在 C++ 中打印链接列表
Jinku Hu
2023年10月12日
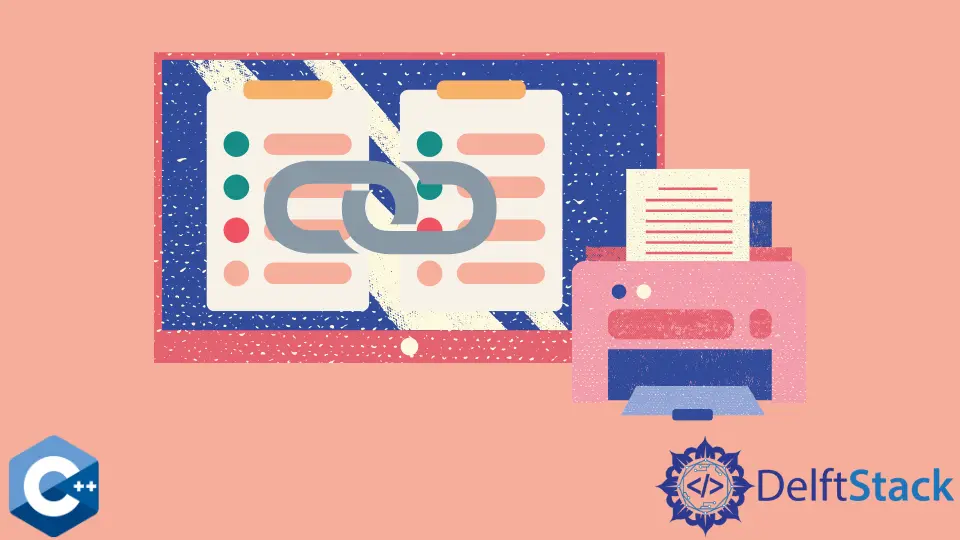
本文将介绍几种在 C++ 中打印链接列表元素的方法。
使用自定义函数打印链接列表中的元素
在下面的例子中,我们手动构建一个链接列表数据结构,用任意值初始化它,然后将元素打印到控制台。实现的结构是一个单一的链接列表,有三个数据成员,分别称为 city
、country
和 key
。
函数 addNewNode
用于在链接列表中构造一个新元素。它以 Node*
参数作为构建新节点的地址,以及需要为其数据成员分配的 3 个对应值。
由于我们是手动构建数据结构,我们需要利用动态内存分配。因此,在程序退出之前,需要另一个函数 freeNodes
来释放链表。
一旦完成了链接列表的初始化,我们就可以在循环中调用 printNodeData
函数来打印从 vector
对中推送到列表中的相同数量的元素。该函数只取类型为 Node*
的参数,并调用 cout
将每个数据成员输出到控制台。这个函数的缺点是,用户每次需要打印元素时,都需要担心是否正确迭代到链接列表中。
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::pair;
using std::string;
using std::vector;
struct Node {
struct Node *next{};
string city;
string country;
int key{};
};
struct Node *addNewNode(struct Node *node, int key, string &city,
string &country) {
node->next = new Node;
node->next->key = key;
node->next->city = city;
node->next->country = country;
return node;
}
void freeNodes(struct Node *node) {
struct Node *tmp = nullptr;
while (node) {
tmp = node;
node = node->next;
delete tmp;
}
}
void printNodeData(struct Node *node) {
cout << "key: " << node->key << endl
<< "city: " << node->city << endl
<< "county: " << node->country << endl
<< endl;
}
int main() {
struct Node *tmp, *root;
struct Node *end = nullptr;
vector<pair<string, string>> list = {{"Tokyo", "Japan"},
{"New York", "United States"},
{"Mexico City", "Mexico"},
{"Tangshan", "China"},
{"Tainan", "Taiwan"}};
root = new Node;
tmp = root;
for (int i = 0; i < list.size(); ++i) {
tmp = addNewNode(tmp, i + 1, list[i].first, list[i].second);
tmp = tmp->next;
}
tmp = root->next;
for (const auto &item : list) {
printNodeData(tmp);
tmp = tmp->next;
}
freeNodes(root->next);
delete root;
return EXIT_SUCCESS;
}
输出:
key: 1
city: Tokyo
county: Japan
...
使用自定义的函数打印链接列表中的所有元素
print
函数更好的实现是只调用一次的函数。printNodes
函数被定义为 void
类型,它不向调用者返回任何东西。它正好需要一个类型为 Node*
的参数,类似于前面的函数,它自己在链接列表中进行迭代。注意,调用 freeNodes
函数还不足以清理数据结构使用的所有动态内存。从 main
函数中分配的 root
指针也需要释放,否则,内存泄漏将不可避免。
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::pair;
using std::string;
using std::vector;
struct Node {
struct Node *next{};
string city;
string country;
int key{};
};
struct Node *addNewNode(struct Node *node, int key, string &city,
string &country) {
node->next = new Node;
node->next->key = key;
node->next->city = city;
node->next->country = country;
return node;
}
void freeNodes(struct Node *node) {
struct Node *tmp = nullptr;
while (node) {
tmp = node;
node = node->next;
delete tmp;
}
}
void printNodes(struct Node *node) {
while (node) {
cout << "key: " << node->key << endl
<< "city: " << node->city << endl
<< "county: " << node->country << endl
<< endl;
node = node->next;
}
}
int main() {
struct Node *tmp, *root;
struct Node *end = nullptr;
vector<pair<string, string>> list = {{"Tokyo", "Japan"},
{"New York", "United States"},
{"Mexico City", "Mexico"},
{"Tangshan", "China"},
{"Tainan", "Taiwan"}};
root = new Node;
tmp = root;
for (int i = 0; i < list.size(); ++i) {
tmp = addNewNode(tmp, i + 1, list[i].first, list[i].second);
tmp = tmp->next;
}
printNodes(root->next);
freeNodes(root->next);
delete root;
return EXIT_SUCCESS;
}
输出:
key: 1
city: Tokyo
county: Japan
...
作者: Jinku Hu