Scala 中 asInstanceOf 和(O:T) 的区别
Suraj P
2023年1月30日
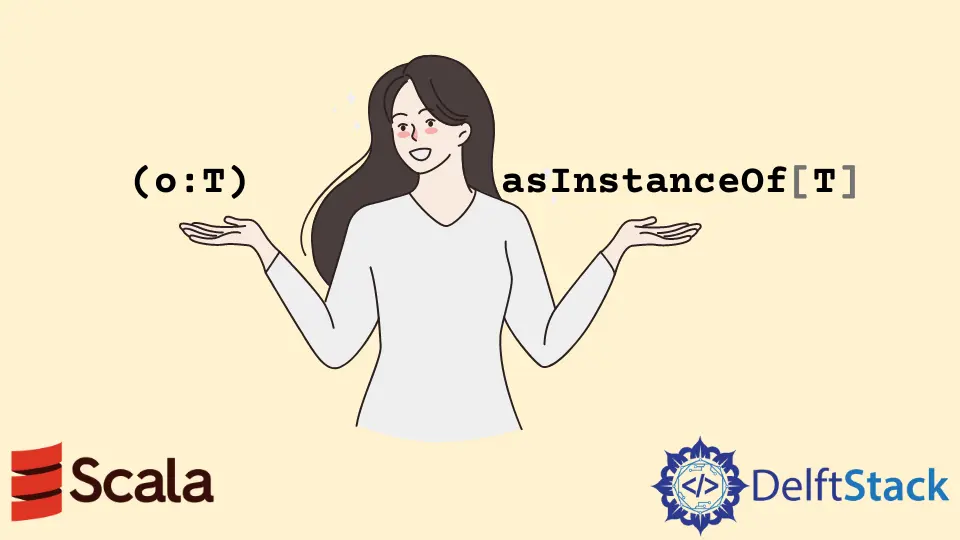
本文将学习 Scala 中的 asInstanceOf[T]
和 (o:T)
之间的区别。
在 Scala 中使用 asInstanceOf[T]
在 Scala 中,asInstanceOf[T]
用于类型转换或将对象的类型转换为另一种类型,例如将 int
转换为 float
。由于这完全是一个运行时操作,运行时错误是可能的。
Scala 中有两种类型转换。这些是隐式和显式类型转换。
编译器本身执行隐式类型转换。这种转换可能是有损的;在某些情况下可能会丢失数据。
例如,可以返回非整数值的两个 int
值的除法将属于 int
类型,从而导致数据丢失。
显式类型转换是用户定义的转换。值的类型转换可以直接完成,无需任何特殊方法。
但是,如果我们想对引用进行类型转换,我们必须使用 asInstanceOf
方法。
语法:对象的类型更改为 T
。
object.asInstanceof[T]
以下代码示例演示了使用 asInstanceOf
方法的显式类型转换。
示例 1:
object MyClass {
//the method takes input int type and outputs char type
def intTocharConverter(obj: Int): Char = {
return obj.asInstanceOf[Char]
}
def main(args: Array[String]):Unit= {
val obj = 100
println("The value of obj is: " + obj + ", type of value is " + obj.getClass)
val obj2 = intTocharConverter(obj) //ASCII value of 100 (d) is present in variable obj2
println("Value after casting is: " + obj2 + ", type of value is " + obj2.getClass)
}
}
输出:
The value of obj is: 100, type of value is int
Value after casting is: d, type of value is char
示例 2:
object MyClass {
//the method takes input int type and outputs double type
def intTodoubleConverter(obj: Int): Double = {
return obj.asInstanceOf[Double]
}
def main(args: Array[String]):Unit= {
val obj = 123
println("The value of obj is: " + obj + ", type of value is " + obj.getClass)
val obj2 = intTodoubleConverter(obj)
println("Value after casting is: " + obj2 + ", type of value is " + obj2.getClass)
}
}
输出:
The value of obj is: 123, type of value is int
Value after casting is: 123.0, type of value is double
在 Scala 中使用 (o:T)
为了类型检查器,这种类型归属是在编译时完成的。向上转换,也称为加宽,意味着我们将一个子类实例转换为它的一个超类。
由于这是一个编译时操作,我们可能会得到编译时错误,但不会发生运行时错误。这种类型归属也可以用于隐式转换。
下面是使用归属的隐式转换的代码示例。
object MyClass {
implicit def foo(s:String):Int = s.length
def main(args: Array[String]):Unit= {
val obj = "123"
println(foo(obj))
}
}
输出:
3
让我们以表格形式总结讨论。
asInstanceOf[T] |
(o:T) |
---|---|
用于引用的显式类型转换。 | 可用于隐式类型转换。 |
运行时操作。 | 编译时操作。 |
可能会发生运行时错误,例如 ClassCastException 。 |
编译时错误可能会像类型不匹配错误一样发生。 |