在 Pandas DataFrame 中将多列中的值合并为一列
- 从头开始编写代码以将多列中的值合并到 Pandas DataFrame 中的一列中
- 在 Pandas DataFrame 中使用 DuckDB 运行 SQL 查询以将多列中的值合并为一列
-
在 Pandas DataFrame 中使用
combine_first()
方法将多列中的值合并为一列 -
在 Pandas DataFrame 中使用
bfill()
方法将多列中的值合并为一列 -
在 Pandas DataFrame 中使用
mask()
方法将多列中的值合并为一列
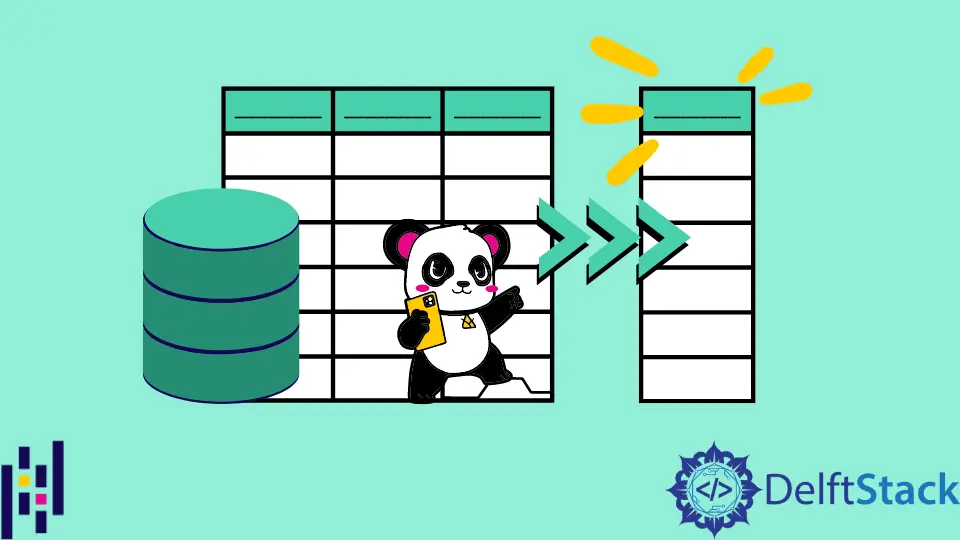
本教程将演示将多列中的第一个非空值合并或返回到 Python Pandas DataFrame 中的另一列。
例如,如果它不为空,则将第 1 列的值用于新的第 3 列;否则,如果第 1 列为空,则将第 2 列的值用于新的第 3 列。
我们可以在 Pandas DataFrame 中以多种方式完成此任务。
从头开始编写代码以将多列中的值合并到 Pandas DataFrame 中的一列中
我们可以从头开始编写逻辑来合并值。我们在以下代码中创建了一个 Pandas DataFrame,其中包含三列,名为 Age_in_Years
、Age_in_Months
和 Age_in_Days
。
DataFrame 也有一些缺失值。如果我们要显示年龄,首先,我们将输出年龄,以年为单位。
如果该列中的值为 Null,我们将以月为单位显示年龄。同样,如果以月为单位的值为 Null,我们将以天为单位显示年龄。
为此,我们从头开始编写代码来获取第一个非空列的值。该函数正在遍历所有 DataFrame 列,并在找到非空值的地方返回该值;否则,它会检查其他列中的值。
示例代码:
# Python 3.x
import pandas as pd
df_age = pd.DataFrame(
{
"Age_in_Years": ["4 y", None, None, None],
"Age_in_Months": ["48 m", "24 m", None, None],
"Age_in_Days": ["1440 d", None, "2520 d", None],
}
)
def get_first_non_null(dfrow, cols):
for c in cols:
if pd.notnull(dfrow[c]):
return dfrow[c]
return None
cols = ["Age_in_Years", "Age_in_Months", "Age_in_Days"]
df_age["Age"] = df_age.apply(lambda x: get_first_non_null(x, cols), axis=1)
display(df_age)
输出:
在 Pandas DataFrame 中使用 DuckDB 运行 SQL 查询以将多列中的值合并为一列
示例代码:
DuckDB 是一个 Python API 和一个使用 SQL 查询与数据库交互的数据库管理系统。这个包有一个内置的合并方法,可以从列中选择第一个非空值。
我们将在 SQL 查询中将列名传递给 coalesce 方法。
# Python 3.x
import pandas as pd
import duckdb
df_age = pd.DataFrame(
{
"Age_in_Years": ["4 y", None, None, None],
"Age_in_Months": ["48 m", "24 m", None, None],
"Age_in_Days": ["1440 d", None, "2520 d", None],
}
)
df_age = duckdb.query(
"""SELECT Age_in_Years, Age_in_Months, Age_in_Days, coalesce(Age_in_Years, Age_in_Months, Age_in_days) as Age from df_age"""
).to_df()
display(df_age)
输出:
在 Pandas DataFrame 中使用 combine_first()
方法将多列中的值合并为一列
combine_first()
方法用来自第二个 DataFrame 的非空数据填充一个 DataFrame 中的空值,以组合两个 DataFrame 对象。
在下面的代码中,我们将返回列值。我们将把 Age_in_Years
与 Age_in_Months
结合起来,将 Age_in_Months
与 Age_in_Days
结合起来。
它将返回来自 Age_in_years
的值。如果为 Null,它将返回来自 Age_in_Months
的值。同样,如果这也是 Null,它将从 Age_in_Days
返回一个值。
实际 DataFrame 中的数据不会改变,我们将在 Age
列中获得我们想要的值。
示例代码:
# Python 3.x
import pandas as pd
df_age = pd.DataFrame(
{
"Age_in_Years": ["4 y", None, None, None],
"Age_in_Months": ["48 m", "24 m", None, None],
"Age_in_Days": ["1440 d", None, "2520 d", None],
}
)
df_age["Age"] = (
df_age["Age_in_Years"]
.combine_first(df_age["Age_in_Months"])
.combine_first(df_age["Age_in_Days"])
)
df_age
输出:
在 Pandas DataFrame 中使用 bfill()
方法将多列中的值合并为一列
bfill
代表反向填充。此方法将 NaN 替换为下一行或下一列值。
在这里,如果当前列中的值为 Null,我们将指定 axis=1
从下一列返回值。
示例代码:
# Python 3.x
import pandas as pd
df_age = pd.DataFrame(
{
"Age_in_Years": ["4 y", None, None, None],
"Age_in_Months": ["48 m", "24 m", None, None],
"Age_in_Days": ["1440 d", None, "2520 d", None],
}
)
df_age["Age"] = df_age.bfill(axis=1).iloc[:, 0]
df_age
输出:
在 Pandas DataFrame 中使用 mask()
方法将多列中的值合并为一列
mask()
方法的工作方式与 if-then
类似。
如果某个列的 null 条件为 false,则将使用其值。否则,它将从其他指定列中获取值。
示例代码:
# Python 3.x
import pandas as pd
df_age = pd.DataFrame(
{
"Age_in_Years": ["4 y", None, None, None],
"Age_in_Months": ["48 m", "24 m", None, None],
"Age_in_Days": ["1440 d", None, "2520 d", None],
}
)
df_age["Age"] = (
df_age["Age_in_Years"]
.mask(pd.isnull, df_age["Age_in_Months"])
.mask(pd.isnull, df_age["Age_in_Days"])
)
df_age
输出:
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn