在 Python 中使用 OpenCV 模組繪製矩形
Vaibhav Vaibhav
2024年2月15日
Python
Python OpenCV
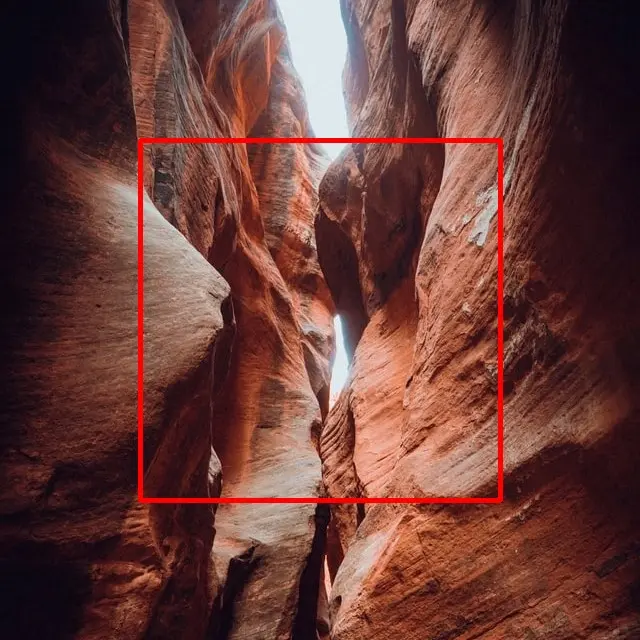
OpenCV 是一個實時計算機視覺庫。它適用於 Python、Java 和 C++ 等程式語言。使用 OpenCV,我們可以高效地繪製形狀和處理影象。在本文中,我們將學習如何使用 OpenCV 模組繪製矩形。
使用 OpenCV 繪製矩形
OpenCV 模組有一個 rectangle()
函式,可用於繪製矩形。它返回一個影象物件。它接受以下引數。
img
:它是必須在其上建立矩形的影象物件。pt1
:它是矩形的起始座標。它接受一個帶有 x 座標值和 y 座標值的元組。pt2
:它是矩形的結束座標。它接受一個帶有 x 座標值和 y 座標值的元組。color
:它是一個表示矩形顏色的元組。OpenCV 預設考慮 BGR 格式。因此,必須以 BGR 格式輸入值,而不是一般的 RGB 格式。例如,(255, 0, 0)
代表藍色,(0, 0, 255)
代表紅色。thickness
:矩形邊框的厚度。它的單位是px
。-1
的厚度將用指定的顏色填充矩形。對於其他正厚度值,矩形將不會填充指定的顏色。它僅適用於邊界。
現在讓我們看看我們如何實際使用這個函式來繪製矩形。示例請參考以下程式碼。
import cv2
image_url = "full\\path\\to\\the\\image\\image.jpg" # Full path is needed
image = cv2.imread(image_url, cv2.COLOR_BGR2RGB)
start_point = (140, 140)
end_point = (500, 500)
color = (0, 0, 255)
thickness = 3
image_with_rectangle = cv2.rectangle(
img=image, pt1=start_point, pt2=end_point, color=color, thickness=thickness
)
cv2.imshow("Image with a Rectangle", image_with_rectangle)
cv2.waitKey(0)
上面的程式碼首先從指定路徑載入影象,使用 cv2.COLOR_BGR2RGB
將 BGR 色序轉換為 RGB 色序,然後在影象上繪製一個大小為 360 x 360
的矩形。注意厚度值為正。這意味著矩形將沒有填充顏色。
此外,我們在程式碼末尾有這個 cv2.waitKey(0)
語句。它可以防止影象在程式執行後自動關閉。如果程式在沒有這條語句的情況下執行,修改後的影象將出現,然後立即關閉。
輸出:
如果我們將厚度值更改為 -1
會怎樣?在這種情況下,矩形將具有填充顏色。相同的參考下面的程式碼。
import cv2
image_url = "full\\path\\to\\the\\image\\image.jpg" # Full path is needed
image = cv2.imread(image_url, cv2.COLOR_BGR2RGB)
start_point = (140, 140)
end_point = (500, 500)
color = (0, 255, 255)
thickness = -1
image_with_rectangle = cv2.rectangle(
img=image, pt1=start_point, pt2=end_point, color=color, thickness=thickness
)
cv2.imshow("Image with a Rectangle", image_with_rectangle)
cv2.waitKey(0)
輸出:
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Vaibhav Vaibhav