在 C++ 中从向量中删除重复项
Jinku Hu
2023年10月12日
C++
C++ Vector
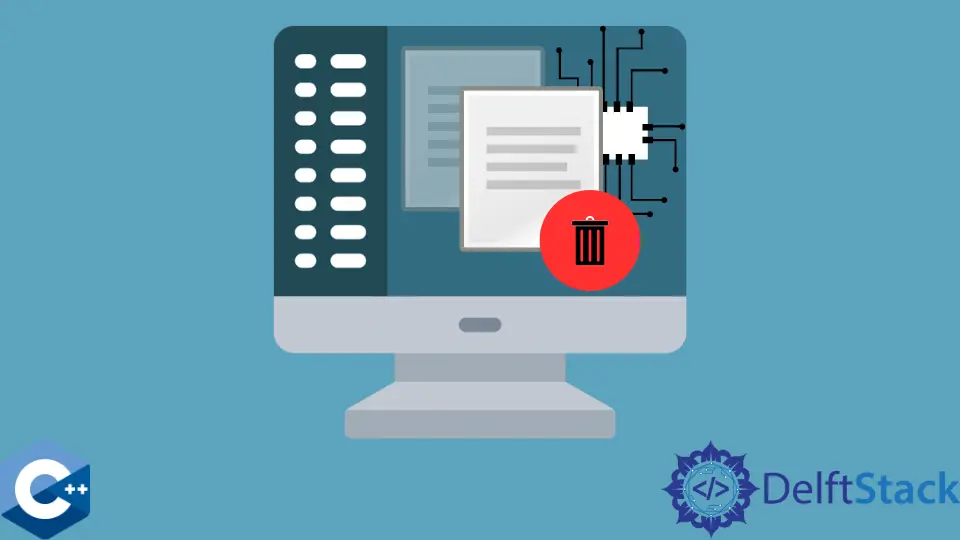
本文将介绍如何在 C++ 中去除向量中的重复项。
使用 std::unique
和 std::vector::erase
函数从 C++ 向量中删除重复项
std::unique
函数是 STL 算法的一部分,它本质上实现了对排序范围的重复元素删除操作。每次删除后元素都会移动,并且该函数返回新形成的范围的最后迭代器。我们在以下示例代码中使用的 std::unique
重载采用两个迭代器参数来表示范围的开始和结束。在这种情况下,我们还使用 std::sort
算法对给定的 vector
进行排序,然后再使用 std::unique
对其进行处理。最后,调用 erase
成员函数来修改原始数组大小以适应新形成的向量。
#include <algorithm>
#include <iomanip>
#include <iostream>
#include <iterator>
#include <vector>
using std::copy;
using std::cout;
using std::endl;
using std::left;
using std::setw;
using std::vector;
int main() {
vector<int> int_vec = {10, 23, 10, 324, 10, 10, 424,
649, 110, 110, 129, 40, 424};
cout << left << setw(10) << "vec: ";
copy(int_vec.begin(), int_vec.end(), std::ostream_iterator<int>(cout, "; "));
cout << endl;
std::sort(int_vec.begin(), int_vec.end());
auto last = std::unique(int_vec.begin(), int_vec.end());
int_vec.erase(last, int_vec.end());
cout << left << setw(10) << "vec: ";
copy(int_vec.begin(), int_vec.end(), std::ostream_iterator<int>(cout, "; "));
cout << endl;
return EXIT_SUCCESS;
}
输出:
vec: 10; 23; 10; 324; 10; 10; 424; 649; 110; 110; 129; 40; 424;
vec: 10; 23; 40; 110; 129; 324; 424; 649;
实现上述解决方案的另一种方法是使用 resize
函数而不是 erase
调用。resize
函数修改向量的元素计数。因此,我们可以使用 distance
调用传递新形成的向量元素的计算计数,而 resize
将缩小向量。
#include <algorithm>
#include <iomanip>
#include <iostream>
#include <iterator>
#include <vector>
using std::copy;
using std::cout;
using std::endl;
using std::left;
using std::setw;
using std::vector;
int main() {
vector<int> int_vec = {10, 23, 10, 324, 10, 10, 424,
649, 110, 110, 129, 40, 424};
cout << left << setw(10) << "vec: ";
copy(int_vec.begin(), int_vec.end(), std::ostream_iterator<int>(cout, "; "));
cout << endl;
std::sort(int_vec.begin(), int_vec.end());
auto last = std::unique(int_vec.begin(), int_vec.end());
int_vec.resize(std::distance(int_vec.begin(), last));
cout << left << setw(10) << "vec: ";
copy(int_vec.begin(), int_vec.end(), std::ostream_iterator<int>(cout, "; "));
cout << endl;
return EXIT_SUCCESS;
}
输出:
vec: 10; 23; 10; 324; 10; 10; 424; 649; 110; 110; 129; 40; 424;
vec: 10; 23; 40; 110; 129; 324; 424; 649;
使用 std::set
容器从 C++ 向量中删除重复项
或者,std::set
容器可用于从向量中删除重复元素。请注意,std::set
在内部存储给定类型的唯一对象,因此我们需要从向量元素构造一个。一旦 set
用需要排序的向量元素初始化,我们可以从原始 vector
对象调用 assign
函数来存储来自 set
对象的唯一元素。
#include <algorithm>
#include <iomanip>
#include <iostream>
#include <iterator>
#include <set>
#include <vector>
using std::copy;
using std::cout;
using std::endl;
using std::left;
using std::set;
using std::setw;
using std::vector;
int main() {
vector<int> int_vec = {10, 23, 10, 324, 10, 10, 424,
649, 110, 110, 129, 40, 424};
cout << left << setw(10) << "vec: ";
copy(int_vec.begin(), int_vec.end(), std::ostream_iterator<int>(cout, "; "));
cout << endl;
set<int> int_set(int_vec.begin(), int_vec.end());
int_vec.assign(int_set.begin(), int_set.end());
cout << left << setw(10) << "vec: ";
copy(int_vec.begin(), int_vec.end(), std::ostream_iterator<int>(cout, "; "));
cout << endl;
return EXIT_SUCCESS;
}
输出:
vec: 10; 23; 10; 324; 10; 10; 424; 649; 110; 110; 129; 40; 424;
vec: 10; 23; 40; 110; 129; 324; 424; 649;
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu