在 Python 中根据空格拆分字符串
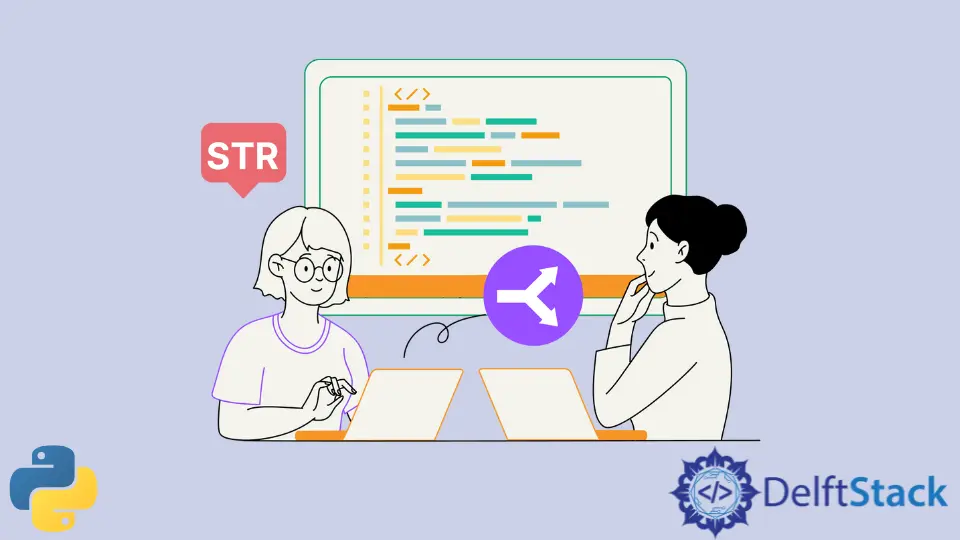
本教程将演示如何在 Python 中根据空格作为定界符来分割字符串。
在 Python 中拆分字符串意味着根据所使用的分隔符或分隔符将单个字符串切成字符串数组。
例如,如果一个字符串初始化为 Hello, World! I am here.
,使用空格作为分隔符将其拆分将产生以下输出:
['Hello,', 'World!', 'I', 'am', 'here.']
在 Python 中使用字符串 split()
方法拆分字符串
内置的 Python 字符串方法 split()
是使用空格分割字符串的理想解决方案。默认情况下,split()
方法返回由使用空格作为分隔符分割原始字符串而得到子字符串数组。
例如,让我们使用相同的字符串示例 Hello, World! I am here.
,并使用 split()
方法将字符串分割成子字符串数组。
string_list = "Hello, World! I am here.".split()
print(string_list)
输出的结果和预期的一样。
["Hello,", "World!", "I", "am", "here."]
除此之外,split()
方法还会自动删除前导和尾随空格,并将它们视为单个空格分隔符。
让我们修改前面的示例,使其包含随机的前导,尾随和连续空格。
string_list = " Hello, World! I am here. ".split()
print(string_list)
split()
方法自动处理的另一种情况是除空格文字外,还使用制表符,换行符和回车符分别用\t
,\n
和\r
表示。提到的空白格式也被认为是定界符和修剪的主题。
输出:
['Hello,', 'World!', 'I', 'am', 'here.']
例如:
string_list = " Hello, World! I am here.\nI am also\there too,\rand here.".split()
print(string_list)
输出:
['Hello,', 'World!', 'I', 'am', 'here.', 'I', 'am', 'also', 'here', 'too,', 'and', 'here.']
考虑到这些因素,你不必担心在执行函数之前显式地修剪每个空格。这是一个方便的功能。
在 Python 中使用 re.split()
分割字符串
Python RegEx(正则表达式)模块 re
还具有预定义的 split()
函数,我们可以使用它代替内置的 split()
方法。不过,请注意,在性能方面,re.split()
与内置的 split()
方法相比要慢一些。
re.split()
函数接受两个主要参数:RegEx 字符串和执行拆分功能的字符串。代表空格的 RegEx 关键字是\s
。\s
是所有空格的归类,包括上述所有的空格(\n
,\t
,\r
,\f
)。
例如,声明一个字符串并执行 re.split()
将它们拆分为子字符串数组。要考虑尾随空格和前导空格,请在 RegEx 字符串中添加一个+
符号,以将一个或多个连续空格作为单个组进行匹配。
另外,将关键字 r
附加到 RegEx 字符串中,以确保 Python 适当地处理转义序列。
import re
exStr = "Hello, World!\nWelcome\tto my tutorial\rarticle."
print(re.split(r"\s+", exStr))
在 Python 中使用 re.findall()
代替 re.split()
拆分字符串
另外,也可以使用 re.findall()
。findall()
函数与 split()
完全相反。此函数查找与给定 RegEx 字符串匹配的所有子字符串,而 split()
方法使用 RegEx 字符串作为分隔符。
要使用 findall()
函数使用空格分割字符串,请通过大写字母(\S
),它是空格匹配字符\s
的相反面。findall()
接受与 split()
相同的参数。
import re
exStr = "Hello, World!\nWelcome\tto my tutorial\rarticle."
print(re.findall(r"\S+", exStr))
这两个函数将产生相同的输出:
['Hello,', 'World!', 'Welcome', 'to', 'my', 'tutorial', 'article.']
总之,使用空格作为定界符来分割字符串的最好和最优化方法是内置的 split()
方法。它附加在字符串对象上,默认情况下考虑前导和尾随空格。使用此函数也不需要任何正则表达式知识。
否则,尽管两个函数的执行速度都比内置的 split()
方法慢,但 re.split()
和 re.findall()
可以用作 split()
方法的替代品。
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn