Kotlin 中的 Getter 和 Setter
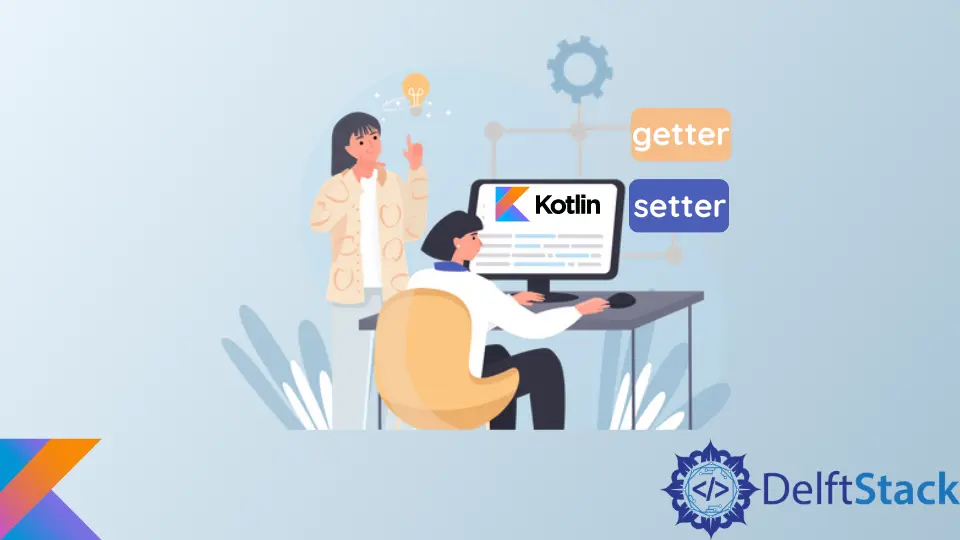
本文将介绍 Kotlin 中的 getter 和 setter 及其使用方法。我们还将演示一些示例来理解 getter 和 setter 的工作。
Kotlin 中的 Getter 和 Setter
在一般编程中,getter 帮助获取属性的值。同样,设置属性的值是使用 setter 完成的。
Kotlin 的 getter 和 setter 是自动生成的。因此,我们不需要为我们的程序单独创建它们。
所以,如果我们写,
class Student {
var Name: String = "John Doe"
}
上面的代码等价于下面的 Kotlin 程序。
class Student {
var Name: String = "John Doe"
get() = field
set(para) {
field = value
}
}
因此,当我们实例化一个类的对象时,如上例中的 Student
,并初始化一个属性,setter
函数会自动将值设置为使用 getter
函数访问的参数。
Kotlin 中的可变和不可变属性
在 Kotlin 中,我们可以创建两种类型的属性:可变的和不可变的。
可变属性使用由关键字 var
表示的可变变量声明。不可变关键字使用由关键字 val
标记的不可更改变量来表示。
这些属性之间的另一个区别是只读或不可变属性不会创建设置器,因为它不需要更改任何内容。
这是一个基于上述语法在 Kotlin 中同时使用 get()
和 set()
函数的示例。
fun main(args: Array<String>) {
val v = Student()
v.Name = "John Doe"
println("${v.Name}")
}
class Student {
var Name: String = "defValue"
get() = field
set(defaultValue) {
field = defaultValue
}
}
输出:
使用 Kotlin Set 作为私有修饰符
默认的 getter 和 setter 有一个 public 修饰符。如果我们想通过使用 private
关键字来使用带有 private 修饰符的 setter,我们可以更改此设置。
通过声明一个私有的 setter,我们只能通过类中的一个方法来设置一个值。
在本例中,我们将为 Student
类创建一个对象并访问最初设置为 John Doe 的 Name 属性。接下来,我们将通过类内的函数将名称分配给 Jane Doe 并重新访问它。
class Student () {
var Name: String = "John Doe"
private set
fun randFunc(n: String) {
Name = n
}
}
fun main(args: Array<String>) {
var s = Student()
println("Initially, name of the student is: ${s.Name}")
s.randFunc("Jane Doe")
println("Now, name of the student is: ${s.Name}")
}
输出:
Kotlin 中的自定义 Getter 和 Setter
我们还可以在 Kotlin 中制作自定义的 getter 和 setter。这是一个示例,我们为属性构建自定义的 get()
和 set()
方法。
class Student{
var Name: String = ""
get() {
println("We are in Name property's get()")
return field.toString()
}
set(defaultValue) {
println("We are in Name property's set()")
field = defaultValue
}
val ID: Int = 1013
get(){
println("We are in ID property's get()")
return field
}
}
fun main() {
val stu = Student()
stu.Name = "John Doe"
println("The age of ${stu.Name} is ${stu.ID}")
}
输出:
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn