PHP 使用 PDO 獲取 MySQL 結果
- 設定資料庫
-
在 PHP 中使用
pdostatement.fetchall()
獲取結果 - 通過迭代 PHP 中的 PDO 語句來獲取結果
-
在 PHP 中使用 PDO
fetch()
方法獲取結果 -
PDO
fetch()
方法的預處理結果
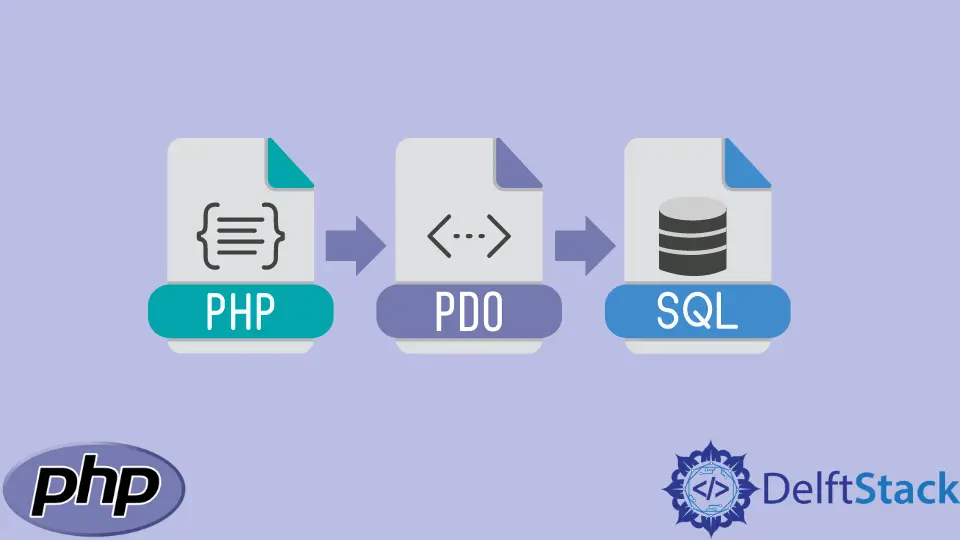
PHP PDO 允許你通過統一的介面使用多個資料庫。它簡化了常規資料庫操作,例如結果獲取。
本教程將解釋如何獲取從 PDO 語句返回的多個結果。你將在 while
迴圈中使用 PDOStatement.fetchAll
、陣列迭代和 fetch()
方法。
設定資料庫
對於本教程,你需要一個 MySQL 資料庫來學習。下載並安裝 XAMPP 伺服器。啟動 XAMPP 控制面板並登入到 MySQL shell。
# This login command assumes that the
# password is empty and the user is "root"
mysql -u root -p
使用以下 SQL 查詢建立一個名為 fruit_db
的資料庫。
CREATE database fruit_db;
輸出:
Query OK, 1 row affected (0.001 sec)
要建立你可以使用的示例資料,請在 fruit_db
資料庫上執行以下 SQL:
CREATE TABLE fruit
(id INT NOT NULL AUTO_INCREMENT,
name VARCHAR(20) NOT NULL,
color VARCHAR(20) NOT NULL,
PRIMARY KEY (id))
ENGINE = InnoDB;
輸出:
Query OK, 0 rows affected (0.028 sec)
使用以下內容確認表存在:
DESC fruit;
輸出:
+-------+-------------+------+-----+---------+----------------+
| Field | Type | Null | Key | Default | Extra |
+-------+-------------+------+-----+---------+----------------+
| id | int(11) | NO | PRI | NULL | auto_increment |
| name | varchar(20) | NO | | NULL | |
| color | varchar(20) | NO | | NULL | |
+-------+-------------+------+-----+---------+----------------+
建立表後,使用以下 SQL 插入示例資料:
INSERT INTO fruit (id, name, color) VALUES (NULL, 'Banana', 'Yellow'), (NULL, 'Pineapple', 'Green')
使用以下 SQL 確認資料存在:
SELECT * FROM fruit;
輸出:
+----+-----------+--------+
| id | name | color |
+----+-----------+--------+
| 1 | Banana | Yellow |
| 2 | Pineapple | Green |
+----+-----------+--------+
現在,你可以從 PHP 中獲取結果。
在 PHP 中使用 pdostatement.fetchall()
獲取結果
在你可以使用 PDOStament.fetchAll()
獲取結果之前,你需要連線到你之前建立的資料庫。建立一個名為 config.php
的檔案,並放置以下程式碼;如果你的資料庫使用者名稱和密碼不同,請替換它們。
<?php
# If necessary, replace the values for the
# user and password variables
$host = 'localhost';
$database = 'fruit_db';
$user = 'root';
$password = '';
?>
當你想在檔案中獲取資料庫結果時,你需要匯入 config.php
。
建立另一個名為 fetchpdo.php
的檔案。在檔案中,你將執行以下操作:
-
連線到資料庫。
-
建立一個新的 PDO 連線。
-
使用
prepare()
方法建立一個準備好的 SQL 語句。 -
執行語句。
-
使用
fetchAll()
方法獲取結果。
現在,在 fetchpdo.php
中鍵入以下程式碼。
<?php
// Require the config file. It contains
// the database connection
require ('config.php');
// Create a connection string
$database_connection = "mysql:host=$host;dbname=$database;charset=UTF8";
// Create a new PDO instance
$pdo = new PDO($database_connection, $user, $password);
// Prepare a SQL statement
$statement = $pdo->prepare('SELECT name, color FROM fruit');
// Execute the statement
$statement->execute();
// Fetch the results
print("Fetch the result set:\n");
$result = $statement->fetchAll(\PDO::FETCH_ASSOC);
print "<pre>";
print_r($result);
print "</pre>";
?>
輸出:
Array
(
[0] => Array
(
[name] => Banana
[color] => Yellow
)
[1] => Array
(
[name] => Pineapple
[color] => Green
)
)
通過迭代 PHP 中的 PDO 語句來獲取結果
執行 SQL 準備語句後,你可以使用 while 迴圈迭代結果。你將在下一個程式碼塊中找到詳細資訊。
<?php
// Require the config file. It contains
// the database connection
require ('config.php');
// Create a connection string
$database_connection = "mysql:host=$host;dbname=$database;charset=UTF8";
// Create a new PDO instance
$pdo = new PDO($database_connection, $user, $password);
// Prepare a SQL statement
$statement = $pdo->prepare('SELECT name, color FROM fruit');
// Execute the statement
$statement->execute(array());
// Iterate over the array
foreach($statement as $row) {
echo $row['name'] . "<br />";
}
?>
輸出:
Banana
Pineapple
在 PHP 中使用 PDO fetch()
方法獲取結果
fetch()
方法將從結果中獲取下一行。它允許你在 while
迴圈中使用它。
詳細資訊在下一個程式碼塊中。
<?php
// Require the config file. It contains
// the database connection
require ('config.php');
// Create a connection string
$database_connection = "mysql:host=$host;dbname=$database;charset=UTF8";
// Create a new PDO instance
$pdo = new PDO($database_connection, $user, $password);
// Prepare a SQL statement
$statement = $pdo->prepare('SELECT name, color FROM fruit');
// Execute the statement
$statement->execute(array());
// Use while loop over the array
while ($row = $statement->fetch()) {
echo $row['name'] . "<br />";
}
?>
PDO fetch()
方法的預處理結果
如果你希望對資料庫資料進行預處理,可以使用 while
迴圈。然後將處理後的結果儲存在一個陣列中。以下程式碼向你展示瞭如何執行此操作。
<?php
// Require the config file. It contains
// the database connection
require ('config.php');
// Create a connection string
$database_connection = "mysql:host=$host;dbname=$database;charset=UTF8";
// Create a new PDO instance
$pdo = new PDO($database_connection, $user, $password);
// Create an empty array to store the results
$result = [];
// Prepare a SQL statement
$statement = $pdo->prepare('SELECT name, color FROM fruit');
// Execute the statement
$statement->execute(array());
// Iterate over the result and assign
// new names to the table rows
while ($row = $statement->fetch()) {
$result[] = [
'Fruit Name' => $row['name'],
'Fruit Color' => $row['color'],
];
}
print "<pre>";
print_r($result);
print "</pre>";
?>
輸出:
Array
(
[0] => Array
(
[Fruit Name] => Banana
[Fruit Color] => Yellow
)
[1] => Array
(
[Fruit Name] => Pineapple
[Fruit Color] => Green
)
)
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn