在 C# 中将对象序列化为 XML
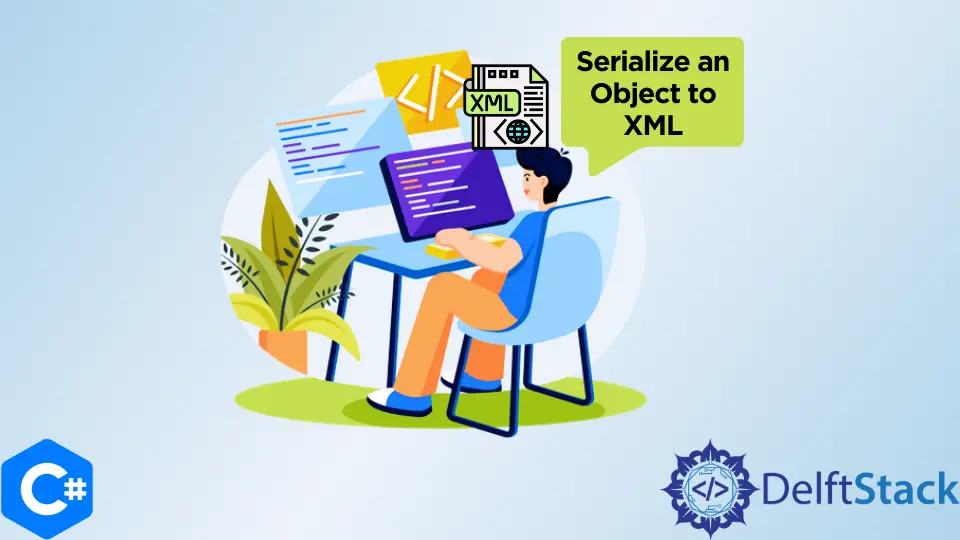
本教程将讨论如何在 C# 中将类对象序列化为 XML 文件或字符串。
使用 C# 中的 XmlSerializer
类将对象序列化为 XML
序列化意味着将类对象转换为 XML 或二进制格式。XmlSerializer
类在 C# 中将类对象转换为 XML,反之亦然。XmlSerializer.Serialize()
方法将类对象的所有公共字段和属性转换为 XML 格式。如果我们想将其写入 XML 文件或字符串,则需要使用 public
访问说明符来定义我们的类。以下代码示例向我们展示了如何使用 C# 中的 XmlSerializer
类将类对象序列化为 XML 字符串变量。
using System;
using System.IO;
using System.Linq;
using System.Xml;
using System.Xml.Serialization;
namespace serialize_object {
public class Sample {
public string value { get; set; }
}
class Program {
static void Main(string[] args) {
Sample obj = new Sample();
obj.value = "this is some value";
string xml = "";
XmlSerializer serializer = new XmlSerializer(typeof(Sample));
using (var sww = new StringWriter()) {
using (XmlWriter writer = XmlWriter.Create(sww)) {
serializer.Serialize(writer, obj);
xml = sww.ToString(); // Your XML
}
}
Console.WriteLine(xml);
}
}
}
输出:
<?xml version="1.0" encoding="utf-16"?><Sample xmlns:xsi="https://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="https://www.w3.org/2001/XMLSchema"><value>this is some value</value></Sample>
在上面的代码中,我们使用 C# 中的 XmlSerializer.Serialize()
函数将 Sample
类的对象 obj
序列化为 XML 字符串变量 xml
。我们首先通过传递 typeof(Sample)
参数来初始化 XmlSerializer
类的实例。这将创建类型为样本
的 XmlSerializer
类的实例。然后,我们创建了 StringWriter
类的实例,以将数据写入字符串变量。然后,我们创建了 XmlWriter
类的实例以写入 StringWriter
类的实例。然后,我们使用 serializer.Serialize(writer, obj)
将对象写入 StringWriter
类的实例,并使用 ToString()
函数将结果存储到字符串变量 xml
中。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn