在 TypeScript 中檢查 Null 和 Undefined
-
使用可選鏈檢查 TypeScript 中的
null
和undefined
-
在 TypeScript 中使用 Nullish Coalescing 檢查
null
和undefined
-
在 TypeScript 中使用
==
和===
運算子檢查null
和undefined
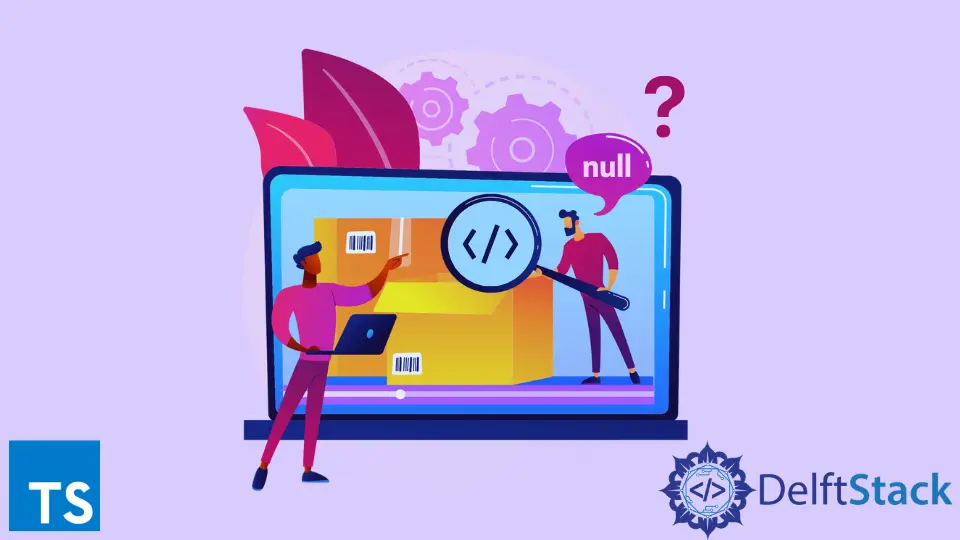
TypeScript 對 null
和 undefined
進行了類似的定義。在程式設計中,null
是指某個為空或不存在的物件,undefined
是指宣告為沒有賦值的變數。
我們可以使用幾種不同的方法來識別 null
或 undefined
,但它們都不包含直接指定 null
或 undefined
值的直接函式或語法。
從 TypeScript 3.7 開始,有一種方法可以使用 Nullish Coalescing、可選鏈以及 ==
和 ===
運算子來識別 null
或 undefined
值。
本文將討論在 TypeScript 中檢查 null
和 undefined
的方法。
使用可選鏈檢查 TypeScript 中的 null
和 undefined
可選連結 (?.
) 是一個運算子,如果遇到 null
或 undefined
,我們可以使用它來終止程式碼執行。因此,當它識別出 null
或 undefined
時,它將簡單地返回 undefined
作為輸出。
參考下面的例子。
const obj =
{
branch1: {
emp1: {
name : "Rory",
age : 25
}
}
}
console.log(obj.branch1.emp1.name)
輸出:
Rory
當 obj
下沒有提及 emp1
時,將返回錯誤訊息。但是,如果我們使用可選連結 (?.
),它將返回 undefined
作為輸出。
const obj =
{
branch1: {
}
}
console.log(obj.branch1?.emp1?.name)
輸出:
Undefined
如果 obj.branch.emp1
不存在,運算子告訴不執行任何操作,而是返回 undefined
。
在這樣的情況下,
console.log(obj.branch1?.emp1.name)
它說當 emp1
元素不存在時訪問 name
元素;它將返回錯誤訊息而不是 undefined
。因此,我們應該手動將 (?.
) 運算子新增到元素中;否則,將返回錯誤。
因此,可以在此方法中檢查 undefined
。
此外,可選連結減少了程式碼並使訪問元素而不是使用&&
更有效。它以更少的程式碼返回元素(如果存在)。
在 TypeScript 中使用 Nullish Coalescing 檢查 null
和 undefined
Nullish Coalescing (??
) 是另一種識別 null
和 undefined
值的方法。當與 null
和 undefined
相對時,它將返回預設值。
在某些情況下,零或空字串是應該使用的實際值,但是當||
被使用,它不會返回那些值。因此,使用 Nullish Coalescing (??
) 運算子,可以返回這些值。
因此||
可以換成 ??
當使用預設值時。
下面是一些帶有 (??
) 的 Nullish Coalescing 應用程式。
null ?? "Value";
// "Value"
undefined ?? "Value";
// "Value"
false ?? true;
// false
0 ?? 100;
// 0
"" ?? "n/a";
// ""
NaN ?? 0;
// NaN
在 TypeScript 中使用 ==
和 ===
運算子檢查 null
和 undefined
在 TypeScript 中可以同時使用 ==
和 ===
來執行 null
和 undefined
檢查。
當 ===
運算子用於通過嚴格檢查方法驗證變數時,它將檢查變數的型別和變數值的型別,並執行嚴格的 undefined
檢查。
此外,我們可以使用 ==
在 TypeScript 中執行 undefined
檢查。在嚴格檢查方法中使用 ==
時,它只會檢查值的型別,這與 ===
運算子不同。
==
運算子可以使用嚴格檢查方法進行 null
檢查。如果變數為 null
或什至是 undefined
,它將返回 true
。
只能使用 ==
運算子對 null
執行嚴格檢查。在 TypeScript 中,我們可以按照 juggling-check 方法同時檢查 null
和 undefined
。
例子:
var var1: number;
var var2: number = null;
function typecheck(x, name) {
if (x == null) {
console.log(name + ' == null');
}
if (x === null) {
console.log(name + ' === null');
}
if (typeof x === 'undefined') {
console.log(name + ' is undefined');
}
}
typecheck(var1, 'var1');
typecheck(var2, 'var2');
輸出:
"var1 == null"
"var1 is undefined"
"var2 == null"
"var2 === null"
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.