如何在 Python 中逐行讀取一個檔案到列表
Jinku Hu
2023年1月30日
-
在 Python 中用
readlines
逐行讀取檔案 - 在 Python 中遍歷檔案方法逐行讀取檔案
-
在 Python 中用
file.read
逐行讀取檔案的方法 - 在 Python 中逐行讀取檔案的不同方法的比較
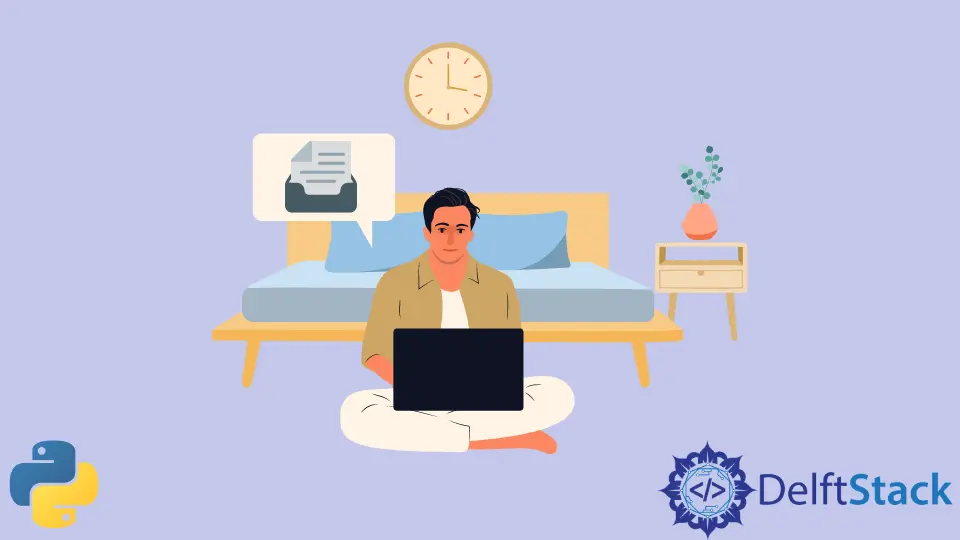
假設我們有一個包含以下內容的檔案,
Line One: 1
Line Two: 2
Line Three: 3
Line Four: 4
Line Five: 5
我們需要逐行將檔案內容讀取到一個列表中 ["Line One: 1", "Line Two: 2", "Line Three: 3", "Line Four: 4", "Line Five: 5"]
。
我們將介紹不同的方法來逐行讀取檔案到下面的列表。
在 Python 中用 readlines
逐行讀取檔案
readlines
返回流中的行列表。
>>> filePath = r"/your/file/path"
>>> with open(filePath, 'r', encoding='utf-8') as f:
f.readlines()
['Line One: 1\n', 'Line Two: 2\n', 'Line Three: 3\n', 'Line Four: 4\n', 'Line Five: 5']
結束符 \n
也包含在字串中,可以用 str.rstrip('\n')
來去除。
>>> with open(filePath, 'r', encoding='utf-8') as f:
[_.rstrip('\n') for _ in f.readlines()]
['Line One: 1', 'Line Two: 2', 'Line Three: 3', 'Line Four: 4', 'Line Five: 5']
在 Python 中遍歷檔案方法逐行讀取檔案
我們可以遍歷檔案以逐行讀取它,而不用 readlines
方法。
>>> with open(filePath, 'r', encoding='utf-8') as f:
[_.rstrip('\n') for _ in f]
['Line One: 1', 'Line Two: 2', 'Line Three: 3', 'Line Four: 4', 'Line Five: 5']
從記憶體使用的角度來看,此方法比上述方法要好得多。readlines
方法將檔案的所有行都儲存在記憶體中,但是遍歷檔案的互動方法僅將檔案內容的一行儲存到記憶體中並進行處理。如果檔案大小過大,遍歷檔案的方法不會出現 readlines
方法中有可能引發的 MemoryError
。
在 Python 中用 file.read
逐行讀取檔案的方法
file.read(size=-1, /)
從檔案中讀取,直到 EOF
假如 size
未設定的話。我們可以使用 str.splitlines
函式從中分割行。
>>> with open(filePath, 'r') as f:
f.read().splitlines()
['Line One: 1', 'Line Two: 2', 'Line Three: 3', 'Line Four: 4', 'Line Five: 5']
在預設 str.splitlines
方法的結果中不包含結尾字元\n
。但是你可以包括 \n
假如將 keepends
引數設定為 True
的話。
>>> with open(filePath, 'r') as f:
f.read().splitlines(keepends=True)
['Line One: 1\n', 'Line Two: 2\n', 'Line Three: 3\n', 'Line Four: 4\n', 'Line Five: 5']
在 Python 中逐行讀取檔案的不同方法的比較
我們將比較本文介紹的不同方法之間的效率表現。我們增加測試檔案中的行數到 8000
以更方便地比較效能差異。
>>> timeit.timeit('''with open(filePath, 'r', encoding='utf-8') as f:
f.readlines()''',
setup='filePath=r"C:\Test\Test.txt"',
number = 10000)
16.36330720000001
>>> timeit.timeit('''with open(filePath, 'r', encoding='utf-8') as f:
[_ for _ in f]''',
setup='filePath=r"C:\Test\Test.txt"',
number = 10000)
18.37279060000003
>>> timeit.timeit('''with open(filePath, 'r', encoding='utf-8') as f:
f.read().splitlines()''',
setup='filePath=r"C:\Test\Test.txt"',
number = 10000)
12.122660100000019
readlines()
方法明顯優於檔案迭代方法,並且 file.read().splitlines()
是最有效的方法,與其他兩種方法相比,效能提升超過 25%。
但是,如果在記憶體受限的大資料 BigData
應用中,檔案迭代方法是最好的方法,原因如上所解釋的那樣。
作者: Jinku Hu