在 Python 中從字串中刪除某些字元
Muhammad Waiz Khan
2023年10月10日
Python
Python String
-
在 Python 中使用
string.replace()
方法從字串中刪除某些字元 -
在 Python 中使用
string.join()
方法從字串中刪除某些字元 -
在 Python 中使用
re.sub()
方法從字串中刪除某些字元
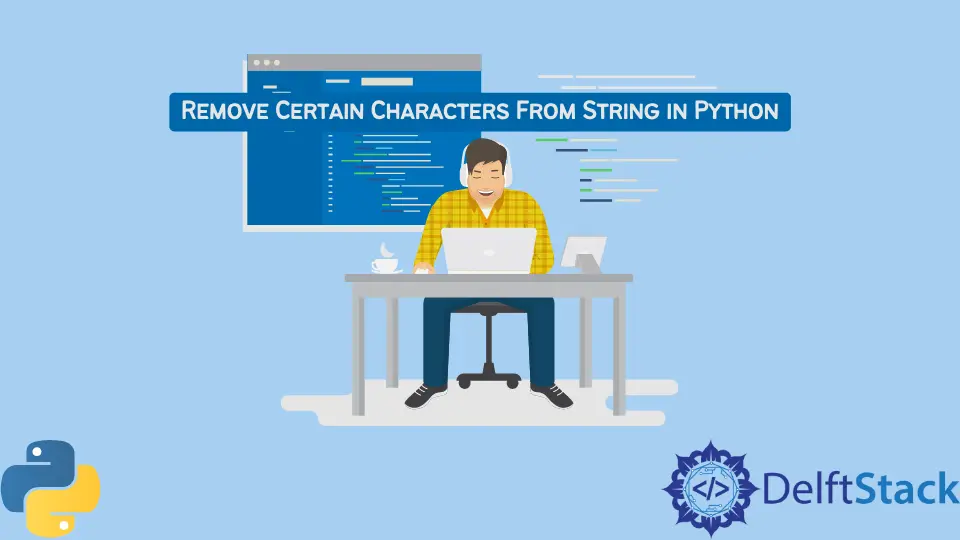
本教程將解釋在 Python 中從字串中刪除某些字元的各種方法。在許多情況下,我們需要從文字中刪除標點符號或某個特殊字元,比如為了資料清理。
在 Python 中使用 string.replace()
方法從字串中刪除某些字元
string.replace()
方法在將第一個字串引數替換為第二個字串引數後返回一個新的字串。要使用 string.replace()
方法從字串中刪除某些字元,我們可以使用 for
迴圈從一個字串中每次迭代刪除一個字元。
由於我們要刪除字元而不是替換它們,我們將傳遞一個空字串作為第二個引數。下面的示例程式碼演示瞭如何使用 string.replace()
方法從字串中刪除字元。
string = "Hey! What's up?"
characters = "'!?"
for x in range(len(characters)):
string = string.replace(characters[x], "")
print(string)
輸出:
Hey Whats up
在 Python 中使用 string.join()
方法從字串中刪除某些字元
string.join(iterable)
方法將可迭代物件的每個元素與 string
連線起來,並返回一個新的字串。要使用 string.join()
方法從字串中刪除某些字元,我們必須遍歷整個字串,並從字串中刪除我們需要刪除的字元。下面的示例程式碼演示了我們如何在 Python 中使用 string.join()
進行操作。
string = "Hey! What's up?"
characters = "'!?"
string = "".join(x for x in string if x not in characters)
print(string)
輸出:
Hey Whats up
在 Python 中使用 re.sub()
方法從字串中刪除某些字元
re
模組的 re.sub(pattern, repl, string, count)
方法在將正規表示式 pattern
替換為原始字串中的 repl
值後,返回一個新的字串。而 count
是指我們要從字串中替換 pattern
的次數。
由於我們需要刪除而不是替換任何字元,所以 repl
將等於一個空字串。下面的程式碼示例演示了我們如何在 Python 中使用 re.sub()
方法來替換字串中的字元。
import re
string = "Hey! What's up?"
string = re.sub("\!|'|\?", "", string)
print(string)
輸出:
Hey Whats up
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe