在 Ruby 中使用 begin 和 rescue 处理异常
Nurudeen Ibrahim
2023年1月30日
Ruby
Ruby Exception
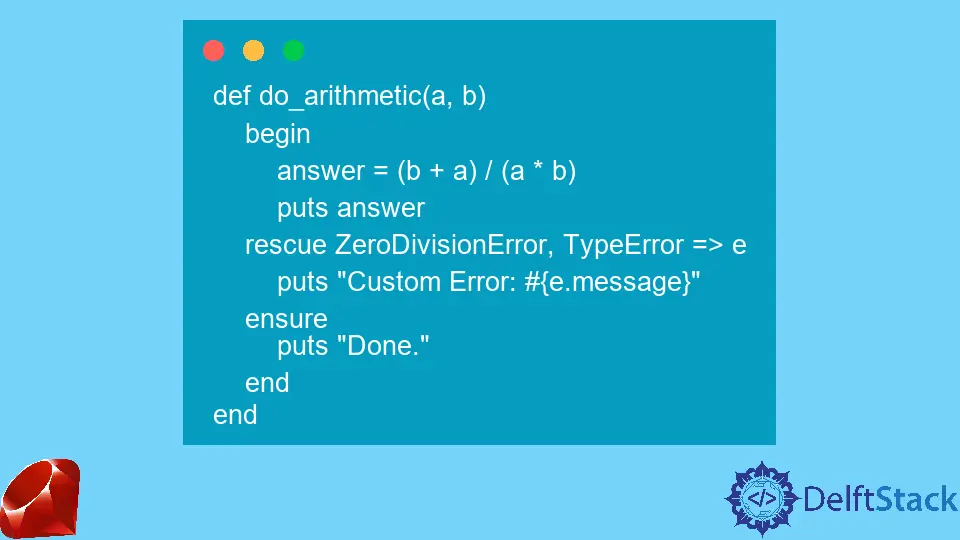
本文将讨论在 Ruby 中使用 begin
和 rescue
处理异常。
Ruby 中异常处理的一般语法
在 Ruby 中,begin
和 rescue
关键字通过将可能引发异常的代码包含在 begin-end 块中来处理异常。
begin
# code that might raise an exception
rescue AnExceptionClass => a_variable
# code that deals with some exception
rescue AnotherException => another_variable
# code that deals with another exception
else
# code that runs only if no exception was raised
ensure
# code that always runs no matter what
end
在 Ruby 中使用 begin
和 rescue
处理异常
下面的示例显示了 begin
和 rescue
关键字。
示例代码:
def do_arithmetic(a, b)
begin
answer = (b + a) / (a * b)
puts answer
rescue ZeroDivisionError => e
puts "Custom Error: #{e.message}"
rescue TypeError => e
puts "Custom Error: #{e.message}"
ensure
puts "Done."
end
end
do_arithmetic(2, 2)
do_arithmetic(0, 2)
do_arithmetic("David", 2)
输出:
# first output
1
Done.
# second output
Custom Error: divided by 0
Done.
# third output
Custom Error: String can't be coerced into Integer
Done.
组合多个异常并在同一个 rescue
块中处理它们也是可能的。上面示例中的 ZeroDivisionError,
和 TypeError
异常可以在下面一起运行。
def do_arithmetic(a, b)
begin
answer = (b + a) / (a * b)
puts answer
rescue ZeroDivisionError, TypeError => e
puts "Custom Error: #{e.message}"
ensure
puts "Done."
end
end
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe