在 Java 中检查一个数字是否是质数
Sheeraz Gul
2023年10月12日
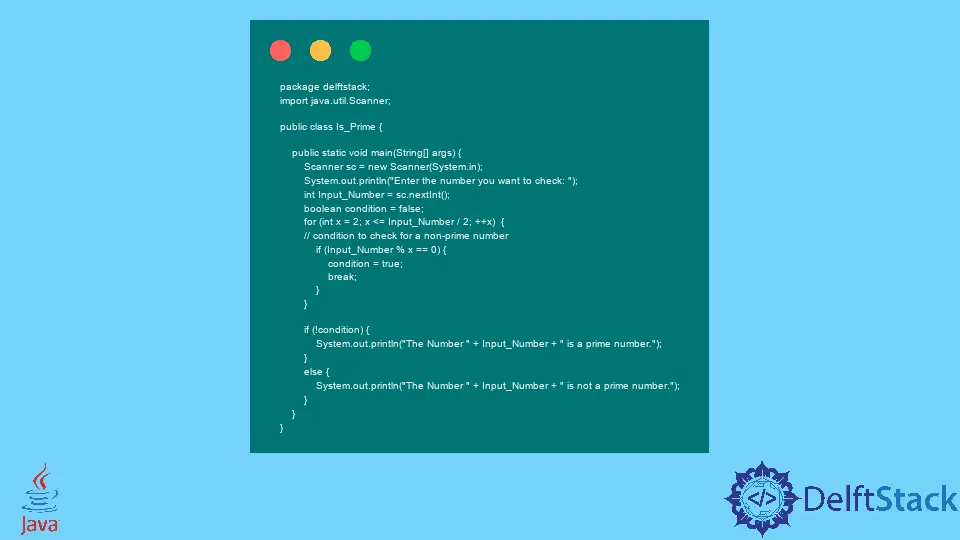
在 Java 中,我们可以实现不同的方法来检查一个数字是否是质数。本教程演示了检查数字是否为质数的不同方法。
在 Java 中使用 while
循环检查一个数是否为质数
你可以使用 while
循环开发一种方法来检查输入数字是否为质数。
示例代码:
package delftstack;
import java.util.Scanner;
public class Is_Prime {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter the number you want to check: ");
int Input_Number = sc.nextInt();
int temp = 2;
boolean condition = false;
while (temp <= Input_Number / 2) {
// condition to check for a non-prime number
if (Input_Number % temp == 0) {
condition = true;
break;
}
++temp;
}
if (!condition) {
System.out.println("The Number " + Input_Number + " is a prime number.");
} else {
System.out.println("The Number " + Input_Number + " is not a prime number.");
}
}
}
输出:
Enter the number you want to check:
11
The Number 11 is a prime number.
或者,
Enter the number you want to check:
90
The Number 90 is not a prime number.
在 Java 中使用 for
循环检查一个数是否为质数
你还可以利用 for
循环创建一个方法来检查输入数字是否为质数。
示例代码:
package delftstack;
import java.util.Scanner;
public class Is_Prime {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter the number you want to check: ");
int Input_Number = sc.nextInt();
boolean condition = false;
for (int x = 2; x <= Input_Number / 2; ++x) {
// condition to check for a non-prime number
if (Input_Number % x == 0) {
condition = true;
break;
}
}
if (!condition) {
System.out.println("The Number " + Input_Number + " is a prime number.");
} else {
System.out.println("The Number " + Input_Number + " is not a prime number.");
}
}
}
输出:
Enter the number you want to check:
3
The Number 3 is a prime number.
在 Java 中创建一个方法来检查一个数字是否为质数
我们将创建一个名为 isPrime()
的方法,并使用它来检查输入数字是否为质数。
示例代码:
package delftstack;
import java.util.Scanner;
public class Is_Prime {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter the number you want to check: ");
int Input_Number = sc.nextInt();
if (isPrime(Input_Number)) {
System.out.println("The Number " + Input_Number + " is a prime number.");
} else {
System.out.println("The Number " + Input_Number + " is not a prime number.");
}
}
static boolean isPrime(int input_number) {
if (input_number <= 1) {
return false;
}
// check for a non-prime number
for (int x = 2; x < input_number; x++) {
if (input_number % x == 0) {
return false;
}
}
return true;
}
}
输出:
Enter the number you want to check:
10
The Number 10 is not a prime number.
作者: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook