Map 函数
Jinku Hu
2020年6月25日
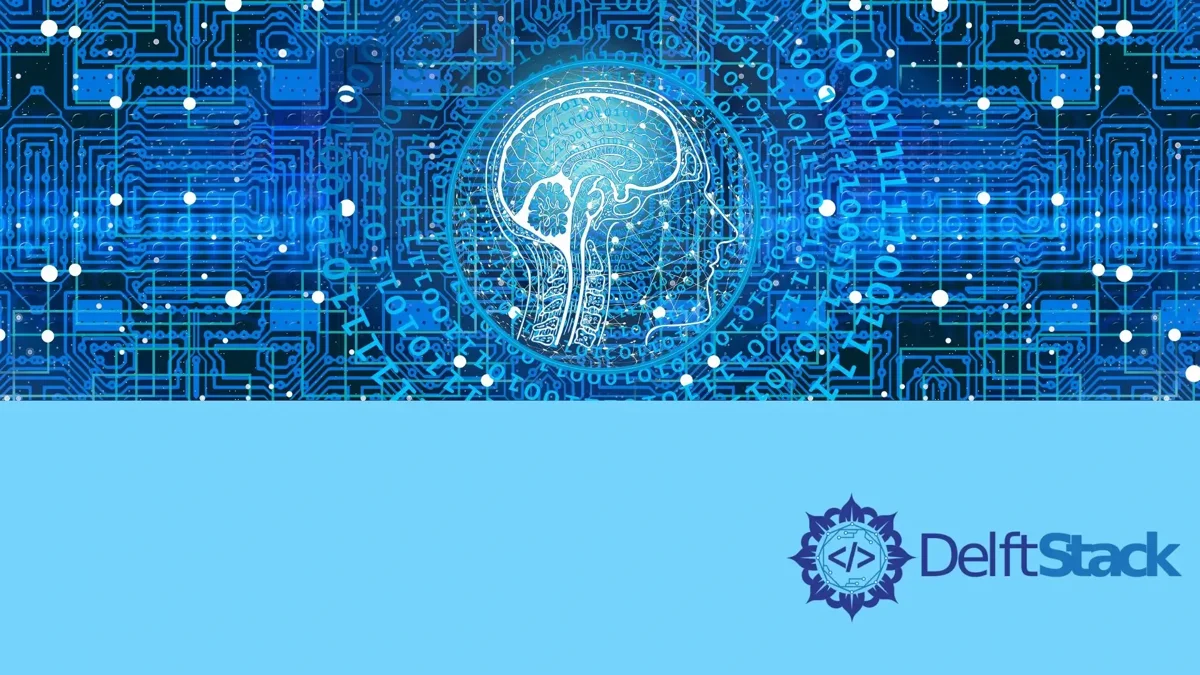
map
函数是 Python 函数式编程的内置函数中最简单的函数。map()
将指定的函数应用于可迭代序列中的每个元素:
names = ["Fred", "Wilma", "Barney"]
Python 3.x3.0
map(len, names) # map in Python 3.x is a class; its instances are iterable
# Out: <map object at 0x00000198B32E2CF8>
它的具体意思就是将 len()
函数应用在列表中的每个元素,但返回一个 map
对象实例,你可以用 __next__()
函数逐一将每个结果取出。
在 Python 2.x 中的 future_builtins
模块中引入了跟 Python3 兼容的 map
函数
Python 2.x2.6
from future_builtins import map # contains a Python 3.x compatible map()
map(len, names) # see below
# Out: <itertools.imap instance at 0x3eb0a20>
或者,在 Python 2 中,可以使用 itertools
中的 imap
来获取生成器
Python 2.x2.3
from itertools import imap
map(len, names) # map() returns a list
# Out: [4, 5, 6]
imap(len, names) # itertools.imap() returns a generator
# Out: <itertools.imap at 0x405ea20>
结果可以显式转换为一个列表以消除 Python 2 和 3 之间的差异:
list(map(len, names))
# Out: [4, 5, 6]
map()
可以用等效的列表推导式或生成器表达式来替换:
[len(item) for item in names] # equivalent to Python 2.x map()
# Out: [4, 5, 6]
(len(item) for item in names) # equivalent to Python 3.x map()
# Out: <generator object <genexpr> at 0x00000195888D5FC0>
map
函数具体实例
你可以获取每个元素的绝对值:
list(map(abs, (1, -1, 2, -2, 3, -3))) # the call to `list` is unnecessary in 2.x
# Out: [1, 1, 2, 2, 3, 3]
map
也支持对列表的匿名函数操作,
map(lambda x: x * 2, [1, 2, 3, 4, 5])
# Out: [2, 4, 6, 8, 10]
我们也可以用自定义的函数,比如将十进制值转换为百分比:
def to_percent(num):
return num * 100
list(map(to_percent, [0.95, 0.75, 1.01, 0.1]))
# Out: [95.0, 75.0, 101.0, 10.0]
或将美元兑换成欧元(给定汇率):
from functools import partial
from operator import mul
rate = 0.9 # fictitious exchange rate, 1 dollar = 0.9 euros
dollars = {"hidden somewhere": 1000, "jeans": 45, "bank": 5000}
# Below it gives the values in Euro
sum(map(partial(mul, rate), dollars.values()))
# Out: 5440.5
functools.partial
是一种方便的方法来给出函数的参数,以便它们可以使用 map
而不是使用 lambda
或创建自定义函数。