計算 Pandas DataFrame 列的數量
Samreena Aslam
2023年1月30日
Pandas
Pandas DataFrame
-
使用
column
屬性計算 PandasDataFrame
的列數 -
使用
shape
屬性計算 PandasDataFrame
的列數 -
使用型別轉換計算 Pandas
DataFrame
的列數 -
使用
dataframe.info()
方法計算 PandasDataFrame
的列數
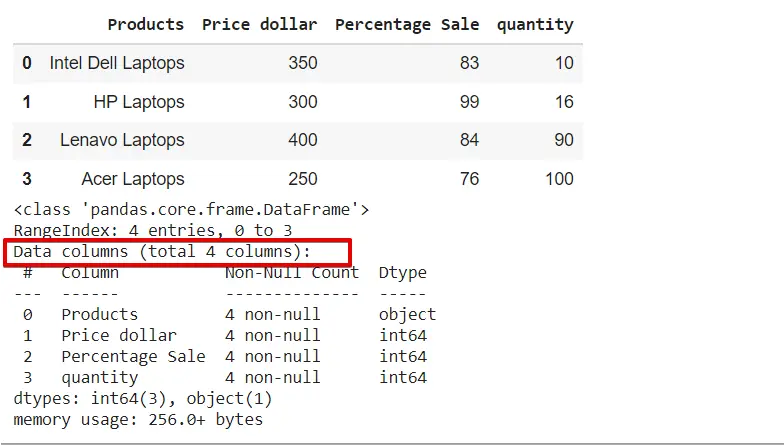
在 Pandas DataFrame
中,資料以表格格式儲存或顯示,如 rows
和 columns
。Pandas 通過使用各種方法幫助我們檢索或計算 DataFrame
中的行數和列數。
我們將在本教程中探索與計算 Pandas DataFrame
的列數相關的各種方法。
使用 column
屬性計算 Pandas DataFrame
的列數
使用 Pandas DataFrame
的 column
屬性,我們可以檢索列列表並計算列長度並計算 DataFrame
中的列數。
請參閱以下示例。首先,我們建立了一個產品的 DataFrame
。使用 column_list = dataframe.columns
,我們檢索列列表,然後使用 len(column_list)
計算列數。
示例程式碼:
import pandas as pd
import numpy as np
from IPython.display import display
# creating a DataFrame
dict = {
"Products": ["Intel Dell Laptops", "HP Laptops", "Lenavo Laptops", "Acer Laptops"],
"Price dollar": [350, 300, 400, 250],
"Percentage Sale": [83, 99, 84, 76],
}
dataframe = pd.DataFrame(dict)
# displaying the DataFrame
display(dataframe)
# To get the list of columns of dataframe
column_list = dataframe.columns
# Printing Number of columns
print("Number of columns:", len(column_list))
輸出:
使用 shape
屬性計算 Pandas DataFrame
的列數
當使用 shape
屬性時,它會檢索表示 DataFrame
形狀的元組。在以下示例中,shape=dataframe.shape
行將返回 DataFrame
形狀,而 shape[1]
計算列數。
示例程式碼:
import pandas as pd
import numpy as np
from IPython.display import display
# creating a DataFrame
dict = {
"Products": ["Intel Dell Laptops", "HP Laptops", "Lenavo Laptops", "Acer Laptops"],
"Price dollar": [350, 300, 400, 250],
"Percentage Sale": [83, 99, 84, 76],
"quantity": [10, 16, 90, 100],
}
dataframe = pd.DataFrame(dict)
# displaying the DataFrame
display(dataframe)
# Get shape of the dataframe
shape = dataframe.shape
# Printing Number of columns
print("Number of columns :", shape[1])
輸出:
正如我們在上面的輸出中看到的那樣,它顯示了上面示例中的 4
的總列數
。
使用型別轉換計算 Pandas DataFrame
的列數
我們在這個方法中使用了型別轉換的方法,它幾乎類似於列屬性。當我們對 DataFrame
列表使用 typecasting
時,它會檢索列名列表。有關型別轉換方法的更多理解,請參見以下示例:
示例程式碼:
import pandas as pd
import numpy as np
from IPython.display import display
# creating a DataFrame
dict = {
"Products": ["Intel Dell Laptops", "HP Laptops", "Lenavo Laptops", "Acer Laptops"],
"Price dollar": [350, 300, 400, 250],
"Percentage Sale": [83, 99, 84, 76],
"quantity": [10, 16, 90, 100],
}
dataframe = pd.DataFrame(dict)
# displaying the DataFrame
display(dataframe)
# Typecasting dataframe to list
dataframe_list = list(dataframe)
# Printing Number of columns
print("Number of columns :", len(dataframe_list))
輸出:
使用 dataframe.info()
方法計算 Pandas DataFrame
的列數
使用 info()
方法,我們可以列印 Pandas DataFrame
的完整簡明摘要。在以下示例中,我們在原始碼末尾使用了 dataframe.info()
。它顯示與 DataFrame
類、dtypes
、記憶體使用情況、列數和範圍索引相關的資訊。
示例程式碼:
import pandas as pd
import numpy as np
from IPython.display import display
# creating a DataFrame
dict = {
"Products": ["Intel Dell Laptops", "HP Laptops", "Lenavo Laptops", "Acer Laptops"],
"Price dollar": [350, 300, 400, 250],
"Percentage Sale": [83, 99, 84, 76],
"quantity": [10, 16, 90, 100],
}
dataframe = pd.DataFrame(dict)
# displaying the DataFrame
display(dataframe)
# Print dataframe information using info() method
dataframe.info()
輸出:
在上圖中,我們可以看到 DataFrame
的簡明摘要,包括列數。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe