React 中具有預設值的可選道具
Shuvayan Ghosh Dastidar
2023年1月30日
TypeScript
TypeScript Props
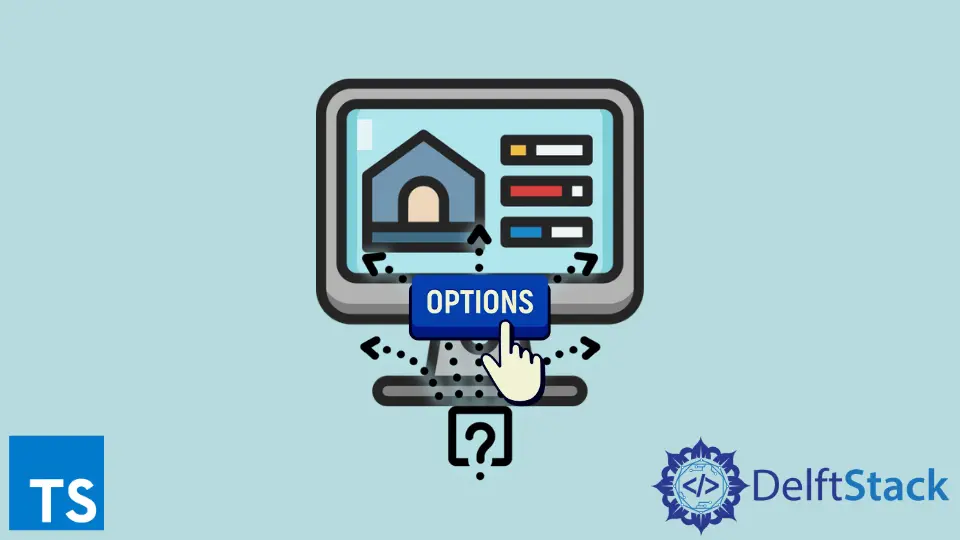
React 中的 Props 允許我們在不同的元件之間傳遞資料和引數,這些資料和引數可以流過 React 中的整個元件樹。傳遞給不同元件的 props 可以具有定義為基本型別或使用者定義型別的固定型別,就像在 TypeScript 中一樣。
本教程將演示如何將 React 的可選 props 與預設值一起使用。
TypeScript 中的可選道具
在 TypeScript 中,可以使用 ?
將型別或道具設為可選操作符。這個事實可以用來在 React 中傳遞可選引數。
interface OptionalComponentProps {
color? : string;
title : string;
size? : number;
}
在上面的示例中,包含特定元件的 props 的型別定義,color
和 size
屬性被定義為可選的。
interface Props {
color? : string;
size: number;
title? : string;
}
function Card(props : Props){
const { color , size, title} = props;
return (
<div>
<div>{color}</div>
<div>{size}</div>
<div>{title}</div>
</div>
)
}
function App() {
return (
<div>
<Card size={20} title="Cool card" />
</div>
)
}
因此在上面的例子中,父元件是 App
,Props
型別的 props 被傳遞給 Card
元件。color
和 title
屬性由 ?
標記為可選操作符。
如果可選屬性沒有作為 prop 傳遞給元件,它會被初始化為 undefined
,因此不會顯示或呈現。
TypeScript 中具有預設值的可選道具
上面的示例也可以與元件提供的預設值一起使用。
interface Props {
color? : string;
size: number;
title? : string;
}
function Card(props : Props){
const { color = 'red' , size, title = 'Default title'} = props;
return (
<div>
<div>{color}</div>
<div>{size}</div>
<div>{title}</div>
</div>
)
}
function App() {
return (
<div>
<Card size={20} title="Cool card" />
</div>
)
}
早些時候,color
屬性沒有被渲染,因為沒有預設值,但現在因為沒有值作為 props 傳遞,所以使用預設值來渲染元件。但是,沒有使用 title
屬性的預設值,因為它被傳遞給元件的 prop 覆蓋。
在 React 中使用 defaultProps
屬性來設定 Props 的預設值
React 支援為使用 defaultProps
屬性傳遞的道具分配預設值。渲染元件時可以直接分配和使用預設值。
interface Props {
color? : string;
size: number;
title? : string;
}
const defaultProps : Props = {
color :"red",
size : 20,
title : "A cool title"
}
function Card(props : Props){
return (
<div>
<div>{props.color}</div>
<div>{props.size}</div>
<div>{props.title}</div>
</div>
)
}
Card.defaultProps = defaultProps;
function App() {
return (
<div>
<Card size={20} title="Cool card" />
</div>
)
}
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe