如何在 Python 中讀取 stdin 中的輸入內容
Hassan Saeed
2023年1月30日
Python
Python Input
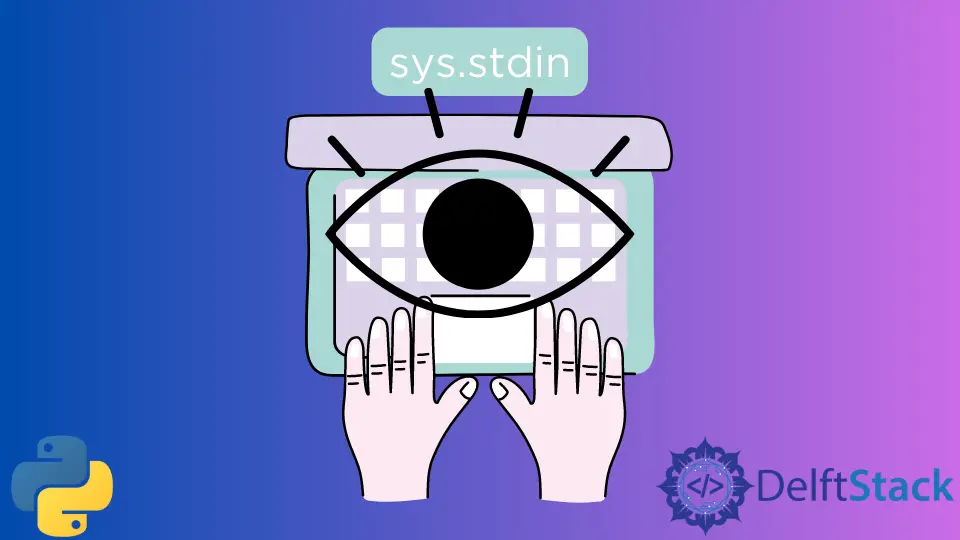
本教程討論了在 Python 中從 stdin
讀取輸入的方法。可以直接從控制檯讀取,也可以從控制檯中指定的檔名讀取。
在 Python 中使用 fileinput.input()
來讀取 stdin
我們可以在 Python 中使用 fileinput
模組來讀取 stdin
。fileinput.input()
讀取命令列引數中指定的輸入檔名的所有行。如果沒有指定引數,它將讀取提供的標準輸入。
下面的例子說明了如何從指定的輸入檔名中讀取。
我們將使用下面的 sample.txt
。
Hello
Line1
Line2
下面是指令碼 read.py
。
import fileinput
for line in fileinput.input():
print(line.rstrip())
我們這樣執行它。
python read.py "sample.txt"
輸出:
Hello
Line1
Line2
下面的例子說明了從標準輸入中讀取資料。
import fileinput
for line in fileinput.input():
print("Output:", line.rstrip())
執行和輸出如下所示。
python read.py
Line 1
Output: Line 1
Line 2
Output: Line2
^Z
我們可以儲存資料以便以後處理,如下所示:
import fileinput
data = []
for line in fileinput.input():
data.append(line.rstrip())
注意,我們使用了 line.rstrip()
. 就是刪除後面的換行。
輸入 y
會刪除所有的變數。
在 Python 中使用 sys.stdin
來讀取 stdin
另一種方法是使用 sys.stdin
來讀取 Python 中的 stdin
。下面的例子說明了從 stdin
逐行讀取資料。
import sys
for line in sys.stdin:
print("Output:", line.rstrip())
執行和輸出如下所示。
python read.py
Line 1
Output: Line 1
Line 2
Output: Line2
^Z
我們也可以一次性從 stdin
中讀取所有資料,而不必逐行讀取。
下面的例子說明了這一點。
import sys
data = sys.stdin.readlines()
data = [line.rstrip() for line in data]
注意我們使用了 line.rstrip()
。這是為了刪除尾部的換行符。
まとめ
我們討論了 Python 中讀取 stdin
輸入的兩種方法,fileinput.input()
和 sys.stdin
。fileinput.input()
可以從命令列引數中指定的檔名或標準輸入中讀取資料,而 sys.stdin
只能從標準輸入中讀取資料。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe