Python 中從 URL 獲取資料
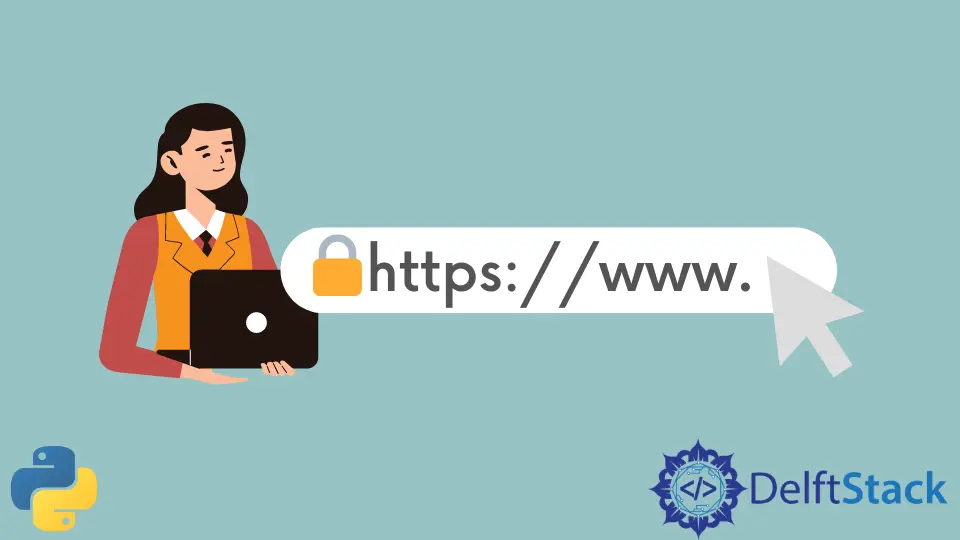
URL 或統一資源定位符是指向 Internet 上某些資源的有效且唯一的 Web 地址。該資源可以是簡單的文字檔案、zip 檔案、exe
檔案、視訊、影象或網頁。
在網頁的情況下,獲取 HTML 或超文字標記語言內容。本文將展示如何使用 Python 從 URL 獲取此 HTML 或超文字標記語言資料。
使用 Python 中的 requests
模組從 URL 獲取資料
Python 有一個 requests
模組,可以輕鬆傳送 HTTP(超文字傳輸協議)請求。此模組可用於從有效 URL 中獲取 HTML 內容或任何內容。
requests
模組有一個 get()
方法,我們可以使用它從 URL 中獲取資料。此方法接受一個 url
作為引數並返回一個 requests.Response
物件。
這個 requests.Response
物件包含有關伺服器對傳送的 HTTP 請求的響應的詳細資訊。如果一個無效的 URL 被傳遞給這個 get()
方法,get()
方法將丟擲一個 ConnectionError
異常。
如果你不確定 URL 的有效性,強烈建議使用 try
和 except
塊。只需將 get()
方法呼叫包含在 try
和 except
塊中。這將在接下來的示例中進行描述。
現在,讓我們瞭解如何使用此函式從有效 URL 中獲取 HTML 內容或任何資料。相同的參考下面的程式碼。
要了解有關 requests.Response
物件的更多資訊,請參閱官方文件此處。
import requests
try:
url = "https://www.lipsum.com/feed/html"
r = requests.get(url)
print("HTML:\n", r.text)
except:
print(
"Invalid URL or some error occured while making the GET request to the specified URL"
)
輸出:
HTML:
...
請注意,...
表示從 URL 獲取的 HTML 內容。由於 HTML 內容太大,因此未在上面的輸出中顯示。
如果 URL 錯誤,上面的程式碼將執行 except
塊內的程式碼。下面的程式碼描述了它是如何工作的。
import requests
try:
url = "https://www.thisisafaultyurl.com/faulty/url/"
r = requests.get(url)
print("HTML:\n", r.text)
except:
print(
"Invalid URL or some error occured while making the GET request to the specified URL"
)
輸出:
Invalid URL or some error occurred while making the GET request to the specified URL
出於安全目的,某些網頁不允許 GET
請求獲取其內容。在這種情況下,我們可以使用 requests
模組中的 post()
方法。
顧名思義,此方法將 POST
請求傳送到有效的 URL。該方法接受兩個引數,即 url
和 data
。
url
是目標 URL,而 data
接受鍵值對形式的標題詳細資訊字典。標頭詳細資訊可以是 API 或應用程式程式設計介面金鑰、CSRF 或跨站點請求偽造令牌等。
這種情況下的 Python 程式碼如下。
import requests
try:
url = "https://www.thisisaurl.com/that/accepts/post/requests/"
payload = {
"api-key": "my-api-key",
# more key-value pairs
}
r = requests.post(url, data=payload)
print("HTML:\n", r.text)
except:
print(
"Invalid URL or some error occured while making the POST request to the specified URL"
)