Scala 中的 Forall() 函式
-
在 Scala 中使用
forall()
函式迭代集合 -
在 Scala 中使用
forall()
函式處理一個空集合 -
在 Scala 中使用
forall()
和HashMap
-
在 Scala 中使用
forall()
和getOrElse()
函式 -
Scala 中
foreach
和forall
的區別 - まとめ
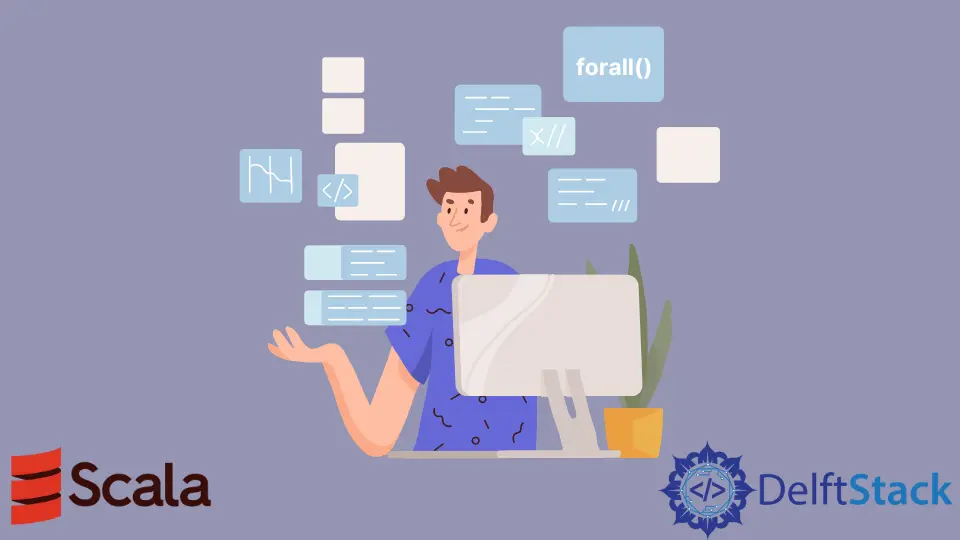
本文將展示 Scala 的 forall()
函式並檢視各種示例以瞭解其用例。
Scala 的 forall()
函式用於在元素集合上應用謂詞邏輯。它用於檢查我們應用的謂詞邏輯是否滿足所有集合元素。
語法:
trait Collection[T] {
def forall(p: (T) => Boolean): Boolean
}
forall()
函式檢查集合的所有元素是否滿足謂詞 p
並相應地返回真或假,因為它具有布林返回型別。讓我們看一些將謂詞邏輯應用於元素集合的例項。
在 Scala 中使用 forall()
函式迭代集合
下面的示例顯示了一個片段,其中 forall
與列表一起使用。
示例程式碼:
object workspace {
def main(args:Array[String])
{
val studentMarks = List(55, 60, 20, 90, 80)
//applyaing forall method on list
val result = studentMarks.forall(y => y>=50)
if(result)
{
println("Student passed the examination")
}
else
println("Student has failed the examination")
}
}
輸出:
Student has failed the examination
邏輯是列表 studentMarks
內的元素應該大於或等於 50。由於 20 小於 50,所以返回 else
語句並儲存在結果變數中。
之後,我們使用 if
條件檢查結果並相應地列印輸出。
在 Scala 中使用 forall()
函式處理一個空集合
forall
方法在處理空集合時總是返回 true
,因為列表中的任何元素都沒有違反謂詞邏輯。如果列表中沒有元素,則謂詞邏輯永遠不會為假。
示例程式碼:
object workspace {
def main(args:Array[String])
{
val studentMarks = List[Int]()
//applyaing forall method on list
val result = studentMarks.forall(y=>y>1)
if(result)
{
println("forall returns true")
}
else
println("forall returns false")
}
}
輸出:
forall returns true
在 Scala 中使用 forall()
和 HashMap
forall
在這裡非常有用。每當我們想對 HashMap 的所有鍵應用某個條件或檢查所有鍵值對是否滿足某個條件時,我們可以使用 forall() 函式。
示例程式碼:
import scala.collection.mutable
object workspace {
def main(args: Array[String]): Unit = {
val mp = mutable.HashMap[Int, Int](1 -> 100, 2 -> 200, 3->300)
val allValuesHunderedTimeTheKey = mp.forall { case (key, value) => key * 100 == value }
println(allValuesHunderedTimeTheKey)
}
}
輸出:
true
我們使用 forall
檢查每個值是否是鍵值的 100 倍。由於所有元素都滿足謂詞,因此返回 true
。
在 Scala 中使用 forall()
和 getOrElse()
函式
示例程式碼:
object workspace {
def main(args: Array[String]): Unit = {
val names : Option[Seq[String]] = Option(Seq("Iron man", "Tony", "Stark"))
println(names.map(_.forall(Seq("Bruce").contains)).getOrElse(false))
}
}
輸出:
false
map
已將 Option
內的序列建立為布林值,然後 getOrElse
提供最終結果,提供預設布林值。
Scala 中 foreach
和 forall
的區別
foreach
經常被初學者與 forall
混淆。讓我們看看它們之間的區別。
foreach
函式允許我們遍歷元素的集合。forall
函式採用布林謂詞,檢查所有元素,如果元素滿足條件,則返回true
。
示例程式碼:
object workspace {
def main(args: Array[String]): Unit = {
val studentMarks = List(55, 60, 20, 90, 80)
//applyaing forall method on list
val result = studentMarks.forall(y => y>=50)
println(result)
studentMarks.foreach(x => println(2*x))
}
}
輸出:
false
110
120
40
180
160
使用 forall()
,我們正在檢查每個元素是否大於或等於 50,它返回 false
,因為元素 20 不符合條件並使用 foreach()
,我們正在迭代所有元素並僅列印該元素的雙倍。
まとめ
在本文中,我們瞭解了 Scala 的 forall()
函式及其在各種條件下將謂詞邏輯應用於元素集合的用途。