在 Ruby 中解析 JSON 字符串
Nurudeen Ibrahim
2022年5月18日
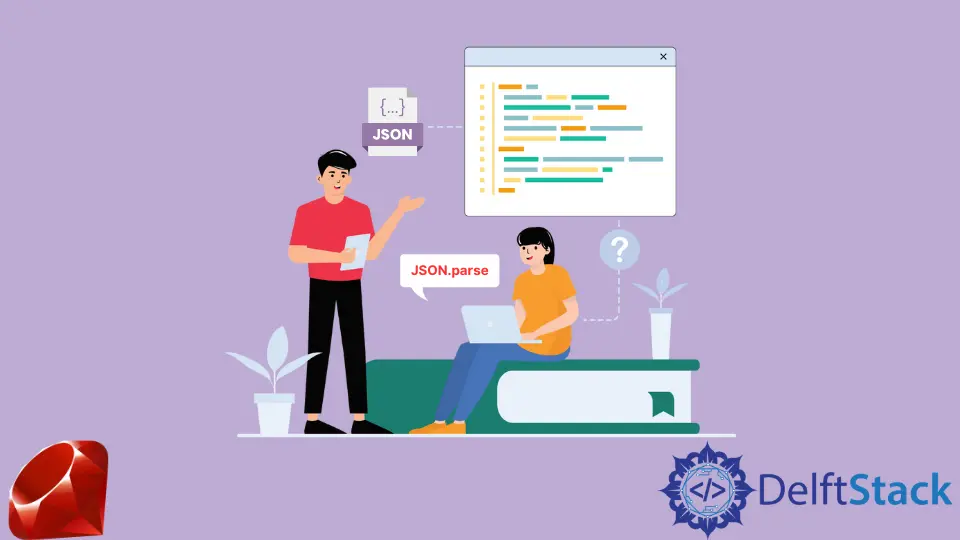
JSON(JavaScript Object Notation)是一种流行的数据交换格式,主要用于 Web 应用程序之间发送和接收数据。在本教程中,我们将研究如何解析 JSON 字符串。
JSON 值的常见示例可能是:
- 方括号括起来的值列表。例如
["Orange", "Apple", [1, 2]]
- 由花括号括起来的键/对的列表。例如
{ "name": "John", "sex": "male", "grades": [60, 70, 80, 90] }
这些 JSON 值有时以字符串形式出现,例如,作为来自外部 API 的响应,并且总是需要将它们转换成 Ruby 可以工作的形式。JSON
模块有一个 parse
方法允许我们这样做。
示例代码:
require 'json'
json_string = '["Orange", "Apple", [1, 2]]'
another_json_string = '{ "name": "John", "sex": "male", "grades": [60, 70, 80, 90] }'
puts JSON.parse(json_string)
puts JSON.parse(another_json_string)
输出:
["Orange", "Apple", [1, 2]]
{"name"=>"John", "sex"=>"male", "grades"=>[60, 70, 80, 90]}
为了使 JSON 模块在我们的代码中可用,我们首先需要该文件,这就是我们代码的第一行所做的。
如上例所示,JSON.parse
将 json_string
转换为 Ruby Array,并将 another_json_string
转换为 Ruby Hash。
如果你有一个不是字符串形式的 JSON 值,你可以在其上调用 to_json
以首先将其转换为 JSON 字符串,然后使用 JSON.parse
将其转换为 Array 或 Hash 视情况而定.
示例代码:
require 'json'
json_one = ["Orange", "Apple", [1, 2]]
json_two = { "name": "John", "sex": "male", "grades": [60, 70, 80, 90] }
puts JSON.parse(json_one.to_json)
puts JSON.parse(json_two.to_json)
输出:
["Orange", "Apple", [1, 2]]
{"name"=>"John", "sex"=>"male", "grades"=>[60, 70, 80, 90]}