如何在 Bash 中使用 if...else 语句
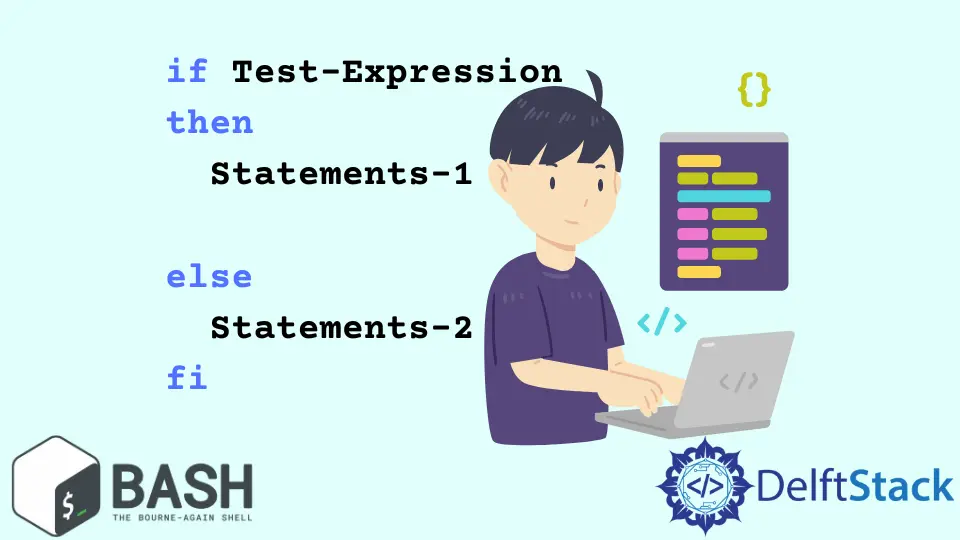
条件语句在几乎所有的编程语言中都很普遍,用于决策。它们只允许在满足特定条件的情况下执行一条或多条语句。if ... else
在大多数编程语言中被用作条件语句。在 Bash
中,我们还有 if
、if...elif...elfer
、if ... else
和嵌套的 if
语句作为条件语句。
Bash
中的 if
语句
if
语句的语法
if Test-Expression
then
Statements
fi
在上面的例子中,如果 Test-Expression
是 True
,则执行 Statements
。fi
关键字用于结束 if
语句。
如果 Test-Expression
不是 True
,Statements
都不会被执行。
为了使我们的代码看起来更易读,更有条理,我们可以使用 4 空格或 2 空格缩进。
示例:Bash
中的 if
语句
echo -n "Enter numnber : "
read n
rem=$(( $n % 2 ))
if [ $rem -eq 0 ]
then
echo "$n is even number"
fi
输出:
Enter numnber : 4
4 is even number
它接受用户提供的数字,只有当数字是偶数时才会输出。
如果数字是偶数,那么当数字除以二时,余数为零,因此测试表达式为 True
,这样 echo
语句就会被执行。
Bash
中的 if ... else
语句
if ... else
语句的语法
if Test-Expression
then
Statements-1
else
Statements-2
fi
在这个例子中,如果 Test-Expression
是 True
,则执行 Statements-1
;否则,执行 Statements-2
。在结束 if ... else
语句时,使用 fi
关键字。
例子:Bash
中的 if...else
语句
echo -n "Enter numnber : "
read n
rem=$(( $n % 2 ))
if [ $rem -eq 0 ]
then
echo "$n is even number"
else
echo "$n is odd number"
fi
输出:
Enter numnber : 5
4 is odd number
它接受用户提供的一个数字,并根据输入数字是否正好被 2
整除而给出输出。
如果数字是偶数,当数字除以 2 时,余数为零;因此,测试表达式为 True
,语句 echo "$n is even number"
得到执行。
如果数字是奇数,余数不为零;因此,测试表达式为 False
,语句 echo "$n is odd number"
被执行。
if...elif...else
语句在 Bash
中的应用
if...elif...else
语句的语法
if Test-Expression-1
then
Statements-1
elif Test-Expression-2
then
Statements-2
else
Statements-3
fi
如果 Test-Expression-1
为 True
,则执行 Statements-1
;否则,如果 Test-Expression-2
为 True
,则执行 Statements-2
。
如果两个测试表达式都不为 True
,则执行 Statements-3
。
我们可以有任意多的 elif
语句,else
语句是可选的。
例子:Bash
中的 if...elif...else
语句
echo -n "Enter the value of a: "
read a
echo -n "Enter the value of b: "
read b
if [ $a -lt $b ]
then
echo "a is less than b"
elif [ $a -gt $b ]
then
echo "a is greater than b"
else
echo "a is equal to b"
fi
输出:
Enter the value of a: 4
Enter the value of b: 4
a is equal to b
它接受两个数字作为用户的输入,并根据哪个测试表达式为真打印结果。
如果 a<b
,程序打印 a is less than b
。
如果 a>b
,程序打印 a is greater then b
。
如果这两个条件语句都不为真,程序就会打印出 a is equal to b
。
嵌套在 Bash
中的 if
语句
当一个 if
语句放在另一个 if
语句中时,称为嵌套 if
语句。
echo -n "Enter numnber : "
read a
rem=$(( $a % 2 ))
if [ $rem -eq 0 ]
then
if [ $a -gt 10 ]
then
echo "$a is even number and greater than 10."
else
echo "$a is even number and less than 10."
fi
else
echo "$a is odd number"
fi
输出:
Enter numnber : 46
46 is even number and greater than 10.
它演示了嵌套的 if
语句的用法。如果数字正好被 2
除以 2
且大于 10
,则执行 echo "$a is even number and greater than 10."
语句。
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn