在 PHP 中生成 UUID
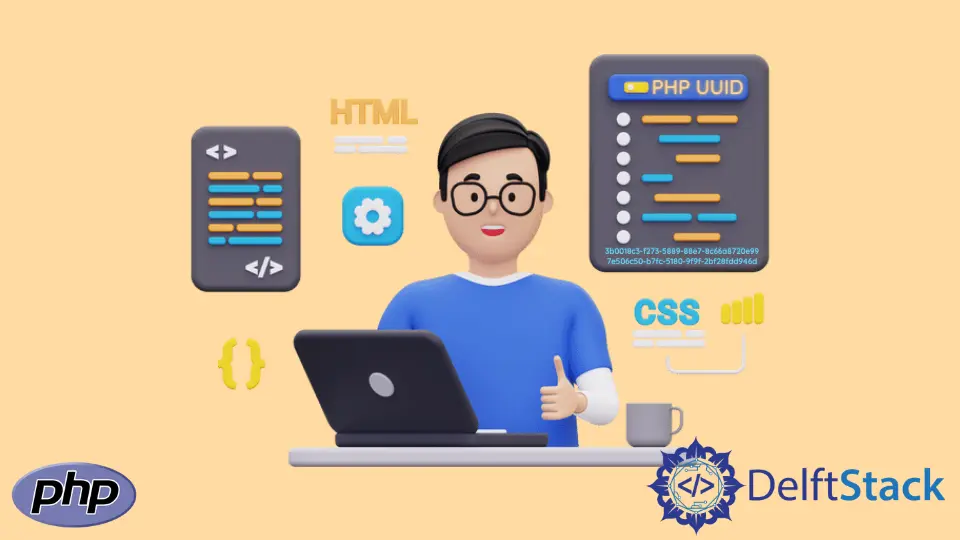
在 PHP 中生成一个普遍唯一的 id UUID
可能会很棘手,因为内置函数 uniqid()
不能保证输出 id 的唯一性。
我们可以创建函数来在 PHP 中生成不同版本的 UUID
,包括 v3、v4 和 v5。本教程演示如何在 PHP 中生成 UUID
。
在 PHP 中创建一个生成 V3 UUID
的函数
V3 UUID
是从命名空间和给定字符串的 MD5 散列生成的唯一 ID。下面的函数是在 PHP 中生成 V3 UUID
的示例。
<?php
function v3_UUID($name_space, $string) {
$n_hex = str_replace(array('-','{','}'), '', $name_space); // Getting hexadecimal components of namespace
$binary_str = ''; // Binary Value
//Namespace UUID to bits conversion
for($i = 0; $i < strlen($n_hex); $i+=2) {
$binary_str .= chr(hexdec($n_hex[$i].$n_hex[$i+1]));
}
//hash value
$hashing = md5($binary_str . $string);
return sprintf('%08s-%04s-%04x-%04x-%12s',
// 32 bits for the time low
substr($hashing, 0, 8),
// 16 bits for the time mid
substr($hashing, 8, 4),
// 16 bits for the time hi,
(hexdec(substr($hashing, 12, 4)) & 0x0fff) | 0x3000,
// 8 bits and 16 bits for the clk_seq_hi_res,
// 8 bits for the clk_seq_low,
(hexdec(substr($hashing, 16, 4)) & 0x3fff) | 0x8000,
// 48 bits for the node
substr($hashing, 20, 12)
);
}
$v3_uuid = v3_UUID('5f6384bfec4ca0b2d4114a13aa2a5435', 'delftstack!');
echo $v3_uuid;
$v3_uuid = v3_UUID('591531f16f581b69a390980eb282ba83', 'this is delftstack!');
echo "<br>";
echo $v3_uuid;
?>
上面的代码从给定的命名空间 md5 哈希和字符串生成版本 3 通用唯一 ID。
输出:
18e24dee-96e1-3dbd-9c3c-e684c1a10fd5
3bf89731-20f0-3d78-b06a-3f70c6fff898
在 PHP 中创建一个生成 V4 UUID
的函数
版本 4 UUID
基于随机数生成生成唯一 ID。
<?php
function v4_UUID() {
return sprintf('%04x%04x-%04x-%04x-%04x-%04x%04x%04x',
// 32 bits for the time_low
mt_rand(0, 0xffff), mt_rand(0, 0xffff),
// 16 bits for the time_mid
mt_rand(0, 0xffff),
// 16 bits for the time_hi,
mt_rand(0, 0x0fff) | 0x4000,
// 8 bits and 16 bits for the clk_seq_hi_res,
// 8 bits for the clk_seq_low,
mt_rand(0, 0x3fff) | 0x8000,
// 48 bits for the node
mt_rand(0, 0xffff), mt_rand(0, 0xffff), mt_rand(0, 0xffff)
);
}
for ($x = 0; $x <= 10; $x++) {
$v4_uuid = v4_UUID();
echo $v4_uuid;
echo "<br>";
}
?>
版本 4 UUID
函数生成一个新的唯一 ID。上面的代码尝试运行该函数十次。
输出:
20a9d4e8-63a8-48f0-910f-c7339d8fd7ec
499a9d4a-fbf1-4ea7-850b-01bf301a98af
8fa903bc-0789-43b2-901b-70d6c60334ba
eb036f8a-75bd-4811-a477-1444e2521f3b
25c00e25-9042-4f04-b059-c34820b800f8
7f65c049-1feb-4d41-98ee-35156e81221f
abfd9937-a08b-47b0-8b64-3338455d99f4
43a9245a-275a-4b23-8ac0-a63fefa13013
bbfc7b5b-f77f-4a58-a3ce-5163bac61a4c
f660bbbf-dd1a-4eab-9866-dba8092c94c5
b7f6046a-b834-48f0-856e-8a360b495406
在 PHP 中创建一个生成 V5 UUID
的函数
V5 UUID
是从 SHA-1
散列命名空间和给定字符串生成的唯一 ID。
<?php
function v5_UUID($name_space, $string) {
$n_hex = str_replace(array('-','{','}'), '', $name_space); // Getting hexadecimal components of namespace
$binray_str = ''; // Binary value string
//Namespace UUID to bits conversion
for($i = 0; $i < strlen($n_hex); $i+=2) {
$binray_str .= chr(hexdec($n_hex[$i].$n_hex[$i+1]));
}
//hash value
$hashing = sha1($binray_str . $string);
return sprintf('%08s-%04s-%04x-%04x-%12s',
// 32 bits for the time_low
substr($hashing, 0, 8),
// 16 bits for the time_mid
substr($hashing, 8, 4),
// 16 bits for the time_hi,
(hexdec(substr($hashing, 12, 4)) & 0x0fff) | 0x5000,
// 8 bits and 16 bits for the clk_seq_hi_res,
// 8 bits for the clk_seq_low,
(hexdec(substr($hashing, 16, 4)) & 0x3fff) | 0x8000,
// 48 bits for the node
substr($hashing, 20, 12)
);
}
$v5_uuid = v5_UUID('8fc990b07418d5826d98de952cfb268dee4a23a3', 'delftstack!');
echo $v5_uuid;
$v5_uuid = v5_UUID('24316ec81e3bea40286b986249a41e29924d35bf', 'this is delftstack!');
echo "<br>";
echo $v5_uuid;
?>
上面的代码从给定的命名空间 SHA-1
哈希和字符串生成版本 5 通用唯一 ID。
输出:
3b0018c3-f273-5889-88e7-8c66a8720e99
7e506c50-b7fc-5180-9f9f-2bf28fdd946d
除了生成 UUIDs
之外,还提供了一些库,例如 Ramsey/uuid 和 Lootils/uuid,它们可以方便地使用。
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook