在 Python 中查找 Powerset
Hemank Mehtani
2023年1月30日
- 在 Python 中使用迭代方法获取 Powerset
-
在 Python 中使用
itertools.combinations
函数查找幂集 - 在 Python 中使用列表推导式查找幂集
- 在 Python 中使用递归方法查找幂集
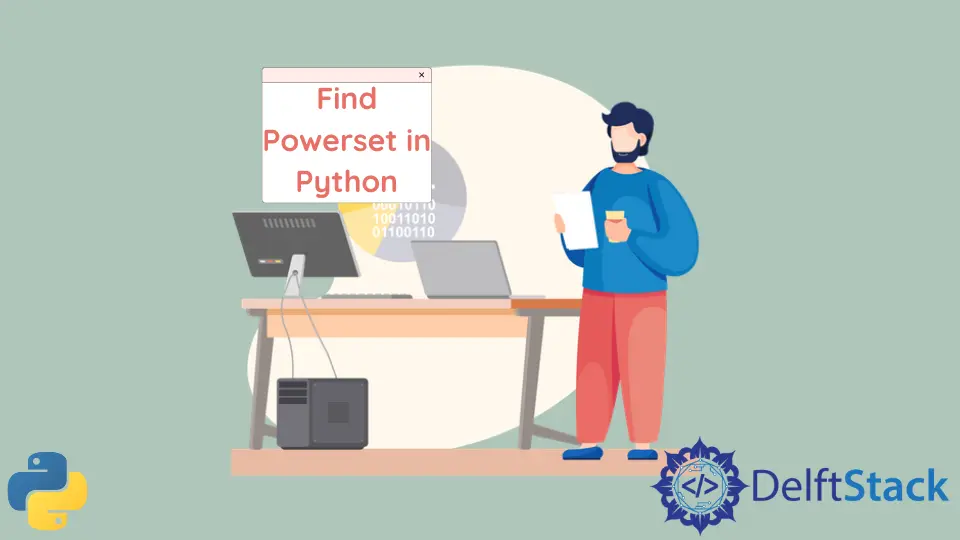
在数学中,任何集合的幂集是一个包含给定集合的所有可能子集以及一个空集的集合。换句话说,集合的所有子集也称为幂集。在 Python 中可以有一个强大的列表、集合、字符串等集合。
在本教程中,我们将在 Python 中找到给定集合的幂集。
在 Python 中使用迭代方法获取 Powerset
虽然我们可以同时使用递归方法和迭代方法来找到幂集,但迭代方法比递归方法更受欢迎,因为它的过程更快。
我们使用嵌套的 for
循环来创建这样的幂集。
例如,
def powerset(fullset):
listsub = list(fullset)
subsets = []
for i in range(2 ** len(listsub)):
subset = []
for k in range(len(listsub)):
if i & 1 << k:
subset.append(listsub[k])
subsets.append(subset)
return subsets
subsets = powerset(set([1, 2, 3, 4]))
print(subsets)
print(len(subsets))
输出:
[[], [1], [2], [1, 2], [3], [1, 3], [2, 3], [1, 2, 3], [4], [1, 4], [2, 4], [1, 2, 4], [3, 4], [1, 3, 4], [2, 3, 4], [1, 2, 3, 4]]
16
在 Python 中使用 itertools.combinations
函数查找幂集
itertools
是 Python 中的一个模块,用于迭代数据结构。这些数据结构也称为可迭代对象。可以使用 for 循环跳过它们。
这个模块的 combinations
函数可以创建一个集合的组合来创建一个 powerset。
请参考下面的代码。
from itertools import combinations
def powerset(string):
n = len(string)
for i in range(0, n + 1):
for element in combinations(string, i):
print("".join(element))
string = ["x", "y", "z"]
powerset(string)
输出:
x
y
z
xy
xz
yz
xyz
在 Python 中使用列表推导式查找幂集
列表推导式是一种基于现有列表创建新列表的方法。它提供了更短的语法,比用于创建列表的其他函数和循环更紧凑、更快。
我们也在这个方法中使用了一个嵌套的 for
循环。
例如,
def get_subsets(fullset):
listrep = list(fullset)
n = len(listrep)
return [[listrep[k] for k in range(n) if i & 1 << k] for i in range(2 ** n)]
string = ["x", "y", "z"]
print(get_subsets(string))
输出:
[[], ['x'], ['y'], ['x', 'y'], ['z'], ['x', 'z'], ['y', 'z'], ['x', 'y', 'z']]
在 Python 中使用递归方法查找幂集
递归方法是一种方法,其中函数不断使用不同的参数调用自身。我们可以创建一个递归函数来查找集合的幂集。
例如,
def powerSet(string, index, c):
if index == len(string):
print(c)
return
powerSet(string, index + 1, c + string[index])
powerSet(string, index + 1, c)
s1 = ["a", "b", "c"]
index = 0
c = ""
powerSet(s1, index, c)
输出:
abc
ab
ac
a
bc
b
c