在 Go 中执行 Shell 命令
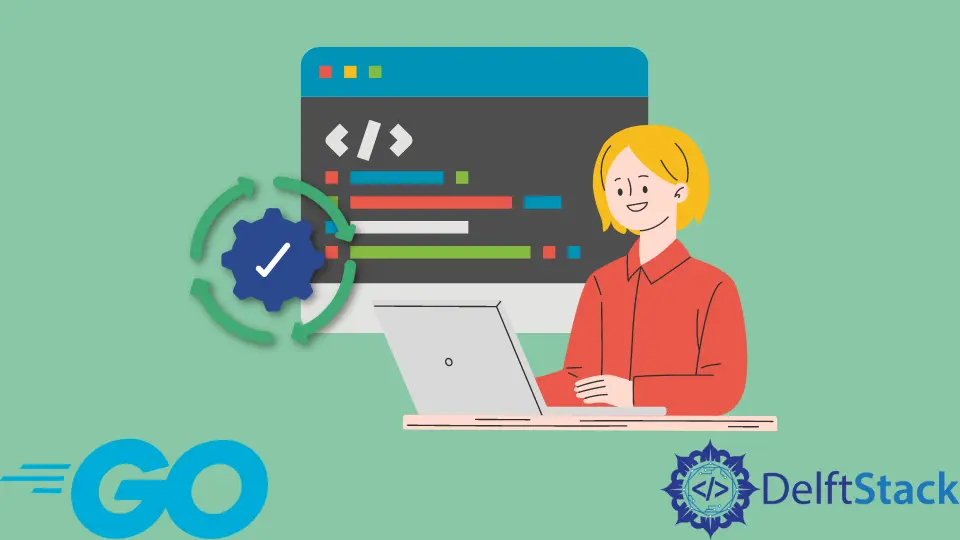
Shell 命令告诉系统如何执行特定任务。外部命令通过包 exec
执行。
os/exec 包与 C 和其他语言的系统库调用不同,它不执行系统 shell,也不扩展任何 glob 模式或处理任何类似于 shell 的扩展、管道或重定向。
该包更类似于 C 语言中的 exec 系列函数。
在本文中,你将学习如何在 Golang 中执行 shell 或外部命令。
在 Go 中使用 os/exec 包执行 Shell 命令
任何操作系统或系统命令都可以使用 Go 的 os/exec 包触发。它提供了两个可用于执行此操作的函数:创建 cmd
对象的命令和执行命令并返回标准输出的输出。
package main
import (
"fmt"
"log"
"os/exec"
)
func main() {
out, err := exec.Command("pwd").Output()
if err != nil {
log.Fatal(err)
}
fmt.Println(string(out))
}
输出:
It will return the location of your current working directory.
现在,让我们看看我们如何使用 os/exec 包来执行像 ls
这样的简单命令。
首先,我们必须导入三个关键包,即 fmt、os/exec 和 runtime。之后,我们将构造一个开始运行代码的 execute()
方法。
package main
import (
"fmt"
"os/exec"
"runtime"
)
func execute() {
out, err := exec.Command("ls").Output()
if err != nil {
fmt.Printf("%s", err)
}
fmt.Println("Program executed")
output := string(out[:])
fmt.Println(output)
}
func main() {
if runtime.GOOS == "windows" {
fmt.Println("This program is not aplicable for windows machine.")
} else {
execute()
}
}
输出:
Program executed
bin
dev
etc
home
lib
lib64
proc
root
sys
tmp
tmpfs
usr
var
在 Go 中使用带参数的 os/exec 包执行 Shell 命令
Command
函数返回一个 Cmd
结构,该结构可以使用指定的参数运行提供的程序。第一个参数是要执行的程序;其他参数是程序参数。
package main
import (
"bytes"
"fmt"
"log"
"os/exec"
"strings"
)
func main() {
cmd := exec.Command("tr", "a-z", "A-Z")
cmd.Stdin = strings.NewReader("Hello Boss!")
var out bytes.Buffer
cmd.Stdout = &out
err := cmd.Run()
if err != nil {
log.Fatal(err)
}
fmt.Printf("New Message: %q\n", out.String())
}
输出:
New Message: "HELLO BOSS!"
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe