How to Convert Stream Element to Map in Java
- Stream of Strings to Map in Java
- Create Map From the Objects of Stream in Java
- Determine Values Length While Converting Stream to Map in Java
- Convert Stream to Map for Unique Product Keys in Java
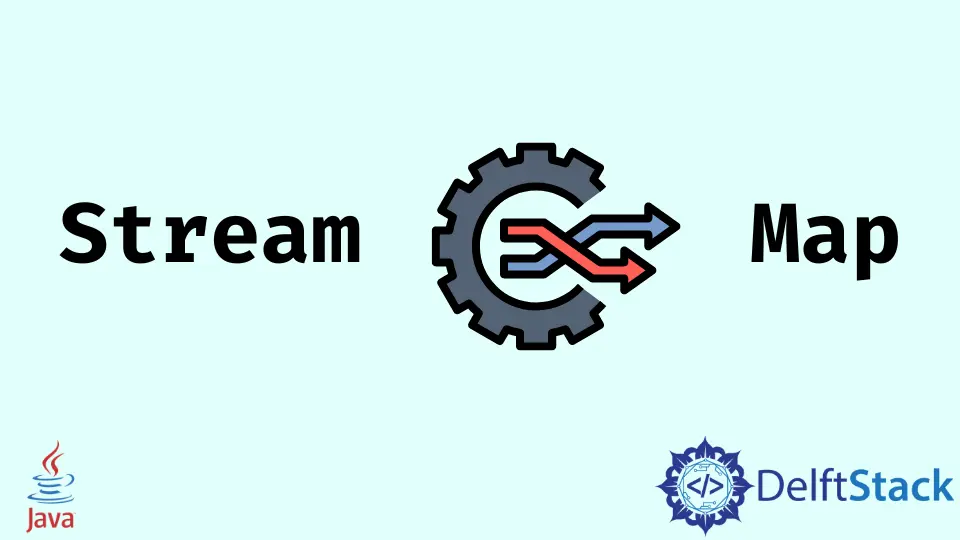
We will go over the practical uses of Java Streams
and how to convert stream elements into map elements.
To understand this article, you must have a basic understanding of Java 8, particularly lambda expressions and the Stream
API. But, if you are a newbie, do not worry; we will explain everything.
Stream of Strings to Map in Java
You must be wondering that the string is fundamentally a list of elements, not a list of either keys or values, aren’t you? To convert a string stream to a map, you will have to understand the basics of the following methods.
Collectors.toMap()
- It performs different functional reduction operations, such as collecting elements into collections.toMap
- It returns aCollector
that gathers elements into aMap
with keys and values.
Map<String, String> GMS = stream.collect(Collectors.toMap(k -> k[0], k -> k[1]));
Got it? We will specify appropriate mapping functions to define how to retrieve keys and values from stream elements.
Implementation of Example1.java
:
package delftstackStreamToMapJava;
import java.util.Map;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class Example1 {
// Method to get stream of `String[]`
private static Stream<String[]> MapStringsStream() {
return Stream.of(new String[][] {{"Sky", "Earth"}, {"Fire", "Water"}, {"White", "Black"},
{"Ocean", "Deep"}, {"Life", "Death"}, {"Love", "Fear"}});
}
// Program to convert the stream to a map in Java 8 and above
public static void main(String[] args) {
// get a stream of `String[]`
Stream<String[]> stream = MapStringsStream();
// construct a new map from the stream
Map<String, String> GMS = stream.collect(Collectors.toMap(k -> k[0], k -> k[1]));
System.out.println(GMS);
}
}
Output:
{Sky=Earth, White=Black, Love=Fear, Fire=Water, Life=Death, Ocean=Deep}
Create Map From the Objects of Stream in Java
This program is another simple demonstration of using the Collectors.toMap()
method.
Besides, it also uses references function/lambda expression. So, we recommend you check it for a few minutes.
Also, read Function.identity()
if you have not used it before.
-
Check out our three streams:
List<Double> L1 = Arrays.asList(1.1, 2.3); List<String> L2 = Arrays.asList("A", "B", "C"); List<Integer> L3 = Arrays.asList(10, 20, 30);
We defined three array lists as streams with different data types in each.
-
To add more to our primary mapping method:
2.1 It takes a key and value mapper to generate aMap
fromStream
objects.
2.2Stream
, in this case, is our array lists, which are then passed toCollectors
for reduction while getting mapped in keys with the help of.toMap
.Map<Double, Object> printL1 = L1.stream().collect(Collectors.toMap(Function.identity(), String::valueOf, (k1, k2) -> k1)); System.out.println("Stream of Double to Map: " + printL1);
Implementation of Example2.java
:
package delftstackStreamToMapJava;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.function.Function;
import java.util.stream.Collectors;
public class Example2 {
public static void main(String[] args) {
// Stream of Integers
List<Double> L1 = Arrays.asList(1.1, 2.3);
List<String> L2 = Arrays.asList("A", "B", "C");
List<Integer> L3 = Arrays.asList(10, 20, 30);
Map<Double, Object> printL1 =
L1.stream().collect(Collectors.toMap(Function.identity(), String::valueOf, (k1, k2) -> k1));
System.out.println("Stream of Double to Map: " + printL1);
Map<String, Object> printL2 =
L2.stream().collect(Collectors.toMap(Function.identity(), String::valueOf, (k1, k2) -> k1));
System.out.println("Stream of String to Map: " + printL2);
Map<Integer, Object> printL3 =
L3.stream().collect(Collectors.toMap(Function.identity(), String::valueOf, (k1, k2) -> k1));
System.out.println("Stream of Integers to Map: " + printL3);
}
}
Output:
Stream of Double to Map: {2.3=2.3, 1.1=1.1}
Stream of String to Map: {A=A, B=B, C=C}
Stream of Integers to Map: {20=20, 10=10, 30=30}
Determine Values Length While Converting Stream to Map in Java
The following code block converts a string into a Map
, with the keys representing the string value and the value indicating the length of each word. This is our final example, although this one falls into the category of an elementary stream to map elementary examples.
Explanation:
The Collectors.toMap
collector accepts two lambda functions as parameters.
stream-> stream
- determines theStream
value and returns it as the map’s key.stream-> stream.length
- finds the present stream value, finds its length and returns it to the map for the given key.
Implementation of Example3.java
:
package delftstackStreamToMapJava;
import java.util.Arrays;
import java.util.Map;
import java.util.stream.Collectors;
public class Example3 {
public static Map<String, Integer> toMap(String str) {
Map<String, Integer> elementL =
Arrays.stream(str.split("/"))
.collect(Collectors.toMap(stream -> stream, stream -> stream.length()));
return elementL;
}
public static void main(String[] args) {
String stream = "We/Will/Convert/Stream/Elements/To/Map";
System.out.println(toMap(stream));
}
}
Output:
{Convert=7, Stream=6, Will=4, To=2, Elements=8, Map=3, We=2}
Convert Stream to Map for Unique Product Keys in Java
We will convert a stream of integer pro_id
and string’s productname
. Not to get confused, though!
This is yet another implementation of a collection of streams to map values.
It is sequential, and the keys here are unique pro_id
. The whole program is the same as an example two except for its concept.
This is the somewhat more realistic situation of using streams to map. It will also enable your understanding of how many ways you can construct a customized program that extracts unique values and keys from array objects.
Implementation of Products.java
:
package delftstackStreamToMapJava;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.function.Function;
import java.util.stream.Collectors;
public class Products {
private Integer pro_id;
private String productname;
public Products(Integer pro_id, String productname) {
this.pro_id = pro_id;
this.productname = productname;
}
public Integer extractpro_id() {
return pro_id;
}
public String getproductname() {
return productname;
}
public String toString() {
return "[productid = " + this.extractpro_id() + ", productname = " + this.getproductname()
+ "]";
}
public static void main(String args[]) {
List<Products> listofproducts = Arrays.asList(new Products(1, "Lamda 2.0 "),
new Products(2, "Gerrio 3A ultra"), new Products(3, "Maxia Pro"),
new Products(4, "Lemna A32"), new Products(5, "Xoxo Pro"));
Map<Integer, Products> map = listofproducts.stream().collect(
Collectors.toMap(Products::extractpro_id, Function.identity()));
map.forEach((key, value) -> { System.out.println(value); });
}
}
Output:
[productid = 1, productname = Lamda 2.0 ]
[productid = 2, productname = Gerrio 3A ultra]
[productid = 3, productname = Maxia Pro]
[productid = 4, productname = Lemna A32]
[productid = 5, productname = Xoxo Pro]
IllegalArgumentException
.Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedInRelated Article - Java Map
- Increment Map in Java
- How to Convert Map Values Into a List in Java
- How to Convert List to Map in Java
- How to Filter A Value From Map in Java
- How to Create Map in Java