在 Scala 中獲取當前日期和時間
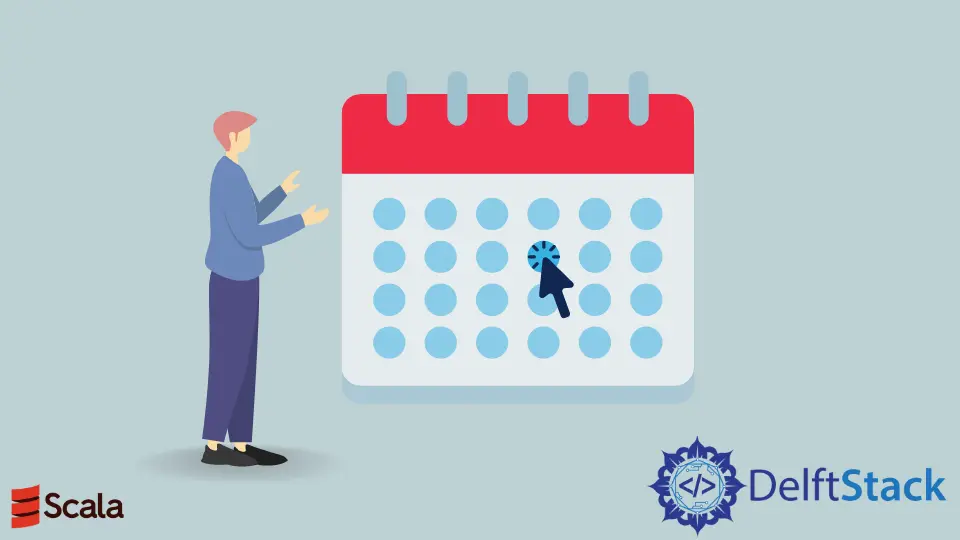
本文將演示如何在 Scala 中獲取當前日期和時間。Scala 是一種基於 JVM 的通用程式語言,就像這裡的任何通用程式設計一樣,日期和時間起著非常重要的作用。
與 Java 不同,Scala 沒有本地日期時間庫。但是我們在 Scala 中有一些庫,它們是著名的 Java 庫(如 Joda-Time)的包裝器。
使用這些庫,我們可以獲得 Scala 富有表現力的編碼風格的優雅以及底層 Java 庫的強大功能。我們還可以在 Scala 中使用本機 Java 日期和時間庫。
在 Scala 中使用 Nscala-Time 庫獲取當前日期和時間
在 Scala 中,Nscala-Time 庫是 Joda-Time 的包裝庫。這也使許多運算子也可以執行日期時間算術。
要使用它,我們必須在我們的程式碼檔案中匯入 com.github.nscala_time.time.Imports._
和 org.joda.time.Days
。
讓我們看下面的例子:
val start:DateTime = DateTime.now()
val end:DateTime = x + (1.hours + 10.minutes + 5.seconds)
val elapsed:Interval = start to end
println(elapsed.toPeriod.toString(PeriodFormat.getDefault))
在 Scala 中使用 Java Calendar
類獲取當前日期和時間
使用經典的 Java Calendar
類,我們可以在 Scala 中獲取當前日期和時間。這是一個用於獲取當前日期和時間的 Abstract
類;同樣的類也可以在 Scala 中使用。
僅使用 getInstance()
方法獲取當前時間
示例程式碼:
import java.util.Calendar;
object myClass {
def main(args: Array[String])
{
var date = Calendar.getInstance()
var curr_Minute = date.get(Calendar.MINUTE)
var curr_Hour = date.get(Calendar.HOUR_OF_DAY)
if(curr_Hour > 12)
{
curr_Hour = curr_Hour % 12
println("Current time is "+curr_Hour+":"+curr_Minute+" PM")
}
else{
println("Current time is "+curr_Hour+":"+curr_Minute+" AM")
}
}
}
輸出:
Current time is 11:12 AM
解釋:
在上面的程式碼中,Calendar
類的 getInstance()
方法用於查詢時間。我們使用了 calendar.MINUTE
和 calendar.HOUR_OF_DAY
並將結果分別儲存在變數 curr_Minute
和 curr_Hour
中。
calendar.HOUR_OF_DAY
方法以 24 小時格式返回輸出,我們使用條件語句將其轉換為 12 小時格式。
使用 getTime()
方法獲取完整的當前日期和時間
示例程式碼:
import java.util.Calendar;
object myClass {
def main(args: Array[String]) {
var date = Calendar.getInstance()
var currentHour = date.getTime()
println("Current date and time is "+currentHour)
}
}
輸出:
Current date and time is Fri Jun 03 11:25:16 GMT 2022
解釋:
在上面的程式碼中,我們使用了 Calendar
類的內建方法 getTime()
方法。它以 MM DD time UTC YYYY
返回當前日期和時間。
僅使用 LocalDate
獲取當前日期
示例程式碼:
import java.util.Calendar;
object myClass {
def main(args: Array[String]) {
println(java.time.LocalDate.now)
}
}
輸出:
2022-06-03
解釋:
我們使用 LocalDate.now
來獲取當前日期。我們還可以使用 SimpleDateFormat
類和 Calendar
類將日期格式化為我們選擇的特定格式。
我們必須在我們的程式碼中匯入 java.text.SimpleDateFormat
才能使用它。
使用 getTime()
方法獲取格式化的當前日期
示例程式碼:
import java.util.Calendar;
import java.text.SimpleDateFormat;
object myClass
{
def main(args: Array[String])
{
val ourform = new SimpleDateFormat("dd / MM / yy");
val temp = Calendar.getInstance();
println("current Date is : " + temp.getTime());
val formatted_Date = ourform.format(temp.getTime());
println("Formatted date is: "+formatted_Date);
}
}
輸出:
current Date is : Fri Jun 03 11:45:35 GMT 2022
Formatted date is: 03 / 06 / 22