用 Java 发送电子邮件
- 执行 JavaMail 之前需要采取的步骤
- 用 Java 从 Gmail 发送电子邮件
- 使用 JavaMail API 从 Microsoft 电子邮件发送电子邮件
- 使用 Java 中的 Apache Commons 库发送电子邮件
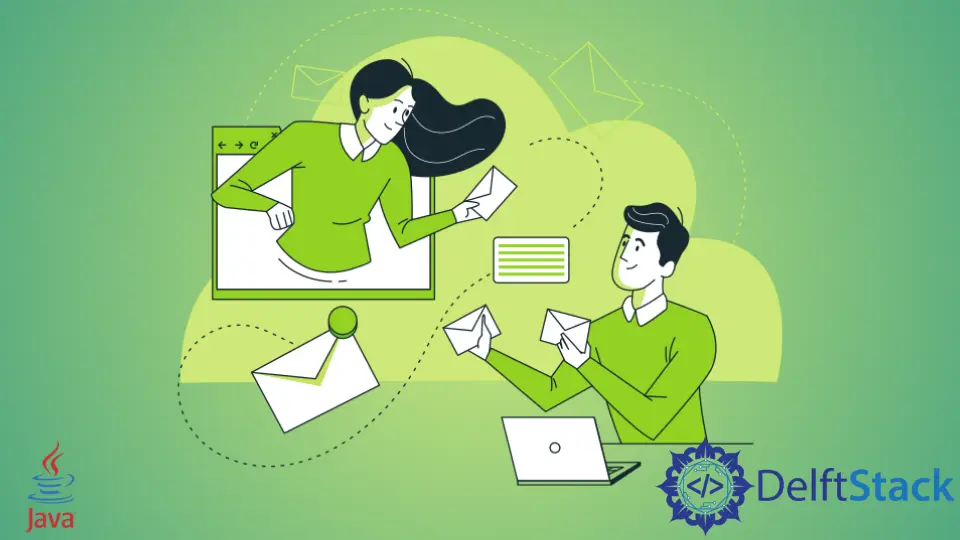
本文将分享使用 JavaMail 和 Apache Commons API 发送电子邮件的最新技术。本次演示共有三个程序。
你可以使用第一个程序从 Gmail 发送电子邮件。第二个程序将揭示如何使用 Microsoft 以 Java 发送电子邮件,而最后一个程序将简单演示你如何使用 Apache Commons 使用 Java 发送电子邮件。
执行 JavaMail 之前需要采取的步骤
- 下载并配置:JavaMail jar 文件和激活 jar 文件
- 关闭两步验证和不太安全的应用程序
- 配置构建路径:点击你的 Java 项目,配置构建路径和库部分,在 classpath 下添加外部 jar 文件。
一旦你成功地完成了这些步骤,你的第一个程序就不会抛出任何异常。
用 Java 从 Gmail 发送电子邮件
在执行我们的程序之前,让我们解释一下代码是如何工作的。
Properties
:这些描述了一组永久的属性,其中键是我们用来在每个键中设置值的字符串。MimeMessage
:它包含三个重要变量,主题、收据、消息文本。这些变量有助于获取用户输入以扩展 MimeMessage。Session
:JavaMail API 包含会话属性。我们也可以覆盖会话,但这不是此任务的目标。Transport
:此 API 中的这个通用类表示发送电子邮件的消息传输功能。
代码:
import java.util.Properties;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.PasswordAuthentication;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
// Main Class
public class FirstProgram {
public static void main(String[] args) {
// Turn off Two Factor Authentication
// Turn off less secure app
final String sender = "Write Yor Email Address"; // The sender email
final String urpass = "The Password of your email here"; // keep it secure
Properties set = new Properties();
// Set values to the property
set.put("mail.smtp.starttls.enable", "true");
set.put("mail.smtp.auth", "true");
set.put("mail.smtp.host", "smtp.gmail.com");
set.put("mail.smtp.port", "587");
Session session = Session.getInstance(set, new javax.mail.Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(sender, urpass);
}
});
try {
// email extends Java's Message Class, check out javax.mail.Message class to read more
Message email = new MimeMessage(session);
email.setFrom(new InternetAddress("senderEmail")); // sender email address here
email.setRecipients(Message.RecipientType.TO,
InternetAddress.parse("receiverEmail")); // Receiver email address here
email.setSubject("I am learning how to send emails using java"); // Email Subject and message
email.setText("Hi, have a nice day! ");
Transport.send(email);
System.out.println("Your email has successfully been sent!");
} catch (MessagingException e) {
throw new RuntimeException(e);
}
}
}
输出:
Your email has successfully been sent!
你还可以查看以下 GIF 中的输出:
使用 JavaMail API 从 Microsoft 电子邮件发送电子邮件
尽管代码块的核心功能与我们已经讨论过的第一个程序相同,但某些功能可能会有所不同:
import java.util.Date;
:它可以生成一个日期对象并实例化它以匹配创建时间,测量到最接近的毫秒。import javax.mail.Authenticator;
:它提供了一个学习如何获得连接身份验证的对象。通常,这是通过向用户提供信息来完成的。import javax.mail.PasswordAuthentication;
:这是一个安全的容器,我们用来收集用户名和密码。注意:我们也可以根据自己的喜好覆盖它。import javax.mail.internet.InternetAddress;
:它表示一个使用RFC822
语法结构存储互联网电子邮件地址的类。
javax.mail
API 中的一些类值得讨论以了解以下代码结构。
代码:
import java.util.Date;
import java.util.Properties;
import javax.mail.Authenticator;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.PasswordAuthentication;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
public class SendEmailUsingJavaOutlook {
// Define contstant strings and set properties of the email
final String SERVIDOR_SMTP = "smtp.office365.com";
final int PORTA_SERVIDOR_SMTP = 587;
final String CONTA_PADRAO = "sender email here";
final String SENHA_CONTA_PADRAO = "sender password";
final String sender = "Write your email again";
final String recvr = "Whom do you want to send?";
final String emailsubject = "Subject of the email";
// Note: you can use the date function just like these strings
final String msg = "Hi! I am sending this email from a java program.";
public void Email() {
// Set the session of email
final Session newsession = Session.getInstance(this.Eprop(), new Authenticator() {
@Override
// password authenication
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(CONTA_PADRAO, SENHA_CONTA_PADRAO);
}
});
// MimeMessage to take user input
try {
final Message newmes = new MimeMessage(newsession);
newmes.setRecipient(Message.RecipientType.TO, new InternetAddress(recvr));
newmes.setFrom(new InternetAddress(sender));
newmes.setSubject(emailsubject); // Takes email subject
newmes.setText(msg); // The main message of email
newmes.setSentDate(new Date()); // You can set the date of the email here
Transport.send(newmes); // Transfort the email
System.out.println("Email sent!");
} catch (final MessagingException ex) { // exception to catch the errors
System.out.println("try hard!"); // failed
}
}
// The permenant set of prperties containg string keys, the following configuration enables the
// SMPTs to function
public Properties Eprop() {
final Properties config = new Properties();
config.put("mail.smtp.auth", "true");
config.put("mail.smtp.starttls.enable", "true");
config.put("mail.smtp.host", SERVIDOR_SMTP);
config.put("mail.smtp.port", PORTA_SERVIDOR_SMTP);
return config;
}
public static void main(final String[] args) {
new SendEmailUsingJavaOutlook().Email();
}
}
输出:
Email sent!
你还可以在下图中查看输出:
使用 Java 中的 Apache Commons 库发送电子邮件
你可以从这里下载 Commons Email 并将 commons-email-1.5.jar 文件添加到你的 IDE 的构建路径中。打开这些库文件并阅读代码以了解更多信息。
这个最终的代码块使用了所有的 Apache 类,但我们总是可以根据我们的要求定制它们。
代码:
import org.apache.commons.mail.DefaultAuthenticator;
import org.apache.commons.mail.Email;
import org.apache.commons.mail.EmailException;
import org.apache.commons.mail.SimpleEmail;
public class SendMailWithApacheCommonsJava {
@SuppressWarnings("deprecation")
public static void main(String[] args) throws EmailException {
Email sendemail = new SimpleEmail();
sendemail.setSmtpPort(587);
sendemail.setAuthenticator(
new DefaultAuthenticator("uremail", "urpassword")); // password and email
sendemail.setDebug(false);
sendemail.setHostName("smtp.gmail.com");
sendemail.setFrom("uremail");
sendemail.setSubject("The subject of your email");
sendemail.setMsg("Your email Message");
Your Email Address sendemail.addTo("receivermail"); // Receiver Email Address
sendemail.setTLS(true);
sendemail.send(); // sending email
System.out.println("You have sent the email using Apache Commons Mailing API");
}
}
输出:
You have sent the email using Apache Commons Mailing API
你还可以在下面提供的 GIF 中看到输出:
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn