在 C++ 中使用 cin.fail 方法
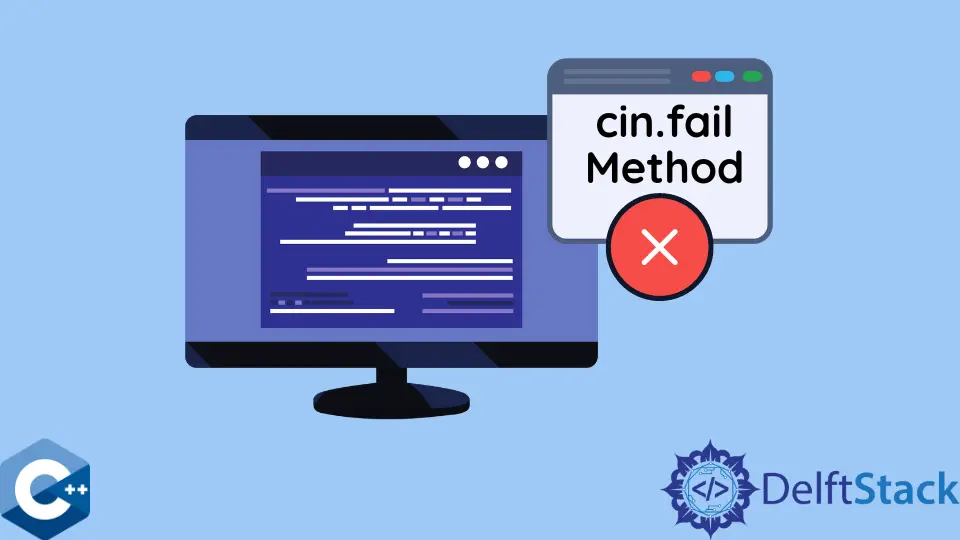
本文将演示在 C++ 中对流对象正确使用 fail
方法的多种方法。
在 C++ 中使用 fail
方法检查流对象是否发生错误
fail
方法是 basic_ios
类的内置函数,可以调用它来验证给定的流是否有错误状态。该函数不接受任何参数,如果流对象发生任何错误,则返回布尔值 true
。注意,流对象有几个常数位来描述它们的当前状态。这些常数是-goodbit
、eofbit
、failbit
和 badbit
。如果设置了 failbit
,意味着操作失败,但流本身处于良好状态。badbit
是在流被破坏时设置的。如果给定的流对象上设置了 badbit
或 failbit
,fail
方法返回 true
。
#include <fstream>
#include <iostream>
#include <sstream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::ifstream;
using std::ostringstream;
using std::string;
int main() {
string filename("tmp.txt");
string file_contents;
auto ss = ostringstream{};
ifstream input_file(filename);
if (input_file.fail()) {
cerr << "Could not open the file - '" << filename << "'" << endl;
exit(EXIT_FAILURE);
}
ss << input_file.rdbuf();
file_contents = ss.str();
cout << file_contents;
exit(EXIT_SUCCESS);
}
在 C++ 中使用 good
方法检查流对象是否发生错误
另外,也可以调用 good
内置函数来检查流对象是否处于良好状态。由于 goodbit
常量在流状态下被设置,意味着其他每一个位都被清除,检查它意味着流的良好状态。注意,这些常量是在类 ios_base
中定义的,可以称为 std::ios_base::goodbit
。下面的代码示例显示,可以指定 good
方法返回值的逻辑否定作为检查流上任何故障的条件。
#include <fstream>
#include <iostream>
#include <sstream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::ifstream;
using std::ostringstream;
using std::string;
int main() {
string filename("tmp.txt");
string file_contents;
auto ss = ostringstream{};
ifstream input_file(filename);
if (!input_file.good()) {
cerr << "Could not open the file - '" << filename << "'" << endl;
exit(EXIT_FAILURE);
}
ss << input_file.rdbuf();
file_contents = ss.str();
cout << file_contents;
exit(EXIT_SUCCESS);
}
在 C++ 中使用流对象作为表达式来检查是否发生错误
前面的方法 - fail
和 good
是用现代 C++ 风格评估流错误的推荐方法,因为它使代码更易读。我们也可以将流对象本身指定为一个表达式来检查是否有任何错误发生。后一种方法比较神秘,但却是实现错误检查条件语句的正确方法。
#include <fstream>
#include <iostream>
#include <sstream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::ostringstream;
using std::string;
int main() {
string filename("tmp.txt");
string file_contents;
auto ss = ostringstream{};
ifstream input_file(filename);
if (!input_file) {
cerr << "Could not open the file - '" << filename << "'" << endl;
exit(EXIT_FAILURE);
}
ss << input_file.rdbuf();
file_contents = ss.str();
cout << file_contents;
exit(EXIT_SUCCESS);
}
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu