在 Python 中检查字符串是否包含数字
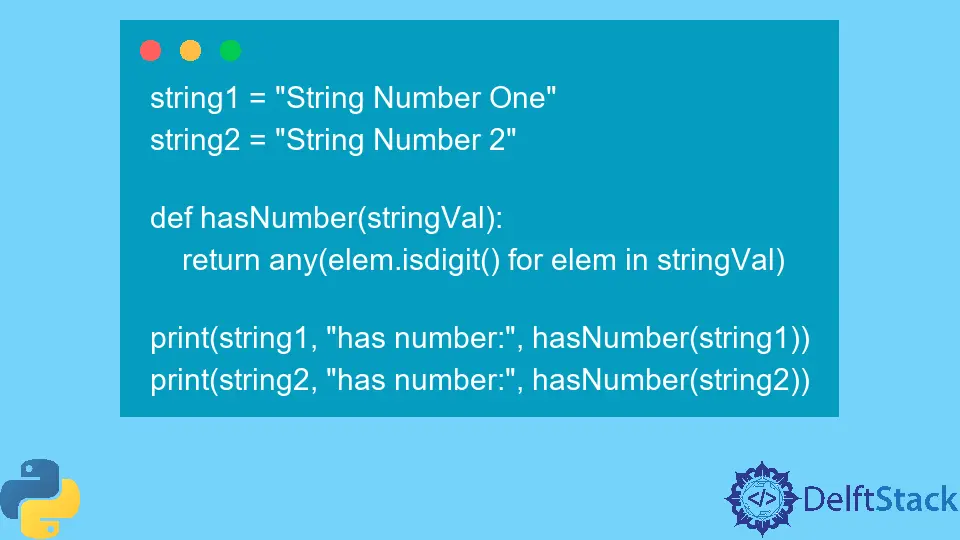
本教程将介绍在 Python 中检查一个字符串是否包含一个数字的不同方法。
Python 中使用 any()
和 isdigit()
函数来检查字符串是否包含数字
内置函数 any()
接受一个迭代对象,并将其作为布尔值读取。如果在可迭代对象中至少有一个元素被识别为 True
,函数将返回 True
,如果没有一个 True
值则返回 False
。
我们可以将 any()
函数与内置的字符串方法 isdigit()
结合起来,该方法检查一个字符串是否在其大小范围内包含任何数字字符,如果一个字符串包含 Python 中的数字,则返回 True
。
例如,让我们声明两个字符串变量,一个有数字,一个没有。之后,声明一个函数来检查字符串是否包含一个数字。
string1 = "String Number One"
string2 = "String Number 2"
def hasNumber(stringVal):
return any(elem.isdigit() for elem in stringVal)
print(string1, "has number:", hasNumber(string1))
print(string2, "has number:", hasNumber(string2))
为了进一步缩短代码,我们可以声明一个包含同样两个函数的 lambda 函数来检查字符串。
string1 = "String Number One"
string2 = "String Number 2"
def hasNumber(stringVal):
return any(elem.isdigit() for elem in stringVal)
print(string1, "has number:", hasNumber(string1))
print(string2, "has number:", hasNumber(string2))
输出:
String Number One has number: False
String Number 2 has number: True
在 Python 中使用 re.search()
检查字符串是否包含一个数字
Python 支持使用模块 re
对正则表达式进行操作。我们可以使用函数 re.search()
来检查一个给定的字符串中是否包含一个数字。
re.search()
接受两个主要参数,第一个是正则表达式,第二个是要检查的字符串是否匹配表达式。
用于匹配任何数字的表达式是\d
或 [0-9]
。在这个例子中,我们将使用\d
作为 search()
的第一个参数。
import re
string1 = "Number One Two Three"
string2 = "Number 123"
def hasNumber(stringVal):
if re.search("\d", stringVal) == None:
return False
else:
return True
print(string1, "contains number:", hasNumber(string1))
print(string2, "contains number:", hasNumber(string2))
使用 lambda 声明和三元运算符可以将函数缩短为一行,就像这样。
def hasNumber(stringVal):
return False if re.search("\d", stringVal) == None else True
输出:
Number One Two Three contains number: False
Number 123 contains number: True
就运行时间而言,正则表达式评估比使用内置的字符串函数要快得多。如果字符串的值相当大,那么 re.search()
比使用字符串函数更理想。
在给定字符串上运行 search()
函数之前,使用 re.compile()
编译表达式,也会使执行时间更快。
将 compile()
的返回值捕获到一个变量中,然后在这个变量中调用 search()
函数。在这种情况下,search()
只需要一个参数,即对照编译后的表达式搜索的字符串。
def hasNumber(stringVal):
re_numbers = re.compile("\d")
return False if (re_numbers.search(stringVal) == None) else True
综上所述,内置函数 any()
和 isdigit()
可以很容易地串联使用,检查一个字符串是否包含一个数字。
然而,在正则表达式模块 re
中使用实用函数 search()
和 compile()
会比内置的字符串函数更快地生成结果。所以,如果你要处理大的数值或字符串,那么正则表达式的解决方案要比字符串函数更理想。
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn