Python 中捕獲鍵盤中斷錯誤
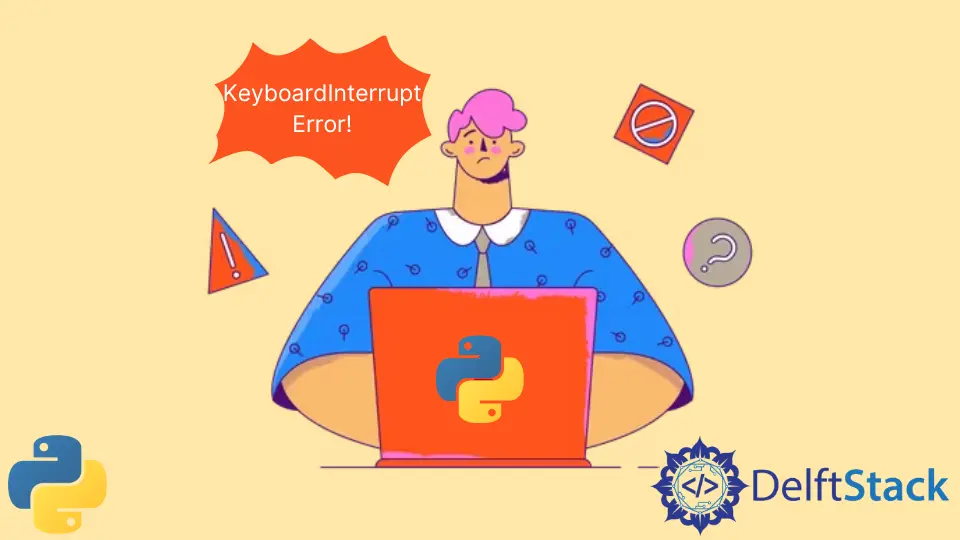
當使用者使用 Ctrl + C 或 Ctrl + Z 手動嘗試停止正在執行的程式時,或在 Jupyter Notebook 的情況下通過中斷核心,會發生 KeyboardInterrupt
錯誤。為了防止經常發生的 KeyboardInterrupt
的意外使用,我們可以在 Python 中使用異常處理。
在本指南中,你將學習如何在 Python 中捕獲 KeyboardInterrupt
錯誤。
使用 try...except
語句捕獲 Python 中的 KeyboardInterrupt
錯誤
try...except
語句用於 Python 中的異常處理目的。try...except
語句具有獨特的語法;它分為三個塊,所有這些塊在 Python 程式碼中都有不同的用途和功能。
try
塊包含直譯器必須檢查任何錯誤的程式碼群。except
塊用於新增所需的異常並繞過程式碼錯誤。finally
塊包含需要在不檢查的情況下執行並被try
和except
塊忽略的語句。
為了解釋 Python 中 KeyboardInterrupt
的程式碼,我們採用一個簡單的程式,在手動處理 KeyboardInterrupt
異常的同時要求使用者輸入。
以下程式碼使用 try...except
語句來捕獲 Python 中的 KeyboardInterrupt
錯誤。
try:
x = input()
print("Try using KeyboardInterrupt")
except KeyboardInterrupt:
print("KeyboardInterrupt exception is caught")
else:
print("No exceptions are caught")
上面的程式提供了以下輸出。
KeyboardInterrupt exception is caught
在上面的程式碼中,輸入函式位於 try
塊之間,並留空,因為在這種情況下不需要進一步的細節。然後,except
塊處理 KeyboardInterrupt
錯誤。KeyboardInterrupt
錯誤是手動引發的,以便我們可以識別何時發生 KeyboardInterrupt
程序。
Python 允許在一段程式碼中定義儘可能多的 except
塊。
在 Python 中使用訊號處理程式捕獲的 KeyboardInterrupt
錯誤
signal
模組用於提供在 Python 中使用訊號處理程式的功能和機制。我們可以捕捉到 SIGINT
訊號,它基本上是來自鍵盤 Ctrl+C 的中斷。發生這種情況時,提高 KeyboardInterrupt
是預設操作。
Python 中的 sys
模組用於提供幾個必要的變數和函式,用於操作 Python 執行時環境的不同部分。
signal
和 sys
模組需要匯入到 Python 程式碼中才能成功使用此方法而不會出現任何錯誤。
以下程式碼使用訊號處理程式來捕獲 Python 中的 KeyboardInterrupt
錯誤。
import signal
import sys
def sigint_handler(signal, frame):
print("KeyboardInterrupt is caught")
sys.exit(0)
signal.signal(signal.SIGINT, sigint_handler)
上面的程式碼提供了以下輸出。
KeyboardInterrupt is caught
在上面的程式碼中,signal.signal()
函式用於定義在接收到某種型別的訊號時要執行的自定義處理程式。
我們應該注意,一旦為特定訊號設定了一個處理程式,它就會一直保持安裝狀態,直到使用者手動重置它。在這種情況下,唯一的例外是 SIGCHLD
的處理程式。
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn