面上找到确切的方法列表。本文将重点介绍最流行的方法 - console.log()
,并探讨如何充分利用它。
登录 React
在 React 中,有很多方法可以 console.log()
一个值。如果你想在每次组件重新渲染时继续记录一个值,你应该将 console.log()
方法放在 render()
调用下。下面是这个实现的样子。
class App extends Component {
constructor(props){
super(props)
this.state = {
input: ""
}
}
render() {
console.log('I log every time the state is updated');
return <input type="text" onChange={(e) => this.setState({input: e.target.value})}></input>;
}
}
每次我们在文本 <input>
元素中输入一个新值时,状态都会改变,这将触发组件重新渲染。每次这样做时,它都会将指定的文本记录到控制台。
如果你只想 console.log()
一个值一次,你可以使用 React 类组件的生命周期方法。下面是实际代码的样子:
class App extends Component {
constructor(props){
super(props)
this.state = {
input: ""
}
}
componentDidMount(){
console.log('I log only once');
}
render() {
return <input type="text" onChange={(e) => this.setState({input: e.target.value})}></input>;
}
}
调试应用程序后,请确保在退出开发模式之前删除 console.log()
的所有实例。console.log()
语句不会向生产模式添加任何内容,因此应将其删除。
定制
React 开发人员可以自定义常规 console.log()
方法的样式。例如,如果你想为需要注意的任务创建一条特殊的消息,你可以基于 console.log()
的核心功能创建一个自定义的 console.attention
方法。
如果你在应用程序的任何位置添加此定义并使用 message 参数调用 console.important()
方法,你将看到你的控制台将显示一条彩色消息。
console.important = function(text) {
console.log('%c important message', 'color: #913831')
}
这是彩色控制台消息的示例:
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn