如何在 Python 2 和 Python 3 中将字节转换为字符串
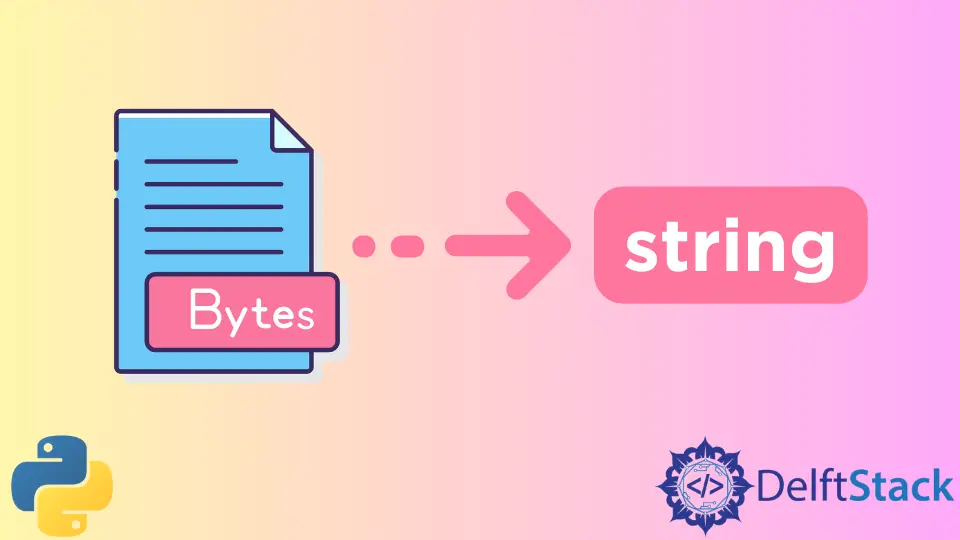
我们将来介绍将如何在 Python 2.x 和 Python 3.x 中将 bytes
转换为字符串。
在 Python 2.x 中将字节转换为字符串
Python 2.7 中的 bytes
等同于 str
,因此 bytes
变量本身就是字符串。
python 2.7.10 (default, May 23 2015, 09:44:00) [MSC v.1500 64 bit (AMD64)] on win32
Type "copyright", "credits" or "license()" for more information.
>>> A = b'cd'
>>> A
'cd'
>>> type(A)
<type 'str'>
在 Python 3.x 中将字节转换为字符串
bytes
是 Python 3 中引入的新数据类型。
python 3.6.3 (v3.6.3:2c5fed8, Oct 3 2017, 18:11:49) [MSC v.1900 64 bit (AMD64)] on win32
Type "copyright", "credits" or "license()" for more information.
>>> A = b'cd'
>>> A
b'cd'
>>> type(A)
<class 'bytes'>
>>>
bytes
中的元素的数据类型为 int
。
>>> A = b'cd'
>>> A[0]
99
>>> type(A[0])
<class 'int'>
在 Python 3.x 中通过 decode
将字节转换为字符串
bytes
的 decode
方法可以使用给定的 encoding
将字节转换为字符串。在大多数情况下,如果将 encoding
方法没有被指定,那默认设置是 utf-8
。但它并非总是安全的,因为字节可以使用其他的不同于 utf-8
的编码方法进行编码。
>>> b'\x50\x51'.decode()
'PQ'
>>> b'\x50\x51'.decode('utf-8')
'PQ'
>>> b'\x50\x51'.decode(encoding = 'utf-8')
'PQ'
如上所示,三种对 bytes
的解码方式得到的结果相同,因为它们都是用 utf-8
作编码方法。
如果 bytes
不是用 utf-8
编码,但你用 utf-8
解码的话,系统会报 UnicodeDecodeError
错误。
>>> b'\x50\x51\xffed'.decode('utf-8')
Traceback (most recent call last):
File "<pyshell#16>", line 1, in <module>
b'\x50\x51\xffed'.decode('utf-8')
UnicodeDecodeError: 'utf-8' codec can't decode byte 0xff in position 2: invalid start byte
我们有两种方法可以解决此类 encoding
问题。
backslashreplace
, ignore
或者 replace
作为 errors
的参数
decode
除 encoding
外,还有另一个参数 errors
。它定义了 error
事件发生时的行为。默认值 errors
是 strict
,这意味着如果在解码过程中发生错误,则会抛出错误。
error
还有其他选项,如 ignore
,replace
或其他注册的 codecs.register_error
名称,比如 backslashreplace
。
ignore
忽略错误的解码错误,并尽可能创建输出字符串。
replace
用 encoding
给定方法中定义的字符替换相应的字符。backslashreplace
用与原始 bytes
内容相同的内容替换无法解码的字符。
>>> b'\x50\x51\xffed'.decode('utf-8', 'backslashreplace')
'PQ\\xffed'
>>> b'\x50\x51\xffed'.decode('utf-8', 'ignore')
'PQed'
>>> b'\x50\x51\xffed'.decode('utf-8', 'replace')
'PQ�ed'
如果 bytes
数据的编码未知,则可以使用 MS-DOS cp437
编码。
>>> b'\x50\x51\xffed'.decode('cp437')
'PQ\xa0ed'
在 Python 3.x 中用 chr
将字节转换为字符串
chr(i, /)
返回一个包含一个序号的字符的 Unicode 字符串,它可以将 bytes
的单个元素转换为 string
,而不是转换整个 bytes
。
我们可以使用列表推导或 map
,用 chr
来获取 bytes
单个元素转换成的字符,从而得到整个转换后的字符串。
>>> A = b'\x50\x51\x52\x53'
>>> "".join([chr(_) for _ in A])
'PQRS'
>>> "".join(map(chr, A))
'PQRS'
Python 字节转换为字符串的不同方法的性能比较和结论
我们用 timeit
来比较本教程中介绍的方法- decode
和 chr
的性能。
>>> import timeit
>>> timeit.timeit('b"\x50\x51\x52\x53".decode()', number=1000000)
0.1356779
>>> timeit.timeit('"".join(map(chr, b"\x50\x51\x52\x53"))', number=1000000)
0.8295201999999975
>>> timeit.timeit('"".join([chr(_) for _ in b"\x50\x51\x52\x53"])', number=1000000)
0.9530071000000362
从上面显示的时间性能中可以看出,decode()
它要比 chr()
快得多。chr()
效率相对较低,因为它需要从单个字符串字符中重建字符串。
我们建议在性能重要的应用程序中使用 decode
。
相关文章 - Python Bytes
- Python 中如何将字节 bytes 转换为整数 int
- 如何将整型 int 转换为字节 bytes
- 如何在 Python 中把整型转换为二进制
- 在 Python 中转换字节为十六进制
- 在Python中将十六进制转换为字节