在 Scala 中拆分字符串
Suraj P
2022年5月18日
Scala
Scala String
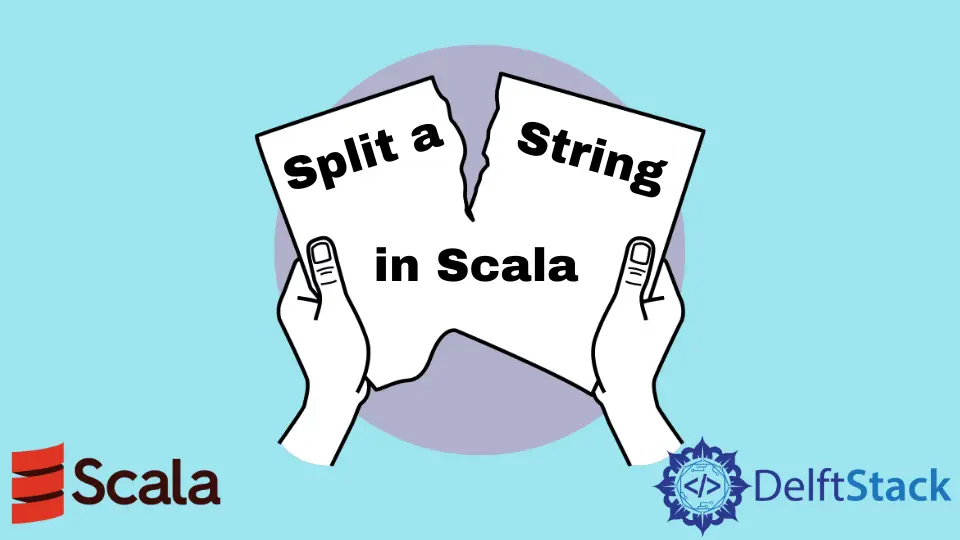
本文将介绍 Scala 编程语言中拆分字符串的方法。
在 Scala 中使用 split()
方法拆分字符串
Scala 提供了一个名为 split()
的方法,用于使用作为参数传递的 delimiter
将给定字符串拆分为字符串数组。
这是可选的,但我们也可以使用 limit
参数来限制结果数组的元素总数。
让我们看一个字符串中只有一个分隔符的示例。
语法:
val resultant_array = string_Name.split(delimiter, limit(optional))
案例 1:
object MyClass
{
def main(args: Array[String])
{
val line = "brick,cement,concrete"
// Split on each comma.
val arr = line.split(',')
arr.foreach(println) //printing the resultant array
}
}
输出:
brick
cement
concrete
现在,让我们看一个字符串中有多个分隔符的情况。在 split 方法中,我们传递数组中的所有分隔符。
拆分处理分隔符。然后,在数组中返回按字母顺序排列的字符串。
语法:
val resultant_array = string_Name.split(Array_containing_delimiters, limit(optional))
案例 2:
object MyClass
{
def main(args: Array[String])
{
val codes = "abc;def,ghi:jkl"
val arr = codes.split(Array(';', ',', ':')) //all the delimiters are passed in an array
arr.foreach(println)
}
}
输出:
abc
def
ghi
jkl
我们来看一个分隔符是字符串的情况;而不是单个字符,它是字符的集合。
语法:
val resultant_array = string_Name.split(string delimiter, limit(optional))
案例 3:
object MyClass {
def main(args: Array[String]) {
val codes = "Tony stark is the iron man he is the hero"
val arr = codes.split("the") //splitting at every "the"
arr.foreach(println)
}
}
输出:
Tony stark is
iron man he is
hero
此外,我们可以使用 regex
正则表达式来分隔字符串。这是首选方法,因为我们可以编写一个可以同时处理多个案例的 regex
。
案例 4:
object MyClass {
def main(args: Array[String]) {
val items = "scala; ; python; c++"
// Any number of spaces or semicolons is a delimiter.
val arr = items.split("[; ]+")
//we can see that no empty element or whitespace is printed as regex handles it perfectly
arr.foreach(println)
}
}
输出:
scala
python
c++
items
中分隔符之间的空字符串在上面的代码中被忽略。然后,将两个周围的定界符组合起来。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Suraj P